KTextEditor::Attribute
#include <KTextEditor/Attribute>
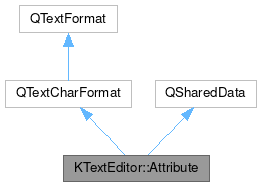
Public Types | |
typedef QExplicitlySharedDataPointer< Attribute > | Ptr |
![]() | |
enum | FontPropertiesInheritanceBehavior |
enum | UnderlineStyle |
enum | VerticalAlignment |
![]() | |
enum | FormatType |
enum | ObjectTypes |
enum | PageBreakFlag |
enum | Property |
Public Member Functions | |
Attribute () | |
Attribute (const Attribute &a) | |
Attribute (const QString &name, KSyntaxHighlighting::Theme::TextStyle style) | |
virtual | ~Attribute () |
Attribute & | operator+= (const Attribute &a) |
Attribute & | operator= (const Attribute &a) |
Custom properties | |
The following functions provide custom properties which can be set for rendering by editor implementations. | |
QString | name () const |
void | setName (const QString &name) |
KSyntaxHighlighting::Theme::TextStyle | defaultStyle () const |
void | setDefaultStyle (KSyntaxHighlighting::Theme::TextStyle style) |
bool | skipSpellChecking () const |
void | setSkipSpellChecking (bool skipspellchecking) |
bool | fontBold () const |
void | setFontBold (bool bold=true) |
QBrush | outline () const |
void | setOutline (const QBrush &brush) |
QBrush | selectedForeground () const |
void | setSelectedForeground (const QBrush &foreground) |
QBrush | selectedBackground () const |
void | setSelectedBackground (const QBrush &brush) |
bool | backgroundFillWhitespace () const |
void | setBackgroundFillWhitespace (bool fillWhitespace) |
void | clear () |
bool | hasAnyProperty () const |
![]() | |
QString | anchorHref () const const |
QStringList | anchorNames () const const |
qreal | baselineOffset () const const |
QFont | font () const const |
QFont::Capitalization | fontCapitalization () const const |
QVariant | fontFamilies () const const |
QString | fontFamily () const const |
bool | fontFixedPitch () const const |
QFont::HintingPreference | fontHintingPreference () const const |
bool | fontItalic () const const |
bool | fontKerning () const const |
qreal | fontLetterSpacing () const const |
QFont::SpacingType | fontLetterSpacingType () const const |
bool | fontOverline () const const |
qreal | fontPointSize () const const |
int | fontStretch () const const |
bool | fontStrikeOut () const const |
QFont::StyleHint | fontStyleHint () const const |
QVariant | fontStyleName () const const |
QFont::StyleStrategy | fontStyleStrategy () const const |
bool | fontUnderline () const const |
int | fontWeight () const const |
qreal | fontWordSpacing () const const |
bool | isAnchor () const const |
bool | isValid () const const |
void | setAnchor (bool anchor) |
void | setAnchorHref (const QString &value) |
void | setAnchorNames (const QStringList &names) |
void | setBaselineOffset (qreal baseline) |
void | setFont (const QFont &font, FontPropertiesInheritanceBehavior behavior) |
void | setFontCapitalization (QFont::Capitalization capitalization) |
void | setFontFamilies (const QStringList &families) |
void | setFontFamily (const QString &family) |
void | setFontFixedPitch (bool fixedPitch) |
void | setFontHintingPreference (QFont::HintingPreference hintingPreference) |
void | setFontItalic (bool italic) |
void | setFontKerning (bool enable) |
void | setFontLetterSpacing (qreal spacing) |
void | setFontLetterSpacingType (QFont::SpacingType letterSpacingType) |
void | setFontOverline (bool overline) |
void | setFontPointSize (qreal size) |
void | setFontStretch (int factor) |
void | setFontStrikeOut (bool strikeOut) |
void | setFontStyleHint (QFont::StyleHint hint, QFont::StyleStrategy strategy) |
void | setFontStyleName (const QString &styleName) |
void | setFontStyleStrategy (QFont::StyleStrategy strategy) |
void | setFontUnderline (bool underline) |
void | setFontWeight (int weight) |
void | setFontWordSpacing (qreal spacing) |
void | setSubScriptBaseline (qreal baseline) |
void | setSuperScriptBaseline (qreal baseline) |
void | setTextOutline (const QPen &pen) |
void | setToolTip (const QString &text) |
void | setUnderlineColor (const QColor &color) |
void | setUnderlineStyle (UnderlineStyle style) |
void | setVerticalAlignment (VerticalAlignment alignment) |
qreal | subScriptBaseline () const const |
qreal | superScriptBaseline () const const |
QPen | textOutline () const const |
QString | toolTip () const const |
QColor | underlineColor () const const |
UnderlineStyle | underlineStyle () const const |
VerticalAlignment | verticalAlignment () const const |
![]() | |
QTextFormat (const QTextFormat &other) | |
QTextFormat (int type) | |
QBrush | background () const const |
bool | boolProperty (int propertyId) const const |
QBrush | brushProperty (int propertyId) const const |
void | clearBackground () |
void | clearForeground () |
void | clearProperty (int propertyId) |
QColor | colorProperty (int propertyId) const const |
qreal | doubleProperty (int propertyId) const const |
QBrush | foreground () const const |
bool | hasProperty (int propertyId) const const |
int | intProperty (int propertyId) const const |
bool | isBlockFormat () const const |
bool | isCharFormat () const const |
