TaskManager::AbstractTasksModel
#include <abstracttasksmodel.h>
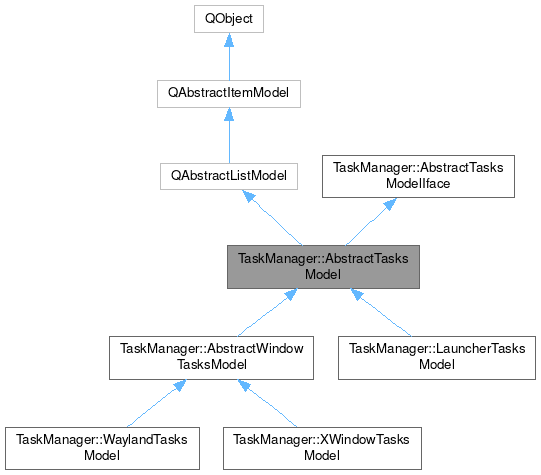
Public Types | |
enum | AdditionalRoles { AppId = Qt::UserRole + 1 , AppName , GenericName , LauncherUrl , LauncherUrlWithoutIcon , WinIdList , MimeType , MimeData , IsWindow , IsStartup , IsLauncher , HasLauncher , IsGroupParent , ChildCount , IsGroupable , IsActive , IsClosable , IsMovable , IsResizable , IsMaximizable , IsMaximized , IsMinimizable , IsMinimized , IsKeepAbove , IsKeepBelow , IsFullScreenable , IsFullScreen , IsShadeable , IsShaded , IsVirtualDesktopsChangeable , VirtualDesktops , IsOnAllVirtualDesktops , Geometry , ScreenGeometry , Activities , IsDemandingAttention , SkipTaskbar , SkipPager , AppPid , StackingOrder , LastActivated , ApplicationMenuServiceName , ApplicationMenuObjectPath , IsHidden , CanLaunchNewInstance } |
![]() | |
enum | CheckIndexOption |
typedef | CheckIndexOptions |
enum | LayoutChangeHint |
![]() | |
typedef | QObjectList |
Public Member Functions | |
AbstractTasksModel (QObject *parent=nullptr) | |
QVariant | data (const QModelIndex &index, int role) const override |
QModelIndex | index (int row, int column=0, const QModelIndex &parent=QModelIndex()) const override |
void | requestActivate (const QModelIndex &index) override |
void | requestActivities (const QModelIndex &index, const QStringList &activities) override |
void | requestClose (const QModelIndex &index) override |
void | requestMove (const QModelIndex &index) override |
void | requestNewInstance (const QModelIndex &index) override |
void | requestNewVirtualDesktop (const QModelIndex &index) override |
void | requestOpenUrls (const QModelIndex &index, const QList< QUrl > &urls) override |
void | requestPublishDelegateGeometry (const QModelIndex &index, const QRect &geometry, QObject *delegate=nullptr) override |
void | requestResize (const QModelIndex &index) override |
void | requestToggleFullScreen (const QModelIndex &index) override |
void | requestToggleKeepAbove (const QModelIndex &index) override |
void | requestToggleKeepBelow (const QModelIndex &index) override |
void | requestToggleMaximized (const QModelIndex &index) override |
void | requestToggleMinimized (const QModelIndex &index) override |
void | requestToggleShaded (const QModelIndex &index) override |
void | requestVirtualDesktops (const QModelIndex &index, const QVariantList &desktops) override |
QHash< int, QByteArray > | roleNames () const override |
![]() | |
QAbstractListModel (QObject *parent) | |
virtual bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) override |
virtual Qt::ItemFlags | flags (const QModelIndex &index) const const override |
virtual QModelIndex | sibling (int row, int column, const QModelIndex &idx) const const override |
![]() | |
QAbstractItemModel (QObject *parent) | |
virtual QModelIndex | buddy (const QModelIndex &index) const const |
virtual bool | canDropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const const |
virtual bool | canFetchMore (const QModelIndex &parent) const const |
bool | checkIndex (const QModelIndex &index, CheckIndexOptions options) const const |
virtual bool | clearItemData (const QModelIndex &index) |
virtual int | columnCount (const QModelIndex &parent) const const=0 |
void | columnsAboutToBeInserted (const QModelIndex &parent, int first, int last) |
void | columnsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | columnsInserted (const QModelIndex &parent, int first, int last) |
void | columnsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsRemoved (const QModelIndex &parent, int first, int last) |
void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight, const QList< int > &roles) |
virtual void | fetchMore (const QModelIndex &parent) |
virtual bool | hasChildren (const QModelIndex &parent) const const |
bool | hasIndex (int row, int column, const QModelIndex &parent) const const |
virtual QVariant | headerData (int section, Qt::Orientation orientation, int role) const const |
void | headerDataChanged (Qt::Orientation orientation, int first, int last) |
