KLineEdit
#include <KLineEdit>
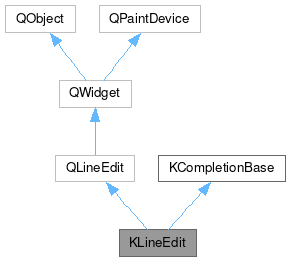
Signals | |
void | aboutToShowContextMenu (QMenu *contextMenu) |
void | clearButtonClicked () |
void | completion (const QString &) |
void | completionBoxActivated (const QString &) |
void | completionModeChanged (KCompletion::CompletionMode) |
void | returnKeyPressed (const QString &text) |
void | substringCompletion (const QString &) |
void | textRotation (KCompletionBase::KeyBindingType) |
Public Slots | |
void | rotateText (KCompletionBase::KeyBindingType type) |
void | setCompletedItems (const QStringList &items, bool autoSuggest=true) override |
void | setCompletedText (const QString &) override |
virtual void | setReadOnly (bool) |
void | setSqueezedText (const QString &text) |
virtual void | setText (const QString &) |
Public Member Functions | |
KLineEdit (const QString &string, QWidget *parent=nullptr) | |
KLineEdit (QWidget *parent=nullptr) | |
~KLineEdit () override | |
QSize | clearButtonUsedSize () const |
virtual KCompletionBox * | completionBox (bool create=true) |
virtual void | copy () const |
void | doCompletion (const QString &text) |
bool | isSqueezedTextEnabled () const |
QString | originalText () const |
void | setCompletionBox (KCompletionBox *box) |
void | setCompletionMode (KCompletion::CompletionMode mode) override |
void | setCompletionModeDisabled (KCompletion::CompletionMode mode, bool disable=true) |
void | setCompletionObject (KCompletion *, bool handle=true) override |
void | setSqueezedTextEnabled (bool enable) |
void | setTrapReturnKey (bool trap) |
void | setUrl (const QUrl &url) |
bool | trapReturnKey () const |
bool | urlDropsEnabled () const |
QString | userText () const |
![]() | |
QLineEdit (const QString &contents, QWidget *parent) | |
QLineEdit (QWidget *parent) | |
QAction * | addAction (const QIcon &icon, ActionPosition position) |
void | addAction (QAction *action, ActionPosition position) |
Qt::Alignment | alignment () const const |
void | backspace () |
void | clear () |
QCompleter * | completer () const const |
void | copy () const const |
QMenu * | createStandardContextMenu () |
void | cursorBackward (bool mark, int steps) |
void | cursorForward (bool mark, int steps) |
Qt::CursorMoveStyle | cursorMoveStyle () const const |
int | cursorPosition () const const |
int | cursorPositionAt (const QPoint &pos) |
void | cursorPositionChanged (int oldPos, int newPos) |
void | cursorWordBackward (bool mark) |
void | cursorWordForward (bool mark) |
void | cut () |
void | del () |
void | deselect () |
QString | displayText () const const |
bool | dragEnabled () const const |
EchoMode | echoMode () const const |
void | editingFinished () |
void | end (bool mark) |
bool | hasAcceptableInput () const const |
bool | hasFrame () const const |
bool | hasSelectedText () const const |
void | home (bool mark) |
QString | inputMask () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery property) const const override |
void | inputRejected () |
void | insert (const QString &newText) |
bool | isClearButtonEnabled () const const |
bool | isModified () const const |
bool | isReadOnly () const const |
bool | isRedoAvailable () const const |
bool | isUndoAvailable () const const |
int | maxLength () const const |
virtual QSize | minimumSizeHint () const const override |
void | paste () |
QString | placeholderText () const const |
void | redo () |
void | returnPressed () |
void | selectAll () |
QString | selectedText () const const |
void | selectionChanged () |
int | selectionEnd () const const |
int | selectionLength () const const |
int | selectionStart () const const |
void | setAlignment (Qt::Alignment flag) |
void | setClearButtonEnabled (bool enable) |
void | setCompleter (QCompleter *c) |
void | setCursorMoveStyle (Qt::CursorMoveStyle style) |
void | setCursorPosition (int) |
void | setDragEnabled (bool b) |
void | setEchoMode (EchoMode) |
void | setFrame (bool) |
void | setInputMask (const QString &inputMask) |
void | setMaxLength (int) |
void | setModified (bool) |
void | setPlaceholderText (const QString &) |
void | setReadOnly (bool) |
