Syndication::RSS2::Document
#include <document.h>
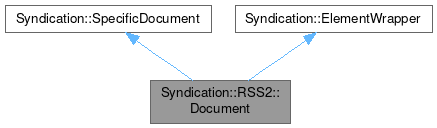
Public Types | |
enum | DayOfWeek { Monday = 0 , Tuesday = 1 , Wednesday = 2 , Thursday = 3 , Friday = 4 , Saturday = 5 , Sunday = 6 } |
Static Public Member Functions | |
static Document | fromXML (const QDomDocument &document) |
![]() | |
static QString | childNodesAsXML (const QDomElement &parent) |
Public Attributes | |
QSharedPointer< DocumentPrivate > | d |
Detailed Description
document implementation, representing an RSS feed from the 0.91-0.94/2.0 family.
Definition at line 43 of file rss2/document.h.
Member Enumeration Documentation
◆ DayOfWeek
days of week, used for skip days
Enumerator | |
---|---|
Monday | self-explanatory |
Tuesday | self-explanatory |
Wednesday | self-explanatory |
Thursday | self-explanatory |
Friday | self-explanatory |
Saturday | self-explanatory |
Sunday | self-explanatory |
Definition at line 239 of file rss2/document.h.
Constructor & Destructor Documentation
◆ Document() [1/3]
Document::Document | ( | ) |
Default constructor, creates a null object, for which isNull() is true
and isValid() is false
.
Definition at line 64 of file rss2/document.cpp.
◆ Document() [2/3]
Document::Document | ( | const Document & | other | ) |
copy constructor
Definition at line 71 of file rss2/document.cpp.
◆ ~Document()
|
override |
destructor
Definition at line 78 of file rss2/document.cpp.
◆ Document() [3/3]
|
explicit |
private: /**
private constructor, used by fromXML() TODO: remove fromXML(), make this one private
Definition at line 50 of file rss2/document.cpp.
Member Function Documentation
◆ accept()
|
overridevirtual |
Used by visitors for double dispatch.
See DocumentVisitor for more information.
- Parameters
-
visitor the visitor calling the method
Implements Syndication::SpecificDocument.
Definition at line 463 of file rss2/document.cpp.
◆ categories()
Specifies one or more categories that the channel belongs to.
- Returns
- TODO
Definition at line 161 of file rss2/document.cpp.
◆ cloud()
Cloud Document::cloud | ( | ) | const |
Allows processes to register with a cloud to be notified of updates to the channel, implementing a lightweight publish-subscribe protocol for RSS feeds.
- Returns
- cloud information, or a null object if not set
Definition at line 185 of file rss2/document.cpp.
◆ copyright()
QString Document::copyright | ( | ) | const |
Copyright notice for content in the channel.
This method returns the content of the <copyright>
element. If <copyright>
is not available, the method returns <dc:rights>
instead, if available.
- Returns
- copyright information, or a null string if not set
Definition at line 120 of file rss2/document.cpp.
◆ debugInfo()
|
overridevirtual |
Returns a description of the object and its children for debugging purposes.
- Returns
- debug string
Implements Syndication::SpecificDocument.
Definition at line 331 of file rss2/document.cpp.
◆ description()
QString Document::description | ( | ) | const |
Phrase or sentence describing the channel.
- Returns
- TODO
Definition at line 103 of file rss2/document.cpp.
◆ docs()
QString Document::docs | ( | ) | const |
A URL that points to the documentation for the format used in the RSS file.
It's probably a pointer to the RSS specification. It's for people who might stumble across an RSS file on a Web server 25 years from now and wonder what it is.
- Returns
- URL pointing to the format specification, or a null string if not set
Definition at line 180 of file rss2/document.cpp.
◆ fromXML()
|
static |
Parses an RSS2 document from an XML document.
TODO: More on supported formats etc.
- Parameters
-
document The dom document to parse the document from
- Returns
- the document parsed from XML, or an invalid document if parsing failed.
