KIO::CommandLauncherJob
#include <KIO/CommandLauncherJob>
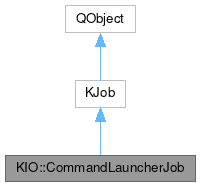
Public Member Functions | |
CommandLauncherJob (const QString &command, QObject *parent=nullptr) | |
CommandLauncherJob (const QString &executable, const QStringList &args, QObject *parent=nullptr) | |
~CommandLauncherJob () override | |
QString | command () const |
qint64 | pid () const |
void | setCommand (const QString &command) |
void | setDesktopName (const QString &desktopName) |
void | setExecutable (const QString &executable) |
void | setProcessEnvironment (const QProcessEnvironment &environment) |
void | setStartupId (const QByteArray &startupId) |
void | setWorkingDirectory (const QString &workingDirectory) |
void | start () override |
QString | workingDirectory () const |
![]() | |
KJob (QObject *parent=nullptr) | |
Capabilities | capabilities () const |
qint64 | elapsedTime () const |
int | error () const |
virtual QString | errorString () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isFinishedNotificationHidden () const |
bool | isStartedWithExec () const |
bool | isSuspended () const |
unsigned long | percent () const |
Q_SCRIPTABLE qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setFinishedNotificationHidden (bool hide=true) |
void | setUiDelegate (KJobUiDelegate *delegate) |
Q_SCRIPTABLE qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef QFlags< Capability > | Capabilities |
enum | Capability |
enum | Unit |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=QPair< QString, QString >(), const QPair< QString, QString > &field2=QPair< QString, QString >()) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &message) |
void | percentChanged (KJob *job, unsigned long percent) |
void | processedAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &message) |
![]() | |
bool | kill (KJob::KillVerbosity verbosity=KJob::Quietly) |
bool | resume () |
bool | suspend () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
Bytes | |
Directories | |
Files | |
Items | |
Killable | |
NoCapabilities | |
Suspendable | |
UnitsCount | |
![]() | |
virtual bool | doKill () |
virtual bool | doResume () |
virtual bool | doSuspend () |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | isFinished () const |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setProgressUnit (Unit unit) |
void | setTotalAmount (Unit unit, qulonglong amount) |
void | startElapsedTimer () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
CommandLauncherJob runs a command and watches it while running.
It creates a startup notification and finishes it on success or on error (for the taskbar). It also emits a "program not found" error message if the requested command did not exist.
The job finishes when the command is successfully started; at that point you can query the PID with pid(). Note that no other errors are handled automatically after the command starts running. As far as CommandLauncherJob is concerned, if the command was launched, the result is a success. If you need to query the command for its exit status or error text later, it is recommended to use QProcess instead.
For error handling, either connect to the result() signal, or for a simple messagebox on error, you can do
- Since
- 5.69
Definition at line 44 of file commandlauncherjob.h.
Constructor & Destructor Documentation
◆ CommandLauncherJob() [1/2]
|
explicit |
Creates a CommandLauncherJob.
- Parameters
-
command the shell command to run The command is given "as is" to the shell, it must already be quoted if necessary. If command
is instead a filename, consider using the other constructor, even if no args are present.parent the parent QObject
Please consider also calling setDesktopName() for better startup notification.
Definition at line 31 of file commandlauncherjob.cpp.
◆ CommandLauncherJob() [2/2]
|
explicit |
Creates a CommandLauncherJob.
- Parameters
-
executable the name of the executable args the commandline arguments to pass to the executable parent the parent QObject
Please consider also calling setDesktopName() for better startup notification.
Definition at line 38 of file commandlauncherjob.cpp.
◆ ~CommandLauncherJob()
|
override |
Destructor.
Note that jobs auto-delete themselves after emitting result
Definition at line 46 of file commandlauncherjob.cpp.
Member Function Documentation
◆ command()
QString KIO::CommandLauncherJob::command | ( | ) | const |
Returns the command executed by this job.
- Since
- 5.83
Definition at line 57 of file commandlauncherjob.cpp.
◆ pid()
qint64 KIO::CommandLauncherJob::pid | ( | ) | const |
- Returns
- the PID of the command that was started
Available after the job emits result().
Definition at line 156 of file commandlauncherjob.cpp.
◆ setCommand()
void KIO::CommandLauncherJob::setCommand | ( | const QString & | command | ) |
Sets the command to execute, this will change the command that was set by any of the constructors.
- Since
- 5.83
Definition at line 52 of file commandlauncherjob.cpp.
◆ setDesktopName()
void KIO::CommandLauncherJob::setDesktopName | ( | const QString & | desktopName | ) |
Set the name of the desktop file (e.g. "org.kde.dolphin", without the ".desktop" filename extension).
This is necessary for startup notification to work.
Definition at line 70 of file commandlauncherjob.cpp.
◆ setExecutable()
void KIO::CommandLauncherJob::setExecutable | ( | const QString & | executable | ) |
Sets the name of the executable, used in the startup notification (see KStartupInfoData::setBin()).
- Parameters
-
executable executable name, with or without a path
Alternatively, use setDesktopName().
Definition at line 65 of file commandlauncherjob.cpp.
◆ setProcessEnvironment()
void KIO::CommandLauncherJob::setProcessEnvironment | ( | const QProcessEnvironment & | environment | ) |
Can be used to pass environment variables to the child process.
- Parameters
-
environment set of environment variables to pass to the child process
- See also
- QProcessEnvironment
- Since
- 5.82
Definition at line 90 of file commandlauncherjob.cpp.
◆ setStartupId()
void KIO::CommandLauncherJob::setStartupId | ( | const QByteArray & | startupId | ) |
Sets the platform-specific startup id of the command launch.
- Parameters
-
startupId startup id, if any (otherwise ""). For X11, this would be the id for the Startup Notification protocol. For Wayland, this would be the token for the XDG Activation protocol.
Definition at line 75 of file commandlauncherjob.cpp.
◆ setWorkingDirectory()
void KIO::CommandLauncherJob::setWorkingDirectory | ( | const QString & | workingDirectory | ) |
Sets the working directory from which to run the command.
- Parameters
-
workingDirectory path of a local directory
Definition at line 80 of file commandlauncherjob.cpp.
◆ start()
|
overridevirtual |
Starts the job.
You must call this, after having called all the necessary setters.
Implements KJob.
Definition at line 95 of file commandlauncherjob.cpp.
◆ workingDirectory()
QString KIO::CommandLauncherJob::workingDirectory | ( | ) | const |
Returns the working directory, which was previously set with setWorkingDirectory()
.
- Since
- 5.83
Definition at line 85 of file commandlauncherjob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:58:45 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.