KViewStateSerializer
#include <KViewStateSerializer>
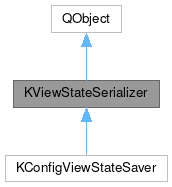
Public Member Functions | |
KViewStateSerializer (QObject *parent=nullptr) | |
~KViewStateSerializer () override | |
QString | currentIndexKey () const |
QStringList | expansionKeys () const |
void | restoreCurrentItem (const QString &indexString) |
void | restoreExpanded (const QStringList &indexStrings) |
void | restoreScrollState (int verticalScoll, int horizontalScroll) |
void | restoreSelection (const QStringList &indexStrings) |
QPair< int, int > | scrollState () const |
QStringList | selectionKeys () const |
QItemSelectionModel * | selectionModel () const |
void | setSelectionModel (QItemSelectionModel *selectionModel) |
void | setView (QAbstractItemView *view) |
QAbstractItemView * | view () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
virtual QModelIndex | indexFromConfigString (const QAbstractItemModel *model, const QString &key) const =0 |
virtual QString | indexToConfigString (const QModelIndex &index) const =0 |
void | restoreState () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
Object for saving and restoring state in QTreeViews and QItemSelectionModels.
Implement the indexFromConfigString and indexToConfigString methods to handle the model in the view whose state is being saved. These implementations can be quite trivial:
It is possible to restore the state of a QTreeView (that is, the expanded state and selected state of all indexes as well as the horizontal and vertical scroll state) by using setView.
If there is no tree view state to restore (for example if using QML), the selection state of a QItemSelectionModel can be saved or restored instead.
The state of any QAbstractScrollArea can also be saved and restored.
A KViewStateSerializer should be created on the stack when saving and on the heap when restoring. The model may be populated dynamically between several event loops, so it may not be immediate for the indexes that should be selected to be in the model. The saver should not be persisted as a member. The saver will destroy itself when it has completed the restoration specified in the config group, or a small amount of time has elapsed.
After creating a saver, the state can be saved using a KConfigGroup.
It is also possible to save and restore state directly by using the restoreSelection, restoreExpanded etc methods. Note that the implementation of these methods should return strings that the indexFromConfigString implementation can handle.
Note that a single instance of this class should be used with only one widget. That is don't do this:
To save the state of 3 different widgets, use three savers, even if they operate on the same root model.
- Note
- The KViewStateSerializer does not take ownership of any widgets set on it.
It is recommended to restore the state on application startup and after the model has been reset, and to save the state on application close and before the model has been reset.
- Since
- 4.5
Definition at line 168 of file kviewstateserializer.h.
Constructor & Destructor Documentation
◆ KViewStateSerializer()
|
explicit |
Constructor.
Definition at line 96 of file kviewstateserializer.cpp.
◆ ~KViewStateSerializer()
|
overridedefault |
Destructor.
Member Function Documentation
◆ currentIndexKey()
QString KViewStateSerializer::currentIndexKey | ( | ) | const |
Returns a QString describing the current index in the selection model.
Definition at line 307 of file kviewstateserializer.cpp.
◆ expansionKeys()
QStringList KViewStateSerializer::expansionKeys | ( | ) | const |
Returns a QStringList representing the expanded indexes in the QTreeView.
Definition at line 316 of file kviewstateserializer.cpp.
◆ indexFromConfigString()
|
protectedpure virtual |
Reimplement to return an index in the model
described by the unique key key
.
◆ indexToConfigString()
|
protectedpure virtual |
Reimplement to return a unique string for the index
.
◆ restoreCurrentItem()
void KViewStateSerializer::restoreCurrentItem | ( | const QString & | indexString | ) |
Make the index described by indexString
the currentIndex in the selectionModel.
Definition at line 202 of file kviewstateserializer.cpp.
◆ restoreExpanded()
void KViewStateSerializer::restoreExpanded | ( | const QStringList & | indexStrings | ) |
Expand the indexes described by indexStrings
in the QTreeView.
Definition at line 236 of file kviewstateserializer.cpp.
◆ restoreScrollState()
void KViewStateSerializer::restoreScrollState | ( | int | verticalScoll, |
int | horizontalScroll ) |
Restores the scroll state of the QAbstractScrollArea to the verticalScoll
and horizontalScroll
.
Definition at line 255 of file kviewstateserializer.cpp.
◆ restoreSelection()
void KViewStateSerializer::restoreSelection | ( | const QStringList & | indexStrings | ) |
Select the indexes described by indexStrings
.
Definition at line 287 of file kviewstateserializer.cpp.
◆ restoreState()
|
protected |
Definition at line 349 of file kviewstateserializer.cpp.
◆ scrollState()
QPair< int, int > KViewStateSerializer::scrollState | ( | ) | const |
Returns the vertical and horizontal scroll of the QAbstractScrollArea.
Definition at line 343 of file kviewstateserializer.cpp.
◆ selectionKeys()
QStringList KViewStateSerializer::selectionKeys | ( | ) | const |
Returns a QStringList describing the selection in the selectionModel.
Definition at line 326 of file kviewstateserializer.cpp.
◆ selectionModel()
QItemSelectionModel * KViewStateSerializer::selectionModel | ( | ) | const |
The QItemSelectionModel whose state is persisted.
Definition at line 126 of file kviewstateserializer.cpp.
◆ setSelectionModel()
void KViewStateSerializer::setSelectionModel | ( | QItemSelectionModel * | selectionModel | ) |
Sets the QItemSelectionModel whose state is persisted.
Definition at line 132 of file kviewstateserializer.cpp.
◆ setView()
void KViewStateSerializer::setView | ( | QAbstractItemView * | view | ) |
Sets the view whose state is persisted.
Definition at line 106 of file kviewstateserializer.cpp.
◆ view()
QAbstractItemView * KViewStateSerializer::view | ( | ) | const |
The view whose state is persisted.
Definition at line 120 of file kviewstateserializer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:09:52 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.