bool | isEmpty () const const |
bool | isFrameFormat () const const |
bool | isImageFormat () const const |
bool | isListFormat () const const |
bool | isTableCellFormat () const const |
bool | isTableFormat () const const |
bool | isValid () const const |
Qt::LayoutDirection | layoutDirection () const const |
QTextLength | lengthProperty (int propertyId) const const |
QList< QTextLength > | lengthVectorProperty (int propertyId) const const |
void | merge (const QTextFormat &other) |
int | objectIndex () const const |
int | objectType () const const |
QVariant | operator QVariant () const const |
bool | operator!= (const QTextFormat &other) const const |
QTextFormat & | operator= (const QTextFormat &other) |
bool | operator== (const QTextFormat &other) const const |
QPen | penProperty (int propertyId) const const |
QMap< int, QVariant > | properties () const const |
QVariant | property (int propertyId) const const |
int | propertyCount () const const |
void | setBackground (const QBrush &brush) |
void | setForeground (const QBrush &brush) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setObjectIndex (int index) |
void | setObjectType (int type) |
void | setProperty (int propertyId, const QList< QTextLength > &value) |
void | setProperty (int propertyId, const QVariant &value) |
QString | stringProperty (int propertyId) const const |
void | swap (QTextFormat &other) |
QTextBlockFormat | toBlockFormat () const const |
QTextCharFormat | toCharFormat () const const |
QTextFrameFormat | toFrameFormat () const const |
QTextImageFormat | toImageFormat () const const |
QTextListFormat | toListFormat () const const |
QTextTableCellFormat | toTableCellFormat () const const |
QTextTableFormat | toTableFormat () const const |
int | type () const const |
![]() | |
QSharedData (const QSharedData &) | |
Dynamic highlighting | |
The following functions allow for text to be highlighted dynamically based on several events. | |
enum | ActivationType { ActivateMouseIn = 0 , ActivateCaretIn } |
Attribute::Ptr | dynamicAttribute (ActivationType type) const |
void | setDynamicAttribute (ActivationType type, Attribute::Ptr attribute) |
Detailed Description
A class which provides customized text decorations.
The Attribute class extends QTextCharFormat, the class which Qt uses internally to provide formatting information to characters in a text document.
In addition to its inherited properties, it provides support for:
- several customized text formatting properties
- dynamic highlighting of associated ranges of text
- binding of actions with associated ranges of text (note: not currently implemented)
Implementations are not required to support all properties. In particular, several properties are not supported for dynamic highlighting (notably: font() and fontBold()).
Unfortunately, as QTextFormat's setProperty() is not virtual, changes that are made to this attribute cannot automatically be redrawn. Once you have finished changing properties, you should call changed() to force redrawing of affected ranges of text.
- See also
- MovingInterface
Definition at line 50 of file attribute.h.
Member Typedef Documentation
◆ Ptr
Shared data pointer for Attribute.
Definition at line 56 of file attribute.h.
Member Enumeration Documentation
◆ ActivationType
Several automatic activation mechanisms exist for associated attributes.
Using this you can conveniently have your ranges highlighted when either the mouse or cursor enter the range.
Enumerator | |
---|---|
ActivateMouseIn | Activate attribute on mouse in. |
ActivateCaretIn | Activate attribute on caret in. |
Definition at line 244 of file attribute.h.
Constructor & Destructor Documentation
◆ Attribute() [1/3]
Attribute::Attribute | ( | ) |
Default constructor.
The resulting Attribute has no properties set to begin with.
Definition at line 29 of file attribute.cpp.
◆ Attribute() [2/3]
Attribute::Attribute | ( | const QString & | name, |
KSyntaxHighlighting::Theme::TextStyle | style ) |
Construct attribute with given name & default style properties.
- Parameters
-
name attribute name style attribute default style
Definition at line 34 of file attribute.cpp.
◆ Attribute() [3/3]
Attribute::Attribute | ( | const Attribute & | a | ) |
Copy constructor.
Definition at line 41 of file attribute.cpp.
◆ ~Attribute()
|
virtual |
Virtual destructor.
Definition at line 49 of file attribute.cpp.
Member Function Documentation
◆ backgroundFillWhitespace()
bool Attribute::backgroundFillWhitespace | ( | ) | const |
Determine whether background color is drawn over whitespace.
Defaults to true if not set.
- Returns
- whether the background color should be drawn over whitespace
Definition at line 147 of file attribute.cpp.
◆ clear()
void Attribute::clear | ( | ) |
Clear all set properties.
Definition at line 175 of file attribute.cpp.
◆ defaultStyle()
KSyntaxHighlighting::Theme::TextStyle Attribute::defaultStyle | ( | ) | const |
◆ dynamicAttribute()
Attribute::Ptr Attribute::dynamicAttribute | ( | ActivationType | type | ) | const |
Return the attribute to use when the event referred to by type occurs.
