KService
#include <KService>
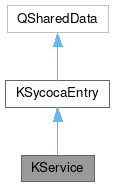
Public Types | |
typedef QList< Ptr > | List |
typedef QExplicitlySharedDataPointer< KService > | Ptr |
![]() | |
typedef QList< Ptr > | List |
typedef QExplicitlySharedDataPointer< KSycocaEntry > | Ptr |
Static Public Member Functions | |
static List | allServices () |
static QString | newServicePath (bool showInMenu, const QString &suggestedName, QString *menuId=nullptr, const QStringList *reservedMenuIds=nullptr) |
static Ptr | serviceByDesktopName (const QString &_name) |
static Ptr | serviceByDesktopPath (const QString &_path) |
static Ptr | serviceByMenuId (const QString &_menuId) |
static Ptr | serviceByStorageId (const QString &_storageId) |
Additional Inherited Members | |
![]() | |
KSERVICE_NO_EXPORT | KSycocaEntry (KSycocaEntryPrivate &d) |
![]() | |
std::unique_ptr< KSycocaEntryPrivate > const | d_ptr |
![]() | |
enum | KSycocaType |
Detailed Description
Represents an installed application.
To obtain a KService instance for a specific application you typically use serviceByDesktopName(), e.g.:
Other typical usage would be in combination with KApplicationTrader to obtain e.g. the default application for a given file type.
- See also
- Desktop Entry Specification
Definition at line 43 of file kservice.h.
Member Typedef Documentation
◆ List
A list of shared data pointers for KService.
Definition at line 53 of file kservice.h.
◆ Ptr
A shared data pointer for KService.
Definition at line 49 of file kservice.h.
Constructor & Destructor Documentation
◆ KService() [1/4]
Construct a temporary service with a given name, exec-line and icon.
- Parameters
-
name the name of the service exec the executable icon the name of the icon
Definition at line 262 of file kservice.cpp.
◆ KService() [2/4]
|
explicit |
Construct a service and take all information from a .desktop file.
- Parameters
-
fullpath Full path to the .desktop file.
Definition at line 274 of file kservice.cpp.
◆ KService() [3/4]
|
explicit |
Construct a service and take all information from a desktop file.
- Parameters
-
config the desktop file to read optional relative path to store for findByName
Definition at line 283 of file kservice.cpp.
◆ KService() [4/4]
KService::KService | ( | const KService & | other | ) |
Definition at line 301 of file kservice.cpp.
◆ ~KService()
|
override |
Definition at line 306 of file kservice.cpp.
Member Function Documentation
◆ actions()
QList< KServiceAction > KService::actions | ( | ) | const |
Returns the actions defined in this desktop file.
Definition at line 806 of file kservice.cpp.
◆ aliasFor()
QString KService::aliasFor | ( | ) | const |
A desktop file name that this application is an alias for.
This is used when a NoDisplay
application is used to enforce specific handling for an application. In that case the NoDisplay
application is an AliasFor
another application and be considered roughly equal to the AliasFor
application (which should not be NoDisplay=true
) For example Okular supplies a desktop file for each supported format (e.g. PDF), all of which NoDisplay
and it is merely there to selectively support specific file formats. A UI may choose to display the aliased entry org.kde.okular instead of the NoDisplay entries.
- Since
- 5.96
- Returns
- QString desktopName of the aliased application (excluding .desktop suffix)
Definition at line 812 of file kservice.cpp.
◆ allowMultipleFiles()
bool KService::allowMultipleFiles | ( | ) | const |
Checks whether this application can handle several files as startup arguments.
- Returns
- true if multiple files may be passed to this service at startup. False if only one file at a time may be passed.
Definition at line 572 of file kservice.cpp.
◆ allServices()
|
static |
Returns the whole list of applications.
Useful for being able to to display them in a list box, for example. More memory consuming than the ones above, don't use unless really necessary.
- Returns
- the list of all applications
Definition at line 409 of file kservice.cpp.
◆ categories()
QStringList KService::categories | ( | ) | const |
Returns a list of VFolder categories.
- Returns
- the list of VFolder categories
Definition at line 582 of file kservice.cpp.
◆ comment()
QString KService::comment | ( | ) | const |
Returns the descriptive comment for the application, if there is one.
- Returns
- the descriptive comment for the application, or QString() if not set
Definition at line 708 of file kservice.cpp.
◆ desktopEntryName()
QString KService::desktopEntryName | ( | ) | const |
Returns the filename of the desktop entry without any extension, e.g.
"org.kde.kate"
- Returns
- the name of the desktop entry without path or extension, or QString() if not set
Definition at line 696 of file kservice.cpp.
◆ docPath()
QString KService::docPath | ( | ) | const |
The path to the documentation for this application.
- Since
- 4.2
- Returns
- the documentation path, or QString() if not set
Definition at line 553 of file kservice.cpp.
◆ exec()
QString KService::exec | ( | ) | const |
Returns the executable.
- Returns
- the command that the service executes, or QString() if not set
Definition at line 661 of file kservice.cpp.
