PagePool
#include <pagepool.h>
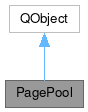
Properties | |
bool | cachePages |
QList< QQuickItem * > | items |
QQuickItem * | lastLoadedItem |
QML_ELEMENTQUrl | lastLoadedUrl |
QList< QUrl > | urls |
![]() | |
objectName | |
Signals | |
void | cachePagesChanged () |
void | itemsChanged () |
void | lastLoadedItemChanged () |
void | lastLoadedUrlChanged () |
void | urlsChanged () |
Public Member Functions | |
PagePool (QObject *parent=nullptr) | |
bool | cachePages () const |
Q_INVOKABLE void | clear () |
Q_INVOKABLE bool | contains (const QVariant &page) const |
Q_INVOKABLE void | deletePage (const QVariant &page) |
Q_INVOKABLE bool | isLocalUrl (const QUrl &url) |
QList< QQuickItem * > | items () const |
QQuickItem * | lastLoadedItem () const |
QUrl | lastLoadedUrl () const |
Q_INVOKABLE QQuickItem * | loadPage (const QString &url, QJSValue callback=QJSValue()) |
Q_INVOKABLE QQuickItem * | loadPageWithProperties (const QString &url, const QVariantMap &properties, QJSValue callback=QJSValue()) |
Q_INVOKABLE QQuickItem * | pageForUrl (const QUrl &url) const |
Q_INVOKABLE QUrl | resolvedUrl (const QString &file) const |
void | setCachePages (bool cache) |
Q_INVOKABLE QUrl | urlForPage (QQuickItem *item) const |
QList< QUrl > | urls () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A Pool of Page items, pages will be unique per url and the items will be kept around unless explicitly deleted.
Instances are C++ owned and can be deleted only manually using deletePage() Instance are unique per url: if you need 2 different instance for a page url, you should instantiate them in the traditional way or use a different PagePool instance.
- See also
- org::kde::kirigami::PagePoolAction
Definition at line 22 of file pagepool.h.
Property Documentation
◆ cachePages
|
readwrite |
If true (default) the pages will be kept around, will have C++ ownership and only one instance per page will be created.
If false the pages will have Javascript ownership (thus deleted on pop by the page stacks) and each call to loadPage will create a new page instance. When cachePages is false, Components get cached nevertheless.
Definition at line 57 of file pagepool.h.
◆ items
|
read |
◆ lastLoadedItem
|
read |
The last item that was loaded with @loadPage.
Definition at line 36 of file pagepool.h.
◆ lastLoadedUrl
|
read |
The last url that was loaded with @loadPage.
Useful if you need to have a "checked" state to buttons or list items that load the page when clicked.
Definition at line 31 of file pagepool.h.
◆ urls
Constructor & Destructor Documentation
◆ PagePool()
PagePool::PagePool | ( | QObject * | parent = nullptr | ) |
Definition at line 17 of file pagepool.cpp.
◆ ~PagePool()
|
override |
Definition at line 22 of file pagepool.cpp.
Member Function Documentation
◆ cachePages()
bool PagePool::cachePages | ( | ) | const |
Definition at line 60 of file pagepool.cpp.
◆ clear()
void PagePool::clear | ( | ) |
Deletes all pages managed by the pool.
Definition at line 274 of file pagepool.cpp.
◆ contains()
bool PagePool::contains | ( | const QVariant & | page | ) | const |
- Returns
- true if the is managed by the PagePool
- Parameters
-
the page can be either a QQuickItem or an url
Definition at line 221 of file pagepool.cpp.
◆ deletePage()
void PagePool::deletePage | ( | const QVariant & | page | ) |
Deletes the page (only if is managed by the pool.
- Parameters
-
page either the url or the instance of the page
Definition at line 235 of file pagepool.cpp.
◆ isLocalUrl()
bool PagePool::isLocalUrl | ( | const QUrl & | url | ) |
- Returns
- true if the url identifies a local resource (local file or a file inside Qt's resource system). False if the url points to a network location
Definition at line 206 of file pagepool.cpp.
◆ items()
QList< QQuickItem * > PagePool::items | ( | ) | const |
Definition at line 36 of file pagepool.cpp.
◆ lastLoadedItem()
QQuickItem * PagePool::lastLoadedItem | ( | ) | const |
Definition at line 31 of file pagepool.cpp.
◆ lastLoadedUrl()
QUrl PagePool::lastLoadedUrl | ( | ) | const |
Definition at line 26 of file pagepool.cpp.
◆ loadPage()
QQuickItem * PagePool::loadPage | ( | const QString & | url, |
QJSValue | callback = QJSValue() ) |
Returns the instance of the item defined in the QML file identified by url, only one instance will be made per url if cachePAges is true.
If the url is remote (i.e. http) don't rely on the return value but us the async callback instead.
- Parameters
-
url full url of the item: it can be a well formed Url, an absolute path or a relative one to the path of the qml file the PagePool is instantiated from callback If we are loading a remote url, we can't have the item immediately but will be passed as a parameter to the provided callback. Normally, don't set a callback, use it only in case of remote urls
- Returns
- the page instance that will have been created if necessary. If the url is remote it will return null, as well will return null if the callback has been provided
Definition at line 65 of file pagepool.cpp.
◆ loadPageWithProperties()
QQuickItem * PagePool::loadPageWithProperties | ( | const QString & | url, |
const QVariantMap & | properties, | ||
QJSValue | callback = QJSValue() ) |
Definition at line 70 of file pagepool.cpp.
◆ pageForUrl()
QQuickItem * PagePool::pageForUrl | ( | const QUrl & | url | ) | const |
- Returns
- The page associated with a given URL, nullptr if there is no correspondence
Definition at line 216 of file pagepool.cpp.
◆ resolvedUrl()
- Returns
- full url from an absolute or relative path
Definition at line 194 of file pagepool.cpp.
◆ setCachePages()
void PagePool::setCachePages | ( | bool | cache | ) |
Definition at line 46 of file pagepool.cpp.
◆ urlForPage()
QUrl PagePool::urlForPage | ( | QQuickItem * | item | ) | const |
- Returns
- The url of the page for the given instance, empty if there is no correspondence
Definition at line 211 of file pagepool.cpp.
◆ urls()
Definition at line 41 of file pagepool.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Oct 11 2024 12:13:25 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.