bool | insertColumn (int column, const QModelIndex &parent) |
virtual bool | insertColumns (int column, int count, const QModelIndex &parent) |
bool | insertRow (int row, const QModelIndex &parent) |
virtual bool | insertRows (int row, int count, const QModelIndex &parent) |
virtual QMap< int, QVariant > | itemData (const QModelIndex &index) const const |
void | layoutAboutToBeChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
void | layoutChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
virtual QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits, Qt::MatchFlags flags) const const |
virtual QMimeData * | mimeData (const QModelIndexList &indexes) const const |
virtual QStringList | mimeTypes () const const |
void | modelAboutToBeReset () |
void | modelReset () |
bool | moveColumn (const QModelIndex &sourceParent, int sourceColumn, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveColumns (const QModelIndex &sourceParent, int sourceColumn, int count, const QModelIndex &destinationParent, int destinationChild) |
bool | moveRow (const QModelIndex &sourceParent, int sourceRow, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveRows (const QModelIndex &sourceParent, int sourceRow, int count, const QModelIndex &destinationParent, int destinationChild) |
virtual void | multiData (const QModelIndex &index, QModelRoleDataSpan roleDataSpan) const const |
virtual QModelIndex | parent (const QModelIndex &index) const const=0 |
bool | removeColumn (int column, const QModelIndex &parent) |
virtual bool | removeColumns (int column, int count, const QModelIndex &parent) |
bool | removeRow (int row, const QModelIndex &parent) |
virtual bool | removeRows (int row, int count, const QModelIndex &parent) |
virtual void | revert () |
virtual int | rowCount (const QModelIndex &parent) const const=0 |
void | rowsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | rowsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | rowsInserted (const QModelIndex &parent, int first, int last) |
void | rowsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsRemoved (const QModelIndex &parent, int first, int last) |
virtual bool | setData (const QModelIndex &index, const QVariant &value, int role) |
virtual bool | setHeaderData (int section, Qt::Orientation orientation, const QVariant &value, int role) |
virtual bool | setItemData (const QModelIndex &index, const QMap< int, QVariant > &roles) |
virtual void | sort (int column, Qt::SortOrder order) |
virtual QSize | span (const QModelIndex &index) const const |
virtual bool | submit () |
virtual Qt::DropActions | supportedDragActions () const const |
virtual Qt::DropActions | supportedDropActions () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
DoNotUseParent | |
HorizontalSortHint | |
IndexIsValid | |
NoLayoutChangeHint | |
NoOption | |
ParentIsInvalid | |
VerticalSortHint | |
![]() | |
void | beginInsertColumns (const QModelIndex &parent, int first, int last) |
void | beginInsertRows (const QModelIndex &parent, int first, int last) |
bool | beginMoveColumns (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
bool | beginMoveRows (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
void | beginRemoveColumns (const QModelIndex &parent, int first, int last) |
void | beginRemoveRows (const QModelIndex &parent, int first, int last) |
void | beginResetModel () |
void | changePersistentIndex (const QModelIndex &from, const QModelIndex &to) |
void | changePersistentIndexList (const QModelIndexList &from, const QModelIndexList &to) |
QModelIndex | createIndex (int row, int column, const void *ptr) const const |
QModelIndex | createIndex (int row, int column, quintptr id) const const |
void | endInsertColumns () |
void | endInsertRows () |
void | endMoveColumns () |
void | endMoveRows () |
void | endRemoveColumns () |
void | endRemoveRows () |
void | endResetModel () |
QModelIndexList | persistentIndexList () const const |
virtual void | resetInternalData () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
An abstract base class for (flat) tasks models.
