KTextTemplate::Engine
#include <KTextTemplate/Engine>
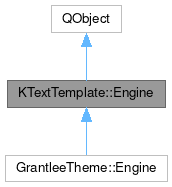
Public Member Functions | |
Engine (QObject *parent={}) | |
~Engine () override | |
void | addDefaultLibrary (const QString &libName) |
void | addPluginPath (const QString &dir) |
void | addTemplateLoader (QSharedPointer< AbstractTemplateLoader > loader) |
QStringList | defaultLibraries () const |
Template | loadByName (const QString &name) const |
std::pair< QString, QString > | mediaUri (const QString &fileName) const |
Template | newTemplate (const QString &content, const QString &name) const |
QStringList | pluginPaths () const |
void | removeDefaultLibrary (const QString &libName) |
void | removePluginPath (const QString &dir) |
void | setPluginPaths (const QStringList &dirs) |
void | setSmartTrimEnabled (bool enabled) |
bool | smartTrimEnabled () const |
QList< QSharedPointer< AbstractTemplateLoader > > | templateLoaders () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
KTextTemplate::Engine is the main entry point for creating KTextTemplate Templates.
The KTextTemplate::Engine is responsible for configuring and creating Template objects. In typical use, one or more AbstractTemplateLoader objects will be added to the Engine to load template objects, and plugin directories will be set to enable finding template tags and filters.
Once it is configured, the Engine can be used to create new templates by name by loading the templates with the loadByName method, or by defining the content in the newTemplate method.
By default the builtin tags and filters distributed with KTextTemplate are available in all templates without using the {% load %}
tag in the template. These pre-loaded libraries may be configured if appropriate to the application. For example, an application which defines its own tags and filters may want them to be always available, or it may be desirable to restrict the features available to template authors by removing built in libraries.
Different Engine objects can be used to create templates with differing configurations.
Insignificant whitespace
The output of rendering a template depends on the content of the template. In some cases when generating content in which whitespace is significant, this can have undesired effects. For example, given a template to generate C++ code like:
class MyClass { {# This loop creates the #} {# methods in the class #} {% for method in methods %} {% if method.hasDox %} {{ method.dox }} {% endif %} {{ method.signature }} {% endfor %} };
The output would have a lot of whitespace which is not necessarily wanted.
It is possible to strip insignificant whitespace by enabling the smartTrim feature with setSmartTrimEnabled. When enabled the output will not contain a newline for any line in the template which has only one token of template syntax, such as a comment, tag or variable.
Constructor & Destructor Documentation
◆ Engine()
Engine::Engine | ( | QObject * | parent = {} | ) |
Constructor.
Definition at line 29 of file engine.cpp.
◆ ~Engine()
|
override |
Destructor.
Definition at line 40 of file engine.cpp.
Member Function Documentation
◆ addDefaultLibrary()
void Engine::addDefaultLibrary | ( | const QString & | libName | ) |
Adds the library named libName
to the libraries available by default to new Templates.
Definition at line 107 of file engine.cpp.
◆ addPluginPath()
void Engine::addPluginPath | ( | const QString & | dir | ) |
Prepend dir
to the list of plugin dirs.
Definition at line 80 of file engine.cpp.
◆ addTemplateLoader()
void Engine::addTemplateLoader | ( | QSharedPointer< AbstractTemplateLoader > | loader | ) |
Adds loader
to the TemplateLoaders currently configured on the Engine.
Definition at line 55 of file engine.cpp.
◆ defaultLibraries()
QStringList Engine::defaultLibraries | ( | ) | const |
Returns the libraries available by default to new Templates.
Definition at line 101 of file engine.cpp.
◆ loadByName()
Load the Template identified by name
.
The Templates and plugins loaded will be determined by the Engine configuration.
Definition at line 311 of file engine.cpp.
◆ mediaUri()
Returns a URI for a media item with the name name
.
Typically this will be used for images. For example the media URI for the image "header_logo.png"
may be "/home/user/common/header_logo.png"
or "/home/user/some_theme/header_logo.png"
depending on the templateLoaders configured.
This method will not usually be called by application code. To load media in a template, use the {% media_finder %}
template tag.
Definition at line 61 of file engine.cpp.
◆ newTemplate()
Create a new Template with the content content
identified by name
.
The secondary Templates and plugins loaded will be determined by the Engine configuration.
Definition at line 332 of file engine.cpp.
◆ pluginPaths()
QStringList Engine::pluginPaths | ( | ) | const |
Returns the currently configured plugin dirs.
Definition at line 95 of file engine.cpp.
◆ removeDefaultLibrary()
void Engine::removeDefaultLibrary | ( | const QString & | libName | ) |
Removes the library named libName
from the libraries available by default to new Templates.
Definition at line 113 of file engine.cpp.
◆ removePluginPath()
void Engine::removePluginPath | ( | const QString & | dir | ) |
Removes all instances of dir
from the list of plugin dirs.
Definition at line 89 of file engine.cpp.
◆ setPluginPaths()
void Engine::setPluginPaths | ( | const QStringList & | dirs | ) |
Sets the plugin dirs currently configured on the Engine to dirs
.
- Warning
- This overwrites the default paths. You normally want addPluginPath.
- See also
- Finding tags and filters
Definition at line 74 of file engine.cpp.
◆ setSmartTrimEnabled()
void Engine::setSmartTrimEnabled | ( | bool | enabled | ) |
Sets whether the smart trim feature is enabled for newly loaded templates.
- See also
- smart_trim
Definition at line 341 of file engine.cpp.
◆ smartTrimEnabled()
bool Engine::smartTrimEnabled | ( | ) | const |
Returns whether the smart trim feature is enabled for newly loaded templates.
- See also
- smart_trim
This is false by default.
Definition at line 347 of file engine.cpp.
◆ templateLoaders()
QList< QSharedPointer< AbstractTemplateLoader > > Engine::templateLoaders | ( | ) |
Returns the TemplateLoaders currently configured on the Engine.
Definition at line 49 of file engine.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:56:17 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.