BluezQt::Manager
#include <BluezQt/Manager>
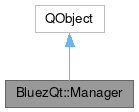
Properties | |
QList< AdapterPtr > | adapters |
bool | bluetoothBlocked |
bool | bluetoothOperational |
QList< DevicePtr > | devices |
bool | initialized |
bool | operational |
BluezQt::Rfkill * | rfkill |
AdapterPtr | usableAdapter |
![]() | |
objectName | |
Signals | |
void | adapterAdded (AdapterPtr adapter) |
void | adapterChanged (AdapterPtr adapter) |
void | adapterRemoved (AdapterPtr adapter) |
void | allAdaptersRemoved () |
void | bluetoothBlockedChanged (bool blocked) |
void | bluetoothOperationalChanged (bool operational) |
void | deviceAdded (DevicePtr device) |
void | deviceChanged (DevicePtr device) |
void | deviceRemoved (DevicePtr device) |
void | operationalChanged (bool operational) |
void | usableAdapterChanged (AdapterPtr adapter) |
Public Member Functions | |
Manager (QObject *parent=nullptr) | |
~Manager () override | |
AdapterPtr | adapterForAddress (const QString &address) const |
AdapterPtr | adapterForUbi (const QString &ubi) const |
QList< AdapterPtr > | adapters () const |
DevicePtr | deviceForAddress (const QString &address) const |
DevicePtr | deviceForUbi (const QString &ubi) const |
QList< DevicePtr > | devices () const |
InitManagerJob * | init () |
bool | isBluetoothBlocked () const |
bool | isBluetoothOperational () const |
bool | isInitialized () const |
bool | isOperational () const |
PendingCall * | registerAgent (Agent *agent) |
PendingCall * | registerProfile (Profile *profile) |
PendingCall * | requestDefaultAgent (Agent *agent) |
Rfkill * | rfkill () const |
void | setBluetoothBlocked (bool blocked) |
PendingCall * | unregisterAgent (Agent *agent) |
PendingCall * | unregisterProfile (Profile *profile) |
AdapterPtr | usableAdapter () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static PendingCall * | startService () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Bluetooth manager.
The entry point to communicate with system BlueZ daemon.
The typical usecase is to work with usableAdapter() (any powered adapter), but it is also possible to use specific adapter.
You must call init() before other functions can be used.
The only functions that can be used before initialization are two rfkill-related functions: isBluetoothBlocked() and setBluetoothBlocked().
Example use in C++ code:
In QML, manager is a singleton and initialization is started when first using the manager. You don't need to track initialized state, just use property binding.
Example use in QML code:
- Note
- All communication with BlueZ daemon happens asynchronously. Almost all methods returns PendingCall to help track the call progress and to check for any errors.
- If manager is not operational, all methods that returns a PendingCall will fail with PendingCall::InternalError.
- See also
- InitManagerJob
Property Documentation
◆ adapters
|
read |
◆ bluetoothBlocked
◆ bluetoothOperational
◆ devices
◆ initialized
◆ operational
◆ rfkill
◆ usableAdapter
|
read |
Constructor & Destructor Documentation
◆ Manager()
|
explicit |
◆ ~Manager()
|
overridedefault |
Destroys a Manager object.
Member Function Documentation
◆ adapterAdded
|
signal |
Indicates that adapter was added.
◆ adapterChanged
|
signal |
Indicates that at least one of the adapter's properties have changed.
◆ adapterForAddress()
AdapterPtr Manager::adapterForAddress | ( | const QString & | address | ) | const |
Returns an adapter for specified address.
- Parameters
-
address address of adapter (eg. "1C:E5:C3:BC:94:7E")
- Returns
- null if there is no adapter with specified address
Definition at line 95 of file manager.cpp.
◆ adapterForUbi()
AdapterPtr Manager::adapterForUbi | ( | const QString & | ubi | ) | const |
Returns an adapter for specified UBI.
- Parameters
-
ubi UBI of adapter (eg. "/org/bluez/hci0")
- Returns
- null if there is no adapter with specified UBI
Definition at line 105 of file manager.cpp.
◆ adapterRemoved
|
signal |
Indicates that adapter was removed.
◆ adapters()
QList< AdapterPtr > Manager::adapters | ( | ) | const |
◆ allAdaptersRemoved
|
signal |
Indicates that all adapters were removed.
◆ bluetoothBlockedChanged
|
signal |
Indicates that Bluetooth blocked state have changed.
◆ bluetoothOperationalChanged
|
signal |
Indicates that Bluetooth operational state have changed.
◆ deviceAdded
|
signal |
Indicates that a new device was added (eg.
found by discovery).
◆ deviceChanged
|
signal |
Indicates that at least one of the device's properties have changed.
◆ deviceForAddress()
Returns a device for specified address.