- Parameters
-
type the activation type for which to return the Attribute.
- Returns
- the attribute to be used for events specified by type, or null if none is set.
Definition at line 71 of file attribute.cpp.
◆ fontBold()
bool Attribute::fontBold | ( | ) | const |
Find out if the font weight is set to QFont::Bold.
- Returns
true
if the font weight is exactly QFont::Bold, otherwisefalse
- See also
- QTextCharFormat::fontWeight()
Definition at line 184 of file attribute.cpp.
◆ hasAnyProperty()
bool Attribute::hasAnyProperty | ( | ) | const |
Determine if any properties are set.
- Returns
- true if any properties are set, otherwise false
Definition at line 198 of file attribute.cpp.
◆ name()
QString Attribute::name | ( | ) | const |
◆ operator+=()
Addition assignment operator.
Use this to merge another Attribute with this Attribute. Where both attributes have a particular property set, the property in a will be used.
- Parameters
-
a attribute to merge into this attribute.
Definition at line 54 of file attribute.cpp.
◆ operator=()
Replacement assignment operator.
Use this to overwrite this Attribute with another Attribute.
- Parameters
-
a attribute to assign to this attribute.
Definition at line 203 of file attribute.cpp.
◆ outline()
QBrush Attribute::outline | ( | ) | const |
Get the brush used to draw an outline around text, if any.
- Returns
- brush to be used to draw an outline, or Qt::NoBrush if no outline is set.
Definition at line 119 of file attribute.cpp.
◆ selectedBackground()
QBrush Attribute::selectedBackground | ( | ) | const |
Get the brush used to draw the background of selected text, if any.
- Returns
- brush to be used to draw the background of selected text, or Qt::NoBrush if not set
Definition at line 161 of file attribute.cpp.
◆ selectedForeground()
QBrush Attribute::selectedForeground | ( | ) | const |
Get the brush used to draw text when it is selected, if any.
- Returns
- brush to be used to draw selected text, or Qt::NoBrush if not set
Definition at line 133 of file attribute.cpp.
◆ setBackgroundFillWhitespace()
void Attribute::setBackgroundFillWhitespace | ( | bool | fillWhitespace | ) |
Set whether background color is drawn over whitespace.
Defaults to true if not set.
Use clearProperty(BackgroundFillWhitespace)
to clear.
- Parameters
-
fillWhitespace whether the background should be drawn over whitespace.
Definition at line 156 of file attribute.cpp.
◆ setDefaultStyle()
void Attribute::setDefaultStyle | ( | KSyntaxHighlighting::Theme::TextStyle | style | ) |
Set default style of this attribute.
- Parameters
-
style new default style
Definition at line 104 of file attribute.cpp.
◆ setDynamicAttribute()
void Attribute::setDynamicAttribute | ( | ActivationType | type, |
Attribute::Ptr | attribute ) |
Set the attribute to use when the event referred to by type occurs.
- Note
- Nested dynamic attributes are ignored.
- Parameters
-
type the activation type to set the attribute for attribute the attribute to assign. As attribute is refcounted, ownership is not an issue.
Definition at line 80 of file attribute.cpp.
◆ setFontBold()
void Attribute::setFontBold | ( | bool | bold = true | ) |
Set the font weight to QFont::Bold.
If bold is false
, the weight will be set to 0 (normal).
- Parameters
-
bold whether the font weight should be bold or not.
- See also
- QTextCharFormat::setFontWeight()
Definition at line 189 of file attribute.cpp.
◆ setName()
void Attribute::setName | ( | const QString & | name | ) |
◆ setOutline()
void Attribute::setOutline | ( | const QBrush & | brush | ) |
Set a brush to be used to draw an outline around text.
Use clearProperty(Outline)
to clear.
- Parameters
-
brush brush to be used to draw an outline.
Definition at line 128 of file attribute.cpp.
◆ setSelectedBackground()
void Attribute::setSelectedBackground | ( | const QBrush & | brush | ) |
Set a brush to be used to draw the background of selected text, if any.
Use clearProperty(SelectedBackground)
to clear.
- Parameters
-
brush brush to be used to draw the background of selected text
Definition at line 170 of file attribute.cpp.
◆ setSelectedForeground()
void Attribute::setSelectedForeground | ( | const QBrush & | foreground | ) |
Set a brush to be used to draw selected text.
Use clearProperty(SelectedForeground)
to clear.
- Parameters
-
foreground brush to be used to draw selected text.
Definition at line 142 of file attribute.cpp.
◆ setSkipSpellChecking()
void Attribute::setSkipSpellChecking | ( | bool | skipspellchecking | ) |
Set if we should spellchecking be skipped?
- Parameters
-
skipspellchecking should spellchecking be skipped?
Definition at line 114 of file attribute.cpp.
◆ skipSpellChecking()
bool Attribute::skipSpellChecking | ( | ) | const |
Should spellchecking be skipped?
- Returns
- skip spellchecking?
Definition at line 109 of file attribute.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 12:00:13 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.