void | setSelection (int start, int length) |
void | setText (const QString &) |
void | setTextMargins (const QMargins &margins) |
void | setTextMargins (int left, int top, int right, int bottom) |
void | setValidator (const QValidator *v) |
virtual QSize | sizeHint () const const override |
QString | text () const const |
void | textChanged (const QString &text) |
void | textEdited (const QString &text) |
QMargins | textMargins () const const |
virtual void | timerEvent (QTimerEvent *e) override |
void | undo () |
const QValidator * | validator () const const |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
![]() | |
KCompletionBase () | |
virtual | ~KCompletionBase () |
KCompletion::CompletionMode | completionMode () const |
KCompletion * | completionObject (bool handleSignals=true) |
KCompletion * | compObj () const |
bool | emitSignals () const |
bool | handleSignals () const |
bool | isCompletionObjectAutoDeleted () const |
QList< QKeySequence > | keyBinding (KeyBindingType item) const |
void | setAutoDeleteCompletionObject (bool autoDelete) |
virtual void | setCompletedItems (const QStringList &items, bool autoSuggest=true)=0 |
virtual void | setCompletedText (const QString &text)=0 |
void | setEmitSignals (bool emitRotationSignals) |
void | setEnableSignals (bool enable) |
virtual void | setHandleSignals (bool handle) |
bool | setKeyBinding (KeyBindingType item, const QList< QKeySequence > &key) |
void | useGlobalKeyBindings () |
Protected Slots | |
virtual void | makeCompletion (const QString &) |
void | userCancelled (const QString &cancelText) |
Protected Member Functions | |
bool | autoSuggest () const |
void | contextMenuEvent (QContextMenuEvent *) override |
QMenu * | createStandardContextMenu () |
bool | event (QEvent *) override |
void | keyPressEvent (QKeyEvent *) override |
void | mouseDoubleClickEvent (QMouseEvent *) override |
void | mousePressEvent (QMouseEvent *) override |
void | mouseReleaseEvent (QMouseEvent *) override |
void | paintEvent (QPaintEvent *ev) override |
void | resizeEvent (QResizeEvent *) override |
virtual void | setCompletedText (const QString &, bool) |
void | setUserSelection (bool userSelection) |
![]() | |
virtual void | changeEvent (QEvent *ev) override |
QRect | cursorRect () const const |
virtual void | dragEnterEvent (QDragEnterEvent *e) override |
virtual void | dragLeaveEvent (QDragLeaveEvent *e) override |
virtual void | dragMoveEvent (QDragMoveEvent *e) override |
virtual void | dropEvent (QDropEvent *e) override |
virtual void | focusInEvent (QFocusEvent *e) override |
virtual void | focusOutEvent (QFocusEvent *e) override |
virtual void | initStyleOption (QStyleOptionFrame *option) const const |
virtual void | inputMethodEvent (QInputMethodEvent *e) override |
virtual void | keyReleaseEvent (QKeyEvent *e) override |
virtual void | mouseMoveEvent (QMouseEvent *e) override |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | enterEvent (QEnterEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
![]() | |
KCompletionBase * | delegate () const |
KeyBindingMap | keyBindingMap () const |
void | setDelegate (KCompletionBase *delegate) |
void | setKeyBindingMap (KeyBindingMap keyBindingMap) |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
enum | ActionPosition |
enum | EchoMode |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
![]() | |
typedef QMap< KeyBindingType, QList< QKeySequence > > | KeyBindingMap |
enum | KeyBindingType { TextCompletion , PrevCompletionMatch , NextCompletionMatch , SubstringCompletion } |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
LeadingPosition | |
NoEcho | |
Normal | |
Password | |
PasswordEchoOnEdit | |
TrailingPosition | |
![]() | |
DrawChildren | |
DrawWindowBackground | |
IgnoreMask | |
typedef | RenderFlags |
![]() | |
typedef | QObjectList |
![]() | |
PdmDepth | |
PdmDevicePixelRatio | |
PdmDevicePixelRatioScaled | |
PdmDpiX | |
PdmDpiY | |
PdmHeight | |
PdmHeightMM | |
PdmNumColors | |
PdmPhysicalDpiX | |
PdmPhysicalDpiY | |
PdmWidth | |
PdmWidthMM | |
Detailed Description
An enhanced QLineEdit widget for inputting text.