◆ genericName()
QString KService::genericName | ( | ) | const |
Returns the generic name for the application, if there is one (e.g.
"Mail Client").
- Returns
- the generic name, or QString() if not set
Definition at line 714 of file kservice.cpp.
◆ hasMimeType()
bool KService::hasMimeType | ( | const QString & | mimeType | ) | const |
Checks whether the application supports this MIME type.
- Parameters
-
mimeType The name of the MIME type you are interested in determining whether this service supports.
- Since
- 4.6
Definition at line 310 of file kservice.cpp.
◆ icon()
QString KService::icon | ( | ) | const |
Returns the name of the icon.
- Returns
- the icon associated with the service, or QString() if not set
Definition at line 667 of file kservice.cpp.
◆ isApplication()
bool KService::isApplication | ( | ) | const |
Whether this service is an application.
- Returns
- true if this service is an application, i.e. it has Type=Application in its .desktop file and exec() will not be empty.
Definition at line 655 of file kservice.cpp.
◆ keywords()
QStringList KService::keywords | ( | ) | const |
Returns a list of descriptive keywords for the application, if there are any.
- Returns
- the list of keywords
Definition at line 720 of file kservice.cpp.
◆ locateLocal()
QString KService::locateLocal | ( | ) | const |
Returns a path that can be used for saving changes to this application.
- Returns
- path that can be used for saving changes to this application
Definition at line 607 of file kservice.cpp.
◆ menuId()
QString KService::menuId | ( | ) | const |
Returns the menu ID of the application desktop entry.
The menu ID is used to add or remove the entry to a menu.
- Returns
- the menu ID
Definition at line 588 of file kservice.cpp.
◆ mimeTypes()
QStringList KService::mimeTypes | ( | ) | const |
Returns the list of MIME types that this application supports.
Note that this doesn't include inherited MIME types, only the MIME types listed in the .desktop file.
- Since
- 4.8.3
Definition at line 726 of file kservice.cpp.
◆ newServicePath()
|
static |
Returns a path that can be used to create a new KService based on suggestedName
.
- Parameters
-
showInMenu true
, if the application should be shown in the KDE menufalse
, if the application should be hidden from the menu This argument isn't used anymore, useNoDisplay=true
to hide the application.suggestedName name to base the file on, if an application with such a name already exists, a suffix will be added to make it unique (e.g. foo.desktop, foo-1.desktop, foo-2.desktop). menuId If provided, menuId will be set to the menu id to use for the KService reservedMenuIds If provided, the path and menu id will be chosen in such a way that the new menu id does not conflict with any of the reservedMenuIds
- Returns
- The path to use for the new KService.
Definition at line 619 of file kservice.cpp.
◆ noDisplay()
bool KService::noDisplay | ( | ) | const |
Whether the entry should be hidden from the menu.
- Returns
true
to hide this application from the menu
Such services still appear in trader queries, i.e. in "Open With" popup menus for instance.
Definition at line 525 of file kservice.cpp.
◆ property() [1/2]
Definition at line 340 of file kservice.cpp.
◆ property() [2/2]
|
inline |
Returns the requested property.
- Template Parameters
-
T the type of the requested property.
- Parameters
-
name the name of the property.
- Since
- 6.0
Definition at line 321 of file kservice.h.
◆ runOnDiscreteGpu()
bool KService::runOnDiscreteGpu | ( | ) | const |
Returns true
if the application indicates that it's preferred to run on a discrete graphics card, otherwise return false
.
In releases older than 5.86, this method checked for the X-KDE-RunOnDiscreteGpu
key in the .desktop file represented by this service; starting from 5.86 this method now also checks for PrefersNonDefaultGPU
key (added to the Freedesktop.org desktop entry spec in version 1.4 of the spec).
- Since
- 5.30
Definition at line 685 of file kservice.cpp.
◆ schemeHandlers()
QStringList KService::schemeHandlers | ( | ) | const |
Returns the list of scheme handlers this application supports.
For example a web browser could return {"http", "https"}.
This is taken from the x-scheme-handler MIME types listed in the .desktop file.
- Since
- 6.0
Definition at line 740 of file kservice.cpp.
◆ serviceByDesktopName()
|
static |
Find an application by the name of its desktop file, not depending on its actual location (as long as it's under the applications or application directories).
For instance "konqbrowser" or "kcookiejar". Note that the ".desktop" extension is implicit.
This is the recommended method (safe even if the user moves stuff) but note that it assumes that no two entries have the same filename.
- Parameters
-
_name the name of the configuration file
- Returns
- a pointer to the requested application or
nullptr
if the application is unknown. Very important: Don't store the result in a KService* !
Definition at line 421 of file kservice.cpp.
◆ serviceByDesktopPath()
|
static |
Find a application based on its path as returned by entryPath().
It's usually better to use serviceByStorageId() instead.
- Parameters
-
_path the path of the configuration file
- Returns
- a pointer to the requested application or
nullptr
if the application is unknown. Very important: Don't store the result in a KService* !
Definition at line 415 of file kservice.cpp.
◆ serviceByMenuId()
|
static |
Find a application by its menu-id.