This class serves as abstract base class for flat tasks model implementations. It provides data roles and no-op default implementations of methods in the AbstractTasksModelIface interface.
Definition at line 27 of file abstracttasksmodel.h.
Member Enumeration Documentation
◆ AdditionalRoles
Enumerator | |
---|---|
AppId | KService storage id (.desktop name sans extension). |
AppName | Application name. |
GenericName | Generic application name. |
LauncherUrl | URL that can be used to launch this application (.desktop or executable). |
LauncherUrlWithoutIcon | Special path to get a launcher URL while skipping fallback icon encoding. Used as speed optimization. |
WinIdList | NOTE: On Wayland, these ids are only useful within the same process. On X11, they are global window ids. |
MimeType | MIME type for this task (window, window group), needed for DND. |
MimeData | Data for MimeType. |
IsWindow | This is a window task. |
IsStartup | This is a startup task. |
IsLauncher | This is a launcher task. |
HasLauncher | A launcher exists for this task. Only implemented by TasksModel, not by either the single-type or munging tasks models. |
IsGroupParent | This is a parent item for a group of child tasks. |
ChildCount | The number of tasks in this group. |
IsGroupable | Whether this task is being ignored by grouping or not. |
IsActive | This is the currently active task. |
IsClosable | requestClose (see below) available. |
IsMovable | requestMove (see below) available. |
IsResizable | requestResize (see below) available. |
IsMaximizable | requestToggleMaximize (see below) available. |
IsMaximized | Task (i.e. window) is maximized. |
IsMinimizable | requestToggleMinimize (see below) available. |
IsMinimized | Task (i.e. window) is minimized. |
IsKeepAbove | Task (i.e. window) is keep-above. |
IsKeepBelow | Task (i.e. window) is keep-below. |
IsFullScreenable | requestToggleFullScreen (see below) available. |
IsFullScreen | Task (i.e. window) is fullscreen. |
IsShadeable | requestToggleShade (see below) available. |
IsShaded | Task (i.e. window) is shaded. |
IsVirtualDesktopsChangeable | requestVirtualDesktop (see below) available. |
VirtualDesktops | Virtual desktops for the task (i.e. window). |
IsOnAllVirtualDesktops | Task is on all virtual desktops. |
Geometry | The task's geometry (i.e. the window's). |
ScreenGeometry | Screen geometry for the task (i.e. the window's screen). |
Activities | Activities for the task (i.e. window). |
IsDemandingAttention | Task is demanding attention. |
SkipTaskbar | Task should not be shown in a 'task bar' user interface. |
SkipPager | Task should not to be shown in a 'pager' user interface. |
AppPid | Application Process ID. This is provided best-effort, and may not be what you expect: For window tasks owned by processes started from e.g. kwin_wayland, it would be the process id of kwin itself. DO NOT use this for destructive actions such as closing the application. The intended use case is to try and (smartly) gather more information about the task when needed. |
StackingOrder | A window task's index in the window stacking order. Care must be taken not to assume this index to be unique when iterating over model contents due to the asynchronous nature of the windowing system. |
LastActivated | The timestamp of the last time a task was the active task. |
ApplicationMenuServiceName | The DBus service name for the application's menu. May be empty.
|
ApplicationMenuObjectPath | The DBus object path for the application's menu. May be empty.
|
IsHidden | Task (i.e window) is hidden on screen. A minimzed window is not necessarily hidden. |
CanLaunchNewInstance | A new instance of the task can be launched.
|
Definition at line 39 of file abstracttasksmodel.h.
Constructor & Destructor Documentation
◆ AbstractTasksModel()
|
explicit |
Definition at line 13 of file abstracttasksmodel.cpp.