Detail
This widget inherits from QLineEdit and implements the following additional functionalities:
- a completion object that provides both automatic and manual text completion as well as multiple match iteration features
- configurable key-bindings to activate these features
- a popup-menu item that can be used to allow the user to set text completion modes on the fly based on their preference
To support these features KLineEdit also emits a few more additional signals:
completion(const QString &)
: this signal can be connected to a slot that will assist the user in filling out the remaining texttextRotation(KeyBindingType)
: this signal is intended to be used to iterate through the list of all possible matches whenever there is more than one match for the entered textreturnKeyPressed(const QString &)
: this signal provides the current text in the widget as its argument whenever appropriate (this is in addition to theQLineEdit::returnPressed()
signal which KLineEdit inherits from QLineEdit).
This widget by default creates a completion object when you invoke the completionObject(bool)
member function for the first time or use setCompletionObject(KCompletion *, bool)
to assign your own completion object. Additionally, to make this widget more functional, KLineEdit will by default handle the text rotation and completion events internally when a completion object is created through either one of the methods mentioned above. If you do not need this functionality, simply use KCompletionBase::setHandleSignals(bool)
or set the boolean parameter in the above functions to false.
The default key-bindings for completion and rotation is determined from the global settings in KStandardShortcut. These values, however, can be overridden locally by invoking KCompletionBase::setKeyBinding()
. The values can easily be reverted back to the default setting, by simply calling useGlobalSettings()
. An alternate method would be to default individual key-bindings by using setKeyBinding() with the default second argument.
If EchoMode
for this widget is set to something other than QLineEdit::Normal
, the completion mode will always be defaulted to CompletionNone. This is done purposefully to guard against protected entries, such as passwords, being cached in KCompletion's list. Hence, if the EchoMode
is not QLineEdit::Normal
, the completion mode is automatically disabled.
A read-only KLineEdit will have the same background color as a disabled KLineEdit, but its foreground color will be the one used for the read-write mode. This differs from QLineEdit's implementation and is done to give visual distinction between the three different modes: disabled, read-only, and read-write.
Usage
To enable the basic completion feature:
To use a customized completion object or your own completion object:
Note if you specify your own completion object you have to either delete it when you don't need it anymore, or you can tell KLineEdit to delete it for you:
Miscellaneous function calls :

Definition at line 138 of file klineedit.h.
Property Documentation
◆ squeezedTextEnabled
|
readwrite |
Definition at line 146 of file klineedit.h.
◆ trapEnterKeyEvent
|
readwrite |
Definition at line 145 of file klineedit.h.
Constructor & Destructor Documentation
◆ KLineEdit() [1/2]
Constructs a KLineEdit object with a default text, a parent, and a name.
- Parameters
-
string Text to be shown in the edit widget. parent The parent widget of the line edit.
Definition at line 128 of file klineedit.cpp.
◆ KLineEdit() [2/2]
|
explicit |
Constructs a line edit.
- Parameters
-
parent The parent widget of the line edit.
Definition at line 136 of file klineedit.cpp.
◆ ~KLineEdit()
|
override |
Destructor.
Definition at line 144 of file klineedit.cpp.
Member Function Documentation
◆ aboutToShowContextMenu
|
signal |
Emitted before the context menu is displayed.
The signal allows you to add your own entries into the the context menu that is created on demand.
NOTE: Do not store the pointer to the QMenu provided through since it is created and deleted on demand.
- Parameters
-
contextMenu the context menu about to be displayed
◆ autoSuggest()
|
protected |
Whether in current state text should be auto-suggested.
Definition at line 1366 of file klineedit.cpp.
◆ clearButtonClicked
|
signal |
Emitted when the user clicked on the clear button.
◆ clearButtonUsedSize()
QSize KLineEdit::clearButtonUsedSize | ( | ) | const |
◆ completion
|
signal |
Emitted when the completion key is pressed.
Please note that this signal is not emitted if the completion mode is set to CompletionNone
or EchoMode
is normal.
◆ completionBox()
|
virtual |
This method will create a completion-box if none is there, yet.
- Parameters
-
create Set this to false if you don't want the box to be created i.e. to test if it is available.
- Returns
- the completion-box, that is used in completion mode CompletionPopup.
Definition at line 1274 of file klineedit.cpp.
◆ completionBoxActivated
|
signal |
Emitted whenever the completion box is activated.
◆ completionModeChanged
|
signal |
Emitted when the user changed the completion mode by using the popupmenu.
◆ contextMenuEvent()
|
overrideprotectedvirtual |
Reimplemented for internal reasons.
API not affected.
