Syndication::Atom::Content
#include <content.h>
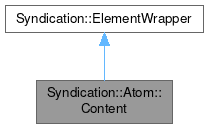
Public Types | |
enum | Format { PlainText , EscapedHTML , XML , Binary } |
Public Member Functions | |
Content () | |
Content (const Content &other) | |
Content (const QDomElement &element) | |
~Content () override | |
QByteArray | asByteArray () const |
QString | asString () const |
QString | debugInfo () const |
Format | format () const |
bool | isBinary () const |
bool | isContained () const |
bool | isEscapedHTML () const |
bool | isPlainText () const |
bool | isXML () const |
Content & | operator= (const Content &other) |
QString | src () const |
QString | type () const |
![]() | |
ElementWrapper () | |
ElementWrapper (const ElementWrapper &other) | |
ElementWrapper (const QDomElement &element) | |
virtual | ~ElementWrapper () |
QString | attribute (const QString &name, const QString &defValue=QString()) const |
QString | attributeNS (const QString &nsURI, const QString &localName, const QString &defValue=QString()) const |
QString | childNodesAsXML () const |
QString | completeURI (const QString &uri) const |
const QDomElement & | element () const |
QList< QDomElement > | elementsByTagName (const QString &tagName) const |
QList< QDomElement > | elementsByTagNameNS (const QString &nsURI, const QString &tagName) const |
QString | extractElementText (const QString &tagName) const |
QString | extractElementTextNS (const QString &namespaceURI, const QString &localName) const |
QDomElement | firstElementByTagNameNS (const QString &nsURI, const QString &tagName) const |
bool | hasAttribute (const QString &name) const |
bool | hasAttributeNS (const QString &nsURI, const QString &localName) const |
bool | isNull () const |
ElementWrapper & | operator= (const ElementWrapper &other) |
bool | operator== (const ElementWrapper &other) const |
QString | text () const |
QString | xmlBase () const |
QString | xmlLang () const |
Static Public Member Functions | |
static Format | mapTypeToFormat (const QString &type, const QString &src=QString()) |
![]() | |
static QString | childNodesAsXML (const QDomElement &parent) |
Detailed Description
The content element either contains or links the content of an entry.
The content is usually plain text or HTML, but arbitrary XML or binary content are also possible. If isContained() is false, the content is not contained in the feed source, but linked.
Member Enumeration Documentation
◆ Format
Constructor & Destructor Documentation
◆ Content() [1/3]
Syndication::Atom::Content::Content | ( | ) |
creates a null content object.
Definition at line 32 of file content.cpp.
◆ Content() [2/3]
|
explicit |
creates a Content object wrapping an atom:content element.
- Parameters
-
element a DOM element, should be a atom:content element (although not enforced), otherwise this object will not parse anything useful
Definition at line 38 of file content.cpp.
◆ Content() [3/3]
Syndication::Atom::Content::Content | ( | const Content & | other | ) |
creates a copy of another Content object
- Parameters
-
other the content object to copy
Definition at line 44 of file content.cpp.
◆ ~Content()
|
override |
destructor
Definition at line 50 of file content.cpp.
Member Function Documentation
◆ asByteArray()
QByteArray Syndication::Atom::Content::asByteArray | ( | ) | const |
returns binary content as byte array.
- Returns
- byte array containing the embedded binary data, or an empty array if the content is not in binary format
Definition at line 71 of file content.cpp.
◆ asString()
QString Syndication::Atom::Content::asString | ( | ) | const |
returns the content as string.
If the content format is Text, the returned string contains the text as HTML. If the content is embedded XML, the XML is returned as string.
- Returns
- a string representation of the content, or a null string if the content is either binary content or not contained but linked (see isContained())
Definition at line 158 of file content.cpp.
◆ debugInfo()
QString Syndication::Atom::Content::debugInfo | ( | ) | const |
returns a description of the content object for debugging purposes
- Returns
- debug string
Definition at line 173 of file content.cpp.
◆ format()
Content::Format Syndication::Atom::Content::format | ( | ) | const |
returns the content format
Definition at line 124 of file content.cpp.
◆ isBinary()
bool Syndication::Atom::Content::isBinary | ( | ) | const |
returns whether the content is binary content or not.
Use asByteArray() to access it.
Definition at line 133 of file content.cpp.
◆ isContained()
bool Syndication::Atom::Content::isContained | ( | ) | const |
returns whether the content is contained in the feed source, or not.
If it is not contained, src() provides a URL to the content.
Definition at line 138 of file content.cpp.
◆ isEscapedHTML()
bool Syndication::Atom::Content::isEscapedHTML | ( | ) | const |
returns whether the content is escaped HTML or not Use asString() to access it
Definition at line 148 of file content.cpp.
◆ isPlainText()
bool Syndication::Atom::Content::isPlainText | ( | ) | const |
returns whether the content is plain text or not.
Use asString() to access it.
Definition at line 143 of file content.cpp.
◆ isXML()
bool Syndication::Atom::Content::isXML | ( | ) | const |
returns whether the content is embedded XML.
Use element() to access the DOM tree, or asString() to get the XML as string.
Definition at line 153 of file content.cpp.
◆ mapTypeToFormat()
|
static |
maps a mimetype to Format enum according to the Atom 1.0 specification
- Parameters
-
type a valid mimetype, or one of "text", "html", "xhtml" src content source, see src() for details.
Definition at line 79 of file content.cpp.
◆ operator=()
assigns another content objecct
- Parameters
-
other the Content object to assign
Definition at line 54 of file content.cpp.
◆ src()
QString Syndication::Atom::Content::src | ( | ) | const |
If src() is set, the content of this entry is not contained in the feed source, but available on the net.
src() then contains a URL (more precise: IRI reference) linking to remote content. If src is provided, type() should contain a mimetype, instead of "text", "html" or "xhtml".
- Returns
- a null string if the content is contained in the feed source, or a URL linking to the remote content
Definition at line 66 of file content.cpp.
◆ type()
QString Syndication::Atom::Content::type | ( | ) | const |
the type of the content.
This is either "text" (plain text), "html" (escaped HTML), "xhtml" (embedded XHTML) or a mime type
- Returns
- the content type. If no type is specified, "text" (the default) is returned.
Definition at line 61 of file content.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:09:18 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.