Definition at line 57 of file rss2/document.cpp.
◆ generator()
QString Document::generator | ( | ) | const |
A string indicating the program used to generate the channel.
- Returns
- description of the generator program, or a null string if not set
Definition at line 175 of file rss2/document.cpp.
◆ image()
Image Document::image | ( | ) | const |
Specifies a GIF, JPEG or PNG image that can be displayed with the channel.
- Returns
- the image, or a null object if not set
Definition at line 200 of file rss2/document.cpp.
◆ isValid()
|
overridevirtual |
returns whether this document is valid or not.
Invalid documents do not contain any useful information.
Implements Syndication::SpecificDocument.
Definition at line 88 of file rss2/document.cpp.
◆ items()
the items contained in this document
Definition at line 271 of file rss2/document.cpp.
◆ language()
QString Document::language | ( | ) | const |
- Returns
- TODO
Definition at line 109 of file rss2/document.cpp.
◆ lastBuildDate()
time_t Document::lastBuildDate | ( | ) | const |
The last time the content of the channel changed.
- Returns
- the last build date, or 0 if no date was specified or parsing failed
Definition at line 154 of file rss2/document.cpp.
◆ link()
QString Document::link | ( | ) | const |
The URL to the HTML website corresponding to the channel.
- Returns
- TODO
Definition at line 98 of file rss2/document.cpp.
◆ managingEditor()
QString Document::managingEditor | ( | ) | const |
Email address for person responsible for editorial content.
- Returns
- editor's email address, or a null string if not set
Definition at line 131 of file rss2/document.cpp.
◆ operator=()
assigns another document.
As the d pointer is shared, this is a cheap operation.
- Parameters
-
other the document to assign
Definition at line 82 of file rss2/document.cpp.
◆ pubDate()
time_t Document::pubDate | ( | ) | const |
The publication date for the content in the channel.
For example, the New York Times publishes on a daily basis, the publication date flips once every 24 hours. That's when the pubDate of the channel changes. This method returns the content of the <pubDate>
element. If <pubDate>
is not available, the method returns <dc:date>
instead, if available.
- Returns
- the publication date, or 0 if no date was specified or parsing failed
Definition at line 141 of file rss2/document.cpp.
◆ skipDays()
QSet< Document::DayOfWeek > Document::skipDays | ( | ) | const |
A set of week days where aggregators shouldn't read the channel.
Definition at line 236 of file rss2/document.cpp.
◆ skipHours()
QSet< int > Document::skipHours | ( | ) | const |
Contains a set of hours (from 0 to 23), time in GMT, when the channel is not updated.
Definition at line 217 of file rss2/document.cpp.
◆ textInput()
TextInput Document::textInput | ( | ) | const |
Specifies a text input box that can be displayed with the channel.
- Returns
- the text input, or a null object if not set
Definition at line 205 of file rss2/document.cpp.
◆ title()
QString Document::title | ( | ) | const |
The title of the channel.
- Returns
- title TODO: more on escaping/HTML
Definition at line 93 of file rss2/document.cpp.
◆ ttl()
int Document::ttl | ( | ) | const |
ttl stands for time to live.
It's a number of minutes that indicates how long a channel can be cached before refreshing from the source.
- Returns
- the "time to live" in minutes, or 0 if not set
Definition at line 190 of file rss2/document.cpp.
◆ unhandledElements()
QList< QDomElement > Document::unhandledElements | ( | ) | const |
returns all child elements of this document not covered by this class.
You can use this to access additional metadata from RSS extensions.
Definition at line 286 of file rss2/document.cpp.
◆ webMaster()
QString Document::webMaster | ( | ) | const |
Email address for person responsible for technical issues relating to channel.
- Returns
- web master's email address, or a null string if not
Definition at line 136 of file rss2/document.cpp.
Member Data Documentation
◆ d
QSharedPointer<DocumentPrivate> Syndication::RSS2::Document::d |
Definition at line 299 of file rss2/document.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Oct 11 2024 12:09:52 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.