◆ ~AbstractTasksModel()
|
override |
Definition at line 18 of file abstracttasksmodel.cpp.
Member Function Documentation
◆ data()
|
overridevirtual |
Implements QAbstractItemModel.
Definition at line 35 of file abstracttasksmodel.cpp.
◆ index()
|
overridevirtual |
Reimplemented from QAbstractListModel.
Definition at line 111 of file abstracttasksmodel.cpp.
◆ requestActivate()
|
overridevirtual |
Request activation of the task at the given index.
Derived classes are free to interpret the meaning of "activate" themselves depending on the nature and state of the task, e.g. launch or raise a window task.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::LauncherTasksModel, TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 116 of file abstracttasksmodel.cpp.
◆ requestActivities()
|
overridevirtual |
Request moving the task at the given index to the specified activities.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model. activities The new list of activities.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 188 of file abstracttasksmodel.cpp.
◆ requestClose()
|
overridevirtual |
Request the task at the given index be closed.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 132 of file abstracttasksmodel.cpp.
◆ requestMove()
|
overridevirtual |
Request starting an interactive move for the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 137 of file abstracttasksmodel.cpp.
◆ requestNewInstance()
|
overridevirtual |
Request an additional instance of the application backing the task at the given index.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::LauncherTasksModel, TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 121 of file abstracttasksmodel.cpp.
◆ requestNewVirtualDesktop()
|
overridevirtual |
Request entering the window at the given index on a new virtual desktop, which is created in response to this request.
- Parameters
-
index An index in this window tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 183 of file abstracttasksmodel.cpp.
◆ requestOpenUrls()
|
overridevirtual |
Requests to open the given URLs with the application backing the task at the given index.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model. urls The URLs to be passed to the application.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::LauncherTasksModel, TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 126 of file abstracttasksmodel.cpp.
◆ requestPublishDelegateGeometry()
|
overridevirtual |
Request informing the window manager of new geometry for a visual delegate for the task at the given index.
The geometry should be in screen coordinates.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model. geometry Visual delegate geometry in screen coordinates. delegate The delegate. Implementations are on their own with regard to extracting information from this, and should take care to reject invalid objects.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 194 of file abstracttasksmodel.cpp.
◆ requestResize()
|
overridevirtual |
Request starting an interactive resize for the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 142 of file abstracttasksmodel.cpp.
◆ requestToggleFullScreen()
|
overridevirtual |
Request toggling the fullscreen state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 167 of file abstracttasksmodel.cpp.
◆ requestToggleKeepAbove()
|
overridevirtual |
Request toggling the keep-above state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 157 of file abstracttasksmodel.cpp.
◆ requestToggleKeepBelow()
|
overridevirtual |
Request toggling the keep-below state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 162 of file abstracttasksmodel.cpp.
◆ requestToggleMaximized()
|
overridevirtual |
Request toggling the maximized state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 152 of file abstracttasksmodel.cpp.
◆ requestToggleMinimized()
|
overridevirtual |
Request toggling the minimized state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 147 of file abstracttasksmodel.cpp.
◆ requestToggleShaded()
|
overridevirtual |
Request toggling the shaded state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 172 of file abstracttasksmodel.cpp.
◆ requestVirtualDesktops()
|
overridevirtual |
Request entering the window at the given index on the specified virtual desktops, leaving any other desktops.
On Wayland, virtual desktop ids are QStrings. On X11, they are uint >0.
An empty list has a special meaning: The window is entered on all virtual desktops in the session.
On X11, a window can only be on one or all virtual desktops. Therefore, only the first list entry is actually used.
On X11, the id 0 has a special meaning: The window is entered on all virtual desktops in the session.
- Parameters
-
index An index in this window tasks model. desktops A list of virtual desktop ids.
Implements TaskManager::AbstractTasksModelIface.
Reimplemented in TaskManager::WaylandTasksModel, and TaskManager::XWindowTasksModel.
Definition at line 177 of file abstracttasksmodel.cpp.
◆ roleNames()
|
overridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 22 of file abstracttasksmodel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:58:38 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.