KIO::MetaData
#include <KIO/MetaData>
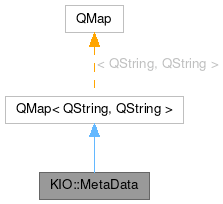
Public Member Functions | |
MetaData () | |
MetaData (const QMap< QString, QString > &metaData) | |
MetaData (const QMap< QString, QVariant > &) | |
MetaData & | operator+= (const QMap< QString, QString > &metaData) |
MetaData & | operator+= (const QMap< QString, QVariant > &metaData) |
MetaData & | operator= (const QMap< QString, QVariant > &metaData) |
QVariant | toVariant () const |
![]() | |
QMap (const QMap< Key, T > &other) | |
QMap (const std::map< Key, T > &other) | |
QMap (QMap< Key, T > &&other) | |
QMap (std::initializer_list< std::pair< Key, T > > list) | |
QMap (std::map< Key, T > &&other) | |
auto | asKeyValueRange () & |
auto | asKeyValueRange () && |
auto | asKeyValueRange () const &&const |
auto | asKeyValueRange () const &const |
iterator | begin () |
const_iterator | begin () const const |
const_iterator | cbegin () const const |
const_iterator | cend () const const |
void | clear () |
const_iterator | constBegin () const const |
const_iterator | constEnd () const const |
const_iterator | constFind (const Key &key) const const |
const_key_value_iterator | constKeyValueBegin () const const |
const_key_value_iterator | constKeyValueEnd () const const |
bool | contains (const Key &key) const const |
size_type | count () const const |
size_type | count (const Key &key) const const |
bool | empty () const const |
iterator | end () |
const_iterator | end () const const |
QPair< iterator, iterator > | equal_range (const Key &key) |
QPair< const_iterator, const_iterator > | equal_range (const Key &key) const const |
iterator | erase (const_iterator first, const_iterator last) |
iterator | erase (const_iterator pos) |
qsizetype | erase_if (QMap< Key, T > &map, Predicate pred) |
iterator | find (const Key &key) |
const_iterator | find (const Key &key) const const |
T & | first () |
const T & | first () const const |
const Key & | firstKey () const const |
iterator | insert (const Key &key, const T &value) |
void | insert (const QMap< Key, T > &map) |
iterator | insert (const_iterator pos, const Key &key, const T &value) |
void | insert (QMap< Key, T > &&map) |
bool | isEmpty () const const |
Key | key (const T &value, const Key &defaultKey) const const |
key_iterator | keyBegin () const const |
key_iterator | keyEnd () const const |
QList< Key > | keys () const const |
QList< Key > | keys (const T &value) const const |
key_value_iterator | keyValueBegin () |
const_key_value_iterator | keyValueBegin () const const |
key_value_iterator | keyValueEnd () |
const_key_value_iterator | keyValueEnd () const const |
T & | last () |
const T & | last () const const |
const Key & | lastKey () const const |
iterator | lowerBound (const Key &key) |
const_iterator | lowerBound (const Key &key) const const |
bool | operator!= (const QMap< Key, T > &lhs, const QMap< Key, T > &rhs) |
QDataStream & | operator<< (QDataStream &out, const QMap< Key, T > &map) |
QMap< Key, T > & | operator= (const QMap< Key, T > &other) |
QMap< Key, T > & | operator= (QMap< Key, T > &&other) |
bool | operator== (const QMap< Key, T > &lhs, const QMap< Key, T > &rhs) |
QDataStream & | operator>> (QDataStream &in, QMap< Key, T > &map) |
T & | operator[] (const Key &key) |
T | operator[] (const Key &key) const const |
size_type | remove (const Key &key) |
size_type | removeIf (Predicate pred) |
size_type | size () const const |
void | swap (QMap< Key, T > &other) |
T | take (const Key &key) |
std::map< Key, T > | toStdMap () && |
std::map< Key, T > | toStdMap () const &const |
iterator | upperBound (const Key &key) |
const_iterator | upperBound (const Key &key) const const |
T | value (const Key &key, const T &defaultValue) const const |
QList< T > | values () const const |
Additional Inherited Members | |
![]() | |
typedef | const_key_value_iterator |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | key_type |
typedef | key_value_iterator |
typedef | mapped_type |
typedef | size_type |
Detailed Description
MetaData is a simple map of key/value strings.
Constructor & Destructor Documentation
◆ MetaData() [1/3]
|
inline |
Creates an empty meta data map.
Definition at line 28 of file metadata.h.
◆ MetaData() [2/3]
Copy constructor.
Definition at line 35 of file metadata.h.
◆ MetaData() [3/3]
Member Function Documentation
◆ operator+=() [1/2]
Adds the given meta data map to this map.
- Parameters
-
metaData the map to add
- Returns
- this map
Definition at line 51 of file metadata.h.
◆ operator+=() [2/2]
|
inline |
Same as above except the value in the map is a QVariant.
This convenience function allows you to easily assign the values of a QVariant to this meta data class.
- Parameters
-
metaData the map to add
- Returns
- this map
- Since
- 4.3.1
Definition at line 94 of file metadata.h.
◆ operator=()
|
inline |
Sets the given meta data map to this map.
- Parameters
-
metaData the map to add
- Returns
- this map
- Since
- 4.3.1
Definition at line 106 of file metadata.h.
◆ toVariant()
|
inline |
Returns the contents of the map as a QVariant.
- Returns
- a QVariant representation of the meta data map.
- Since
- 4.3.1
Definition at line 112 of file metadata.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:58:45 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.