- Parameters
-
_menuId the menu id of the application
- Returns
- a pointer to the requested application or
nullptr
if the application is unknown. Very important: Don't store the result in a KService* !
Definition at line 427 of file kservice.cpp.
◆ serviceByStorageId()
|
static |
Find a application by its storage-id or desktop-file path.
This function will try very hard to find a matching application.
- Parameters
-
_storageId the storage id or desktop-file path of the application
- Returns
- a pointer to the requested application or
nullptr
if the application is unknown. Very important: Don't store the result in a KService* !
Definition at line 433 of file kservice.cpp.
◆ setExec()
void KService::setExec | ( | const QString & | exec | ) |
Overrides the "Exec=" line of the service.
If exec is not empty, its value will override the one the one set when this application was created.
Please note that entryPath is also cleared so the application will no longer be associated with a specific config file.
- Since
- 4.11
Definition at line 786 of file kservice.cpp.
◆ setMenuId()
void KService::setMenuId | ( | const QString & | menuId | ) |
Set the menu id
Definition at line 594 of file kservice.cpp.
◆ setTerminal()
void KService::setTerminal | ( | bool | b | ) |
Sets whether to use a terminal or not
Definition at line 774 of file kservice.cpp.
◆ setTerminalOptions()
void KService::setTerminalOptions | ( | const QString & | options | ) |
Sets the terminal options to use
Definition at line 780 of file kservice.cpp.
◆ setWorkingDirectory()
void KService::setWorkingDirectory | ( | const QString & | workingDir | ) |
Overrides the "Path=" line of the application.
If workingDir is not empty, its value will override the one set when this application was created.
Please note that entryPath is also cleared so the application will no longer be associated with a specific config file.
- Parameters
-
workingDir
- Since
- 5.79
Definition at line 796 of file kservice.cpp.
◆ showInCurrentDesktop()
bool KService::showInCurrentDesktop | ( | ) | const |
Whether the application should be shown in the current desktop (including in context menus).
- Returns
- true if the application should be shown in the current desktop.
KApplicationTrader honors this and removes such services from its results.
- Since
- 5.0
Definition at line 457 of file kservice.cpp.
◆ showOnCurrentPlatform()
bool KService::showOnCurrentPlatform | ( | ) | const |
Whether the application should be shown on the current platform (e.g.
on xcb or on wayland).
- Returns
true
if the application should be shown on the current platform.
- Since
- 5.0
Definition at line 499 of file kservice.cpp.
◆ startupNotify()
std::optional< bool > KService::startupNotify | ( | ) | const |
Returns the value of StartupNotify for this service.
If the service doesn't define a value nullopt is returned.
See StartupNotify in the Desktop Entry Specification.
- Since
- 6.0
Definition at line 823 of file kservice.cpp.
◆ storageId()
QString KService::storageId | ( | ) | const |
Returns a normalized ID suitable for storing in configuration files.
It will be based on the menu-id when available and otherwise falls back to entryPath()
- Returns
- the storage ID
Definition at line 600 of file kservice.cpp.
◆ substituteUid()
bool KService::substituteUid | ( | ) | const |
Checks whether the application needs to run under a different UID.
- Returns
true
if the application needs to run under a different UID.
- See also
- username()
Definition at line 439 of file kservice.cpp.
◆ supportedProtocols()
QStringList KService::supportedProtocols | ( | ) | const |
Returns the list of protocols this application supports.
This is taken from the x-scheme-handler MIME types listed in the .desktop file as well as the 'X-KDE-Protocols' entry
For example a web browser could return {"http", "https"}.
- Since
- 6.0
Definition at line 756 of file kservice.cpp.
◆ terminal()
bool KService::terminal | ( | ) | const |
Checks whether the application should be run in a terminal.
This corresponds to Terminal=true
in the .desktop file.
- Returns
true
if the application should be run in a terminal.
Definition at line 679 of file kservice.cpp.
◆ terminalOptions()
QString KService::terminalOptions | ( | ) | const |
Returns any options associated with the terminal the application runs in, if it requires a terminal.
The application must be a TTY-oriented program.
- Returns
- the terminal options, or QString() if not set
Definition at line 673 of file kservice.cpp.
◆ untranslatedGenericName()
QString KService::untranslatedGenericName | ( | ) | const |
Returns the untranslated (US English) generic name for the application, if there is one (e.g.
"Mail Client").
- Returns
- the generic name, or QString() if not set
Definition at line 541 of file kservice.cpp.
◆ untranslatedName()
QString KService::untranslatedName | ( | ) | const |
- Returns
- untranslated name for the given service
- Since
- 6.0
Definition at line 547 of file kservice.cpp.
◆ username()
QString KService::username | ( | ) | const |
Returns the user name if the application runs with a different user id.
- Returns
- the username under which the service has to be run, or QString() if not set
- See also
- substituteUid()
Definition at line 444 of file kservice.cpp.
◆ workingDirectory()
QString KService::workingDirectory | ( | ) | const |
- Returns
- the working directory to run the program in, or QString() if not set
- Since
- 5.63
Definition at line 702 of file kservice.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 17:00:21 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.