See QLineEdit::contextMenuEvent().
Reimplemented from QLineEdit.
Definition at line 974 of file klineedit.cpp.
◆ copy()
|
virtual |
Reimplemented for internal reasons, the API is not affected.
Definition at line 416 of file klineedit.cpp.
◆ createStandardContextMenu()
|
protected |
Reimplemented for internal reasons.
API not affected.
See QLineEdit::createStandardContextMenu().
Definition at line 882 of file klineedit.cpp.
◆ doCompletion()
void KLineEdit::doCompletion | ( | const QString & | text | ) |
Do completion now.
This is called automatically when typing a key for instance. Emits completion() and/or calls makeCompletion(), depending on emitSignals and handleSignals.
- Since
- 4.2.1
Definition at line 1399 of file klineedit.cpp.
◆ event()
|
overrideprotectedvirtual |
Reimplemented for internal reasons.
API not affected.
Reimplemented from QLineEdit.
Definition at line 1023 of file klineedit.cpp.
◆ isSqueezedTextEnabled()
bool KLineEdit::isSqueezedTextEnabled | ( | ) | const |
Returns true if text squeezing is enabled.
This is only valid when the widget is in read-only mode.
Definition at line 330 of file klineedit.cpp.
◆ keyPressEvent()
|
overrideprotectedvirtual |
Reimplemented for internal reasons.
API not affected.
See QLineEdit::keyPressEvent().
Reimplemented from QLineEdit.
Definition at line 465 of file klineedit.cpp.
◆ makeCompletion
|
protectedvirtualslot |
Completes the remaining text with a matching one from a given list.
Definition at line 253 of file klineedit.cpp.
◆ mouseDoubleClickEvent()
|
overrideprotectedvirtual |
Reimplemented for internal reasons.
API not affected.
See QWidget::mouseDoubleClickEvent().
Reimplemented from QLineEdit.
Definition at line 830 of file klineedit.cpp.
◆ mousePressEvent()
|
overrideprotectedvirtual |
Reimplemented for internal reasons.
API not affected.
See QLineEdit::mousePressEvent().
Reimplemented from QLineEdit.
Definition at line 842 of file klineedit.cpp.
◆ mouseReleaseEvent()
|
overrideprotectedvirtual |
Reimplemented for internal reasons.
API not affected.
See QLineEdit::mouseReleaseEvent().
Reimplemented from QLineEdit.
Definition at line 864 of file klineedit.cpp.
◆ originalText()
QString KLineEdit::originalText | ( | ) | const |
Returns the original text if text squeezing is enabled.
If the widget is not in "read-only" mode, this function returns the same thing as QLineEdit::text().
- See also
- QLineEdit
Definition at line 1350 of file klineedit.cpp.
◆ paintEvent()
|
overrideprotectedvirtual |
Reimplemented from QLineEdit.
Definition at line 1372 of file klineedit.cpp.
◆ resizeEvent()
|
overrideprotectedvirtual |
Reimplemented for internal reasons.
API not affected.
Reimplemented from QWidget.
Definition at line 455 of file klineedit.cpp.
◆ returnKeyPressed
|
signal |
Emitted when the user presses the Return or Enter key.
The argument is the current text. Note that this signal is not emitted if the widget's EchoMode
is set to QLineEdit::EchoMode.
- Since
- 5.81
◆ rotateText
|
slot |
Iterates through all possible matches of the completed text or the history list.
This function simply iterates over all possible matches in case multiple matches are found as a result of a text completion request. It will have no effect if only a single match is found.
- Parameters
-
type The key-binding invoked.
Definition at line 231 of file klineedit.cpp.
◆ setCompletedItems
|
overrideslot |
Same as the above function except it allows you to temporarily turn off text completion in CompletionPopupAuto mode.
- Parameters
-
items list of completion matches to be shown in the completion box. autoSuggest true if you want automatic text completion (suggestion) enabled.
Definition at line 1216 of file klineedit.cpp.
◆ setCompletedText [1/2]
|
overrideslot |
See KCompletionBase::setCompletedText.
Definition at line 221 of file klineedit.cpp.
◆ setCompletedText() [2/2]
|
protectedvirtual |
This function simply sets the lineedit text and highlights the text appropriately if the boolean value is set to true.
- Parameters
-
text marked
Definition at line 201 of file klineedit.cpp.
◆ setCompletionBox()
void KLineEdit::setCompletionBox | ( | KCompletionBox * | box | ) |
Set the completion-box to be used in completion mode CompletionPopup.