- Note
- There may be more devices with the same address (same device in multiple adapters). In this case, the first found device will be returned while preferring powered adapters in search.
- Parameters
-
address address of device (eg. "40:79:6A:0C:39:75")
- Returns
- null if there is no device with specified address
Definition at line 110 of file manager.cpp.
◆ deviceForUbi()
Returns a device for specified UBI.
- Parameters
-
ubi UBI of device (eg. "/org/bluez/hci0/dev_40_79_6A_0C_39_75")
- Returns
- null if there is no device with specified UBI
Definition at line 131 of file manager.cpp.
◆ deviceRemoved
|
signal |
Indicates that a device was removed.
◆ devices()
◆ init()
InitManagerJob * Manager::init | ( | ) |
◆ isBluetoothBlocked()
bool Manager::isBluetoothBlocked | ( | ) | const |
Returns whether Bluetooth is blocked.
Bluetooth is blocked if rfkill state for Bluetooth is either SOFT_BLOCKED or HARD_BLOCKED.
- Note
- This requires read access to /dev/rfkill.
- Returns
- true if Bluetooth is blocked
Definition at line 54 of file manager.cpp.
◆ isBluetoothOperational()
bool Manager::isBluetoothOperational | ( | ) | const |
Returns whether Bluetooth is operational.
Bluetooth is operational when manager is operational and there is a valid usable adapter.
- Returns
- true if Bluetooth is operational
Definition at line 49 of file manager.cpp.
◆ isInitialized()
bool Manager::isInitialized | ( | ) | const |
Returns whether the manager is initialized.
- Returns
- true if manager is initialized
Definition at line 39 of file manager.cpp.
◆ isOperational()
bool Manager::isOperational | ( | ) | const |
Returns whether the manager is operational.
The manager is operational when initialization was successful and BlueZ system daemon is running.
- Returns
- true if manager is operational
Definition at line 44 of file manager.cpp.
◆ operationalChanged
|
signal |
Indicates that operational state have changed.
◆ registerAgent()
PendingCall * Manager::registerAgent | ( | Agent * | agent | ) |
Registers agent.
This agent will be used for for all actions triggered by the application. Eg. show a PIN code in pairing process.
Possible errors: PendingCall::InvalidArguments, PendingCall::AlreadyExists
- Parameters
-
agent agent to be registered
- Returns
- void pending call
Definition at line 136 of file manager.cpp.
◆ registerProfile()
PendingCall * Manager::registerProfile | ( | Profile * | profile | ) |
Registers profile.
Possible errors: PendingCall::InvalidArguments, PendingCall::AlreadyExists
- Parameters
-
profile profile to be registered
- Returns
- void pending call
Definition at line 197 of file manager.cpp.
◆ requestDefaultAgent()
PendingCall * Manager::requestDefaultAgent | ( | Agent * | agent | ) |
Requests default agent.
This requests to make the application agent the default agent.
Possible errors: PendingCall::DoesNotExist
- Parameters
-
agent registered agent
- Returns
- void pending call
Definition at line 186 of file manager.cpp.
◆ rfkill()
Rfkill * Manager::rfkill | ( | ) | const |
Definition at line 229 of file manager.cpp.
◆ setBluetoothBlocked()
void Manager::setBluetoothBlocked | ( | bool | blocked | ) |
Sets a Bluetooth blocked state.
This may fail either due to insufficient permissions or because rfkill state is HARD_BLOCKED. In that case, this function returns false.
- Note
- This requires write access to /dev/rfkill.
Definition at line 59 of file manager.cpp.
◆ startService()
|
static |
Attempts to start org.bluez service by D-Bus activation.
Possible return values are 1 if the service was started, 2 if the service is already running or error if the service could not be started.
- Returns
- quint32 pending call
Definition at line 83 of file manager.cpp.
◆ unregisterAgent()
PendingCall * Manager::unregisterAgent | ( | Agent * | agent | ) |
Unregisters agent.
Possible errors: PendingCall::DoesNotExist
- Parameters
-
agent agent to be unregistered
- Returns
- void pending call
Definition at line 173 of file manager.cpp.
◆ unregisterProfile()
PendingCall * Manager::unregisterProfile | ( | Profile * | profile | ) |
Unregisters profile.
Possible errors: PendingCall::DoesNotExist
- Parameters
-
profile profile to be unregistered
- Returns
- void pending call
Definition at line 216 of file manager.cpp.
◆ usableAdapter()
AdapterPtr Manager::usableAdapter | ( | ) | const |
Returns a usable adapter.
Usable adapter is any adapter that is currently powered on.
- Returns
- usable adapter
Definition at line 68 of file manager.cpp.
◆ usableAdapterChanged
|
signal |
Indicates that usable adapter have changed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:48:04 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.