KTextEditor::InlineNoteProvider
#include <KTextEditor/InlineNoteProvider>
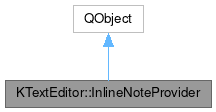
Signals | |
void | inlineNotesChanged (int line) |
void | inlineNotesReset () |
Public Member Functions | |
InlineNoteProvider () | |
~InlineNoteProvider () override | |
virtual void | inlineNoteActivated (const InlineNote ¬e, Qt::MouseButtons buttons, const QPoint &globalPos) |
virtual void | inlineNoteFocusInEvent (const InlineNote ¬e, const QPoint &globalPos) |
virtual void | inlineNoteFocusOutEvent (const InlineNote ¬e) |
virtual void | inlineNoteMouseMoveEvent (const InlineNote ¬e, const QPoint &globalPos) |
virtual QList< int > | inlineNotes (int line) const =0 |
virtual QSize | inlineNoteSize (const InlineNote ¬e) const =0 |
virtual void | paintInlineNote (const InlineNote ¬e, QPainter &painter, Qt::LayoutDirection direction) const =0 |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A source of inline notes for a document.
InlineNoteProvider is a object that can be queried for inline notes in the view. It emits signals when the notes change and should be queried again.
- See also
- KTextEditor::View
- Since
- 5.50
Definition at line 28 of file inlinenoteprovider.h.
Constructor & Destructor Documentation
◆ InlineNoteProvider()
|
default |
Default constructor.
◆ ~InlineNoteProvider()
|
overridedefault |
Virtual destructor to allow inheritance.
Member Function Documentation
◆ inlineNoteActivated()
|
virtual |
Invoked when a note is activated by the user.
This method is called when a user activates a note, i.e. clicks on it. Coordinates of pos
are in note coordinates, i.e. relative to the note's top-left corner (same coordinate system as the painter has in paintInlineNote()).
The default implementation does nothing.
- Parameters
-
note the note which was activated buttons the button(s) the note was clicked with globalPos the point the note was clicked at in global screen coordinates
Definition at line 293 of file ktexteditor.cpp.
◆ inlineNoteFocusInEvent()
|
virtual |
Invoked when the mouse cursor moves into the note
when it was outside before.
The default implementation does nothing.
- Parameters
-
note the note which was activated globalPos the location of the mouse cursor in global screen coordinates
Definition at line 300 of file ktexteditor.cpp.
◆ inlineNoteFocusOutEvent()
|
virtual |
Invoked when the mouse cursor leaves the note.
The default implementation does nothing.
- Parameters
-
note the note which was deactivated
Definition at line 306 of file ktexteditor.cpp.
◆ inlineNoteMouseMoveEvent()
|
virtual |
Invoked when the mouse cursor moves inside the note.
The default implementation does nothing.
- Parameters
-
note the note which was hovered globalPos the location of the mouse cursor in global screen coordinates
Definition at line 311 of file ktexteditor.cpp.
◆ inlineNotes()
|
pure virtual |
Get list of inline notes for given line.
Should return a vector of columns on which the notes are located. 0 means the note is located before the first character of the line. 1 means the note is located after the first character, etc. If the returned number is greater than the length of the line, the note will be placed behind the text as if there were additional spaces.
- Note
- When returning multiple InlineNotes, use InlineNote::index() to map the InlineNote to this QList's index.
- Parameters
-
line Line number
- Returns
- vector of columns where inline notes appear in this line
◆ inlineNotesChanged
|
signal |
The provider should emit the signal inlineNotesChanged() whenever one or more InlineNotes on the line changed.
◆ inlineNoteSize()
|
pure virtual |
Width to be reserved for the note in the text.
Typically, a custom width with the current line height can be returned. If the width depends on the font size, note.font() can be used to obtain the font metrics.
Example to reserve a square size for painting:
- Note
- Do not return heights that are larger than note.lineHeight(), since the painting code clips to the line height anyways.
- Parameters
-
note the InlineNote for which the size is queried
- Returns
- the required size of the InlineNote
◆ inlineNotesReset
|
signal |
The provider should emit the signal inlineNotesReset() when almost all inline notes changed.
◆ paintInlineNote()
|
pure virtual |
Paint the note into the line.
The method should use the given painter to render the note into the line. The painter is translated such that coordinates 0x0 mark the top left corner of the note. The method should not paint outside rectangle given by the size previously returned by inlineNoteSize().
The method is given the height of the line, the metrics of current font and the font which it may use during painting.
If wanted, you can use note.underMouse() to e.g. highlight the
- Parameters
-
note note to paint, containing location and index painter painter prepared for rendering the note direction direction of the line i.e., right to left/left to right
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 17 2024 11:56:22 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.