This will do nothing if a completion-box already exists.
- Parameters
-
box The KCompletionBox to set
Definition at line 1076 of file klineedit.cpp.
◆ setCompletionMode()
|
overridevirtual |
Reimplemented from KCompletionBase for internal reasons.
This function is re-implemented in order to make sure that the EchoMode is acceptable before we set the completion mode.
See KCompletionBase::setCompletionMode
Reimplemented from KCompletionBase.
Definition at line 165 of file klineedit.cpp.
◆ setCompletionModeDisabled()
void KLineEdit::setCompletionModeDisabled | ( | KCompletion::CompletionMode | mode, |
bool | disable = true ) |
Disables completion modes by making them non-checkable.
The context menu allows to change the completion mode. This method allows to disable some modes.
Definition at line 195 of file klineedit.cpp.
◆ setCompletionObject()
|
overridevirtual |
Reimplemented for internal reasons, the API is not affected.
Reimplemented from KCompletionBase.
Definition at line 1286 of file klineedit.cpp.
◆ setReadOnly
|
virtualslot |
Sets the lineedit to read-only.
Similar to QLineEdit::setReadOnly but also takes care of the background color, and the clear button.
Definition at line 291 of file klineedit.cpp.
◆ setSqueezedText
|
slot |
Squeezes text
into the line edit.
This can only be used with read-only line-edits.
Definition at line 318 of file klineedit.cpp.
◆ setSqueezedTextEnabled()
void KLineEdit::setSqueezedTextEnabled | ( | bool | enable | ) |
Enable text squeezing whenever the supplied text is too long.
Only works for "read-only" mode.
Note that once text squeezing is enabled, QLineEdit::text() and QLineEdit::displayText() return the squeezed text. If you want the original text, use originalText.
- See also
- QLineEdit
Definition at line 324 of file klineedit.cpp.
◆ setText
|
virtualslot |
Reimplemented to enable text squeezing.
API is not affected.
Definition at line 336 of file klineedit.cpp.
◆ setTrapReturnKey()
void KLineEdit::setTrapReturnKey | ( | bool | trap | ) |
By default, KLineEdit recognizes Key_Return
and Key_Enter
and emits the returnPressed() signals, but it also lets the event pass, for example causing a dialog's default-button to be called.
Call this method with trap
= true
to make KLineEdit
stop these events. The signals will still be emitted of course.
- See also
- trapReturnKey()
Definition at line 1059 of file klineedit.cpp.
◆ setUrl()
void KLineEdit::setUrl | ( | const QUrl & | url | ) |
Sets url
into the lineedit.
It uses QUrl::toDisplayString() so that the url is properly decoded for displaying.
Definition at line 1071 of file klineedit.cpp.
◆ setUserSelection()
|
protected |
Sets the widget in userSelection mode or in automatic completion selection mode.
This changes the colors of selections.
Definition at line 1304 of file klineedit.cpp.
◆ substringCompletion
|
signal |
Emitted when the shortcut for substring completion is pressed.
◆ textRotation
|
signal |
Emitted when the text rotation key-bindings are pressed.
The argument indicates which key-binding was pressed. In KLineEdit's case this can be either one of two values: PrevCompletionMatch or NextCompletionMatch. See KCompletionBase::setKeyBinding for details.
Note that this signal is not emitted if the completion mode is set to CompletionNone
or echoMode()
is not normal.
◆ trapReturnKey()
bool KLineEdit::trapReturnKey | ( | ) | const |
- Returns
true
if keyevents ofKey_Return
orKey_Enter
will be stopped or if they will be propagated.
- See also
- setTrapReturnKey ()
Definition at line 1065 of file klineedit.cpp.
◆ urlDropsEnabled()
bool KLineEdit::urlDropsEnabled | ( | ) | const |
Returns true
when decoded URL drops are enabled.
Definition at line 1053 of file klineedit.cpp.
◆ userCancelled
|
protectedslot |
Resets the current displayed text.
Call this function to revert a text completion if the user cancels the request. Mostly applies to popup completions.
Definition at line 1111 of file klineedit.cpp.
◆ userText()
QString KLineEdit::userText | ( | ) | const |
Returns the text as given by the user (i.e.
not autocompleted) if the widget has autocompletion disabled, this function returns the same as QLineEdit::text().
- Since
- 4.2.2
Definition at line 1360 of file klineedit.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 24 2025 11:58:10 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.