KPublicTransport::OnboardStatus
#include <onboardstatus.h>
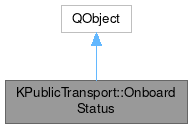
Public Types | |
enum | Status { NotConnected , Onboard , MissingPermissions , WifiNotEnabled , LocationServiceNotEnabled , NotAvailable } |
Properties | |
float | altitude |
bool | hasAltitude |
bool | hasHeading |
bool | hasJourney |
bool | hasPosition |
bool | hasSpeed |
float | heading |
KPublicTransport::Journey | journey |
int | journeyUpdateInterval |
float | latitude |
float | longitude |
int | positionUpdateInterval |
float | speed |
Status | status |
bool | supportsJourney |
bool | supportsPosition |
![]() | |
objectName | |
Signals | |
void | journeyChanged () |
void | positionChanged () |
void | statusChanged () |
void | supportsJourneyChanged () |
void | supportsPositionChanged () |
void | updateIntervalChanged () |
Public Member Functions | |
OnboardStatus (QObject *parent=nullptr) | |
float | altitude () const |
bool | hasAltitude () const |
bool | hasHeading () const |
bool | hasJourney () const |
bool | hasPosition () const |
bool | hasSpeed () const |
float | heading () const |
KPublicTransport::Journey | journey () const |
int | journeyUpdateInterval () const |
float | latitude () const |
float | longitude () const |
int | positionUpdateInterval () const |
Q_INVOKABLE void | requestJourney () |
Q_INVOKABLE void | requestPermissions () |
Q_INVOKABLE void | requestPosition () |
void | setJourneyUpdateInterval (int interval) |
void | setPositionUpdateInterval (int interval) |
float | speed () const |
Status | status () const |
bool | supportsJourney () const |
bool | supportsPosition () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Access to public transport onboard API.
Instances of this class act as a lightweight frontend to an internal singleton, for easy integration with QML.
Definition at line 24 of file onboardstatus.h.
Member Enumeration Documentation
◆ Status
Definition at line 64 of file onboardstatus.h.
Property Documentation
◆ altitude
|
read |
Current altitude in meters, NAN if unknown.
Definition at line 46 of file onboardstatus.h.
◆ hasAltitude
|
read |
Definition at line 47 of file onboardstatus.h.
◆ hasHeading
|
read |
Definition at line 43 of file onboardstatus.h.
◆ hasJourney
|
read |
Whether there is journey information available.
Definition at line 52 of file onboardstatus.h.
◆ hasPosition
|
read |
Whether the geographic position is currently available.
Definition at line 33 of file onboardstatus.h.
◆ hasSpeed
|
read |
Definition at line 39 of file onboardstatus.h.
◆ heading
|
read |
Current heading in degree, NAN if unknown.
Definition at line 42 of file onboardstatus.h.
◆ journey
|
read |
The current journey.
Definition at line 50 of file onboardstatus.h.
◆ journeyUpdateInterval
|
readwrite |
Definition at line 58 of file onboardstatus.h.
◆ latitude
|
read |
Current geographic position, NAN
if not available.
Definition at line 30 of file onboardstatus.h.
◆ longitude
|
read |
Definition at line 31 of file onboardstatus.h.
◆ positionUpdateInterval
|
readwrite |
Update polling intervals in seconds.
Definition at line 57 of file onboardstatus.h.
◆ speed
|
read |
Current speed in km/h.
Definition at line 38 of file onboardstatus.h.
◆ status
|
read |
Definition at line 27 of file onboardstatus.h.
◆ supportsJourney
|
read |
Whether the backend supports querying for the journey.
Definition at line 54 of file onboardstatus.h.
◆ supportsPosition
|
read |
Whether the backend supports querying for the geographic position.
Definition at line 35 of file onboardstatus.h.
Constructor & Destructor Documentation
◆ OnboardStatus()
|
explicit |
Definition at line 24 of file onboardstatus.cpp.
◆ ~OnboardStatus()
OnboardStatus::~OnboardStatus | ( | ) |
Definition at line 37 of file onboardstatus.cpp.
Member Function Documentation
◆ altitude()
float OnboardStatus::altitude | ( | ) | const |
Definition at line 87 of file onboardstatus.cpp.
◆ hasAltitude()
bool OnboardStatus::hasAltitude | ( | ) | const |
Definition at line 92 of file onboardstatus.cpp.
◆ hasHeading()
bool OnboardStatus::hasHeading | ( | ) | const |
Definition at line 82 of file onboardstatus.cpp.
◆ hasJourney()
bool OnboardStatus::hasJourney | ( | ) | const |
Definition at line 102 of file onboardstatus.cpp.
◆ hasPosition()
bool OnboardStatus::hasPosition | ( | ) | const |
Definition at line 57 of file onboardstatus.cpp.
◆ hasSpeed()
bool OnboardStatus::hasSpeed | ( | ) | const |
Definition at line 72 of file onboardstatus.cpp.
◆ heading()
float OnboardStatus::heading | ( | ) | const |
Definition at line 77 of file onboardstatus.cpp.
◆ journey()
Journey OnboardStatus::journey | ( | ) | const |
Definition at line 97 of file onboardstatus.cpp.
◆ journeyUpdateInterval()
int OnboardStatus::journeyUpdateInterval | ( | ) | const |
Definition at line 127 of file onboardstatus.cpp.
◆ latitude()
float OnboardStatus::latitude | ( | ) | const |
Definition at line 47 of file onboardstatus.cpp.
◆ longitude()
float OnboardStatus::longitude | ( | ) | const |
Definition at line 52 of file onboardstatus.cpp.
◆ positionUpdateInterval()
int OnboardStatus::positionUpdateInterval | ( | ) | const |
Definition at line 112 of file onboardstatus.cpp.
◆ requestJourney()
void OnboardStatus::requestJourney | ( | ) |
Request one time journey data update, if available.
For recurring updates, use the polling interval setting instead.
Definition at line 147 of file onboardstatus.cpp.
◆ requestPermissions()
void OnboardStatus::requestPermissions | ( | ) |
Request application permission needed for Wi-Fi montioring.
Only relevant on Android, does nothing on other platforms.
- See also
- Status::MissingPermissions
Definition at line 152 of file onboardstatus.cpp.
◆ requestPosition()
void OnboardStatus::requestPosition | ( | ) |
Request one time update of the position status.
For recurring updates, use the polling interval setting instead.
Definition at line 142 of file onboardstatus.cpp.
◆ setJourneyUpdateInterval()
void OnboardStatus::setJourneyUpdateInterval | ( | int | interval | ) |
Definition at line 132 of file onboardstatus.cpp.
◆ setPositionUpdateInterval()
void OnboardStatus::setPositionUpdateInterval | ( | int | interval | ) |
Definition at line 117 of file onboardstatus.cpp.
◆ speed()
float OnboardStatus::speed | ( | ) | const |
Definition at line 67 of file onboardstatus.cpp.
◆ status()
OnboardStatus::Status OnboardStatus::status | ( | ) | const |
Definition at line 42 of file onboardstatus.cpp.
◆ supportsJourney()
bool OnboardStatus::supportsJourney | ( | ) | const |
Definition at line 107 of file onboardstatus.cpp.
◆ supportsPosition()
bool OnboardStatus::supportsPosition | ( | ) | const |
Definition at line 62 of file onboardstatus.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:47:05 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.