KTextEditor::AbstractAnnotationItemDelegate
#include <KTextEditor/AbstractAnnotationItemDelegate>
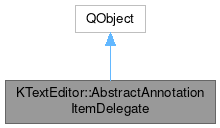
Signals | |
void | sizeHintChanged (KTextEditor::AnnotationModel *model, int line) |
Public Member Functions | |
virtual bool | helpEvent (QHelpEvent *event, KTextEditor::View *view, const KTextEditor::StyleOptionAnnotationItem &option, KTextEditor::AnnotationModel *model, int line)=0 |
virtual void | hideTooltip (KTextEditor::View *view)=0 |
virtual void | paint (QPainter *painter, const KTextEditor::StyleOptionAnnotationItem &option, KTextEditor::AnnotationModel *model, int line) const =0 |
virtual QSize | sizeHint (const KTextEditor::StyleOptionAnnotationItem &option, KTextEditor::AnnotationModel *model, int line) const =0 |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
AbstractAnnotationItemDelegate (QObject *parent=nullptr) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
A delegate for rendering line annotation information and handling events.
Introduction
AbstractAnnotationItemDelegate is a base class that can be reimplemented to customize the rendering of annotation information for each line in a document. It provides also the hooks to define handling of help events like tooltip or of the request for a context menu.
Implementing an AbstractAnnotationItemDelegate
The public interface of this class is loosely based on the QAbstractItemDelegate interfaces. It has five methods to implement.
- Since
- 5.53
- See also
- KTextEditor::AnnotationModel, KTextEditor::AnnotationViewInterface
Definition at line 121 of file abstractannotationitemdelegate.h.
Constructor & Destructor Documentation
◆ AbstractAnnotationItemDelegate()
|
explicitprotected |
Definition at line 431 of file ktexteditor.cpp.
Member Function Documentation
◆ helpEvent()
|
pure virtual |
Whenever a help event occurs, this function is called with the event view option and model
and line
specifying the item where the event occurs.
This pure abstract function must be reimplemented to provide custom tooltips.
- Parameters
-
event the help event view the view for which the help event is requested option the style option object with the info needed for styling, including the rect of the annotation model the annotation model providing the annotation information line index of the real line the annotation information should be painted for
- Returns
true
if the event could be handled (implies that the data obtained from the model had the required role),false
otherwise
Reimplement this in line with hideTooltip().
◆ hideTooltip()
|
pure virtual |
This pure abstract function must be reimplemented to provide custom tooltips.
It is called whenever a possible still shown tooltip no longer is valid, e.g. if the annotations have been hidden.
- Parameters
-
view the view for which the tooltip was requested
Reimplement this in line with helpEvent().
◆ paint()
|
pure virtual |
This pure abstract function must be reimplemented to provide custom rendering.
Use the painter and style option to render the annotation information for the line specified by the arguments model
and line
.
- Parameters
-
painter the painter object option the option object with the info needed for styling model the annotation model providing the annotation information line index of the real line the annotation information should be painted for
Reimplement this in line with sizeHint().
◆ sizeHint()
|
pure virtual |
This pure abstract function must be reimplemented to provide custom rendering.
Use the style option to calculate the best size for the annotation information for the line specified by the arguments model
and line
. This should be the size for the display for a single displayed content line, i.e. with no line wrapping or consecutive multiple annotation item of the same group assumed.
- Note
- If AnnotationViewInterface::uniformAnnotationItemSizes() is
true
for the view this delegate is used by, it is assumed that the returned value is the same for any line.
- Parameters
-
option the option object with the info needed for styling model the annotation model providing the annotation information line index of the real line the annotation information should be painted for
- Returns
- best size for the annotation information
Reimplement this in line with paint().
◆ sizeHintChanged
|
signal |
This signal must be emitted when the sizeHint() for model
and line
changed.
The view automatically connects to this signal and relayouts as necessary. If AnnotationViewInterface::uniformAnnotationItemSizes is set on the view, it is sufficient to emit sizeHintChanged only for one line.
- Parameters
-
model the annotation model providing the annotation information line index of the real line the annotation information should be painted for
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 12:00:13 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.