Okular::Generator
#include <generator.h>
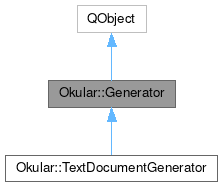
Public Types | |
enum | GeneratorFeature { Threaded , TextExtraction , ReadRawData , FontInfo , PageSizes , PrintNative , PrintPostscript , PrintToFile , TiledRendering , SwapBackingFile , SupportsCancelling } |
enum | PageLayout { NoLayout = -1 , SinglePage , TwoPageLeft , TwoPageRight } |
enum | PageSizeMetric { None , Points , Pixels } |
enum | SwapBackingFileResult { SwapBackingFileError , SwapBackingFileNoOp , SwapBackingFileReloadInternalData } |
Signals | |
void | error (const QString &message, int duration) |
void | notice (const QString &message, int duration) |
void | warning (const QString &message, int duration) |
Public Member Functions | |
Generator (QObject *parent=nullptr, const QVariantList &args=QVariantList()) | |
~Generator () override | |
virtual Okular::Action * | additionalDocumentAction (Document::DocumentAdditionalActionType type) |
virtual bool | canGeneratePixmap () const |
virtual bool | canGenerateTextPage () const |
virtual bool | canSign () const |
virtual CertificateStore * | certificateStore () const |
bool | closeDocument () |
virtual bool | defaultPageContinuous () const |
virtual PageLayout | defaultPageLayout () const |
virtual const QList< EmbeddedFile * > * | embeddedFiles () const |
virtual ExportFormat::List | exportFormats () const |
virtual bool | exportTo (const QString &fileName, const ExportFormat &format) |
virtual FontInfo::List | fontsForPage (int page) |
virtual void | freeOpaqueActionContents (const BackendOpaqueAction &action) |
virtual DocumentInfo | generateDocumentInfo (const QSet< DocumentInfo::Key > &keys) const |
virtual const DocumentSynopsis * | generateDocumentSynopsis () |
virtual void | generatePixmap (PixmapRequest *request) |
void | generateTextPage (Page *page) |
bool | hasFeature (GeneratorFeature feature) const |
virtual bool | isAllowed (Permission action) const |
virtual QAbstractItemModel * | layersModel () const |
virtual bool | loadDocument (const QString &fileName, QVector< Page * > &pagesVector) |
virtual bool | loadDocumentFromData (const QByteArray &fileData, QVector< Page * > &pagesVector) |
virtual Document::OpenResult | loadDocumentFromDataWithPassword (const QByteArray &fileData, QVector< Page * > &pagesVector, const QString &password) |
virtual Document::OpenResult | loadDocumentWithPassword (const QString &fileName, QVector< Page * > &pagesVector, const QString &password) |
virtual QVariant | metaData (const QString &key, const QVariant &option) const |
virtual BackendOpaqueAction::OpaqueActionResult | opaqueAction (const BackendOpaqueAction *action) |
virtual void | pageSizeChanged (const PageSize &pageSize, const PageSize &oldPageSize) |
virtual PageSize::List | pageSizes () const |
virtual PageSizeMetric | pagesSizeMetric () const |
virtual Document::PrintError | print (QPrinter &printer) |
virtual void | rotationChanged (Rotation orientation, Rotation oldOrientation) |
void | setDPI (const QSizeF dpi) |
virtual bool | sign (const NewSignatureData &data, const QString &rFilename) |
virtual SwapBackingFileResult | swapBackingFile (const QString &newFileName, QVector< Okular::Page * > &newPagesVector) |
virtual void | walletDataForFile (const QString &fileName, QString *walletName, QString *walletFolder, QString *walletKey) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Types | |
enum | DocumentMetaDataKey { PaperColorMetaData , TextAntialiasMetaData , GraphicsAntialiasMetaData , TextHintingMetaData } |
Protected Slots | |
void | signalPartialPixmapRequest (Okular::PixmapRequest *request, const QImage &image) |
Protected Member Functions | |
virtual bool | doCloseDocument ()=0 |
const Document * | document () const |
QVariant | documentMetaData (const DocumentMetaDataKey key, const QVariant &option=QVariant()) const |
QSizeF | dpi () const |
virtual QImage | image (PixmapRequest *request) |
virtual QByteArray | requestFontData (const Okular::FontInfo &font) |
void | setFeature (GeneratorFeature feature, bool on=true) |
void | signalPixmapRequestDone (PixmapRequest *request) |
void | signalTextGenerationDone (Page *page, TextPage *textPage) |
virtual TextPage * | textPage (TextRequest *request) |
void | updatePageBoundingBox (int page, const NormalizedRect &boundingBox) |
QMutex * | userMutex () const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
[Abstract Class] The information generator.
Most of class members are virtuals and some of them pure virtual. The pure virtuals provide the minimal functionalities for a Generator, that is being able to generate QPixmap for the Page 's of the Document.
Implementing the other functions will make the Generator able to provide more contents and/or functionalities (like text extraction).
Generation/query is requested by the Document class only, and that class stores the resulting data into Page s. The data will then be displayed by the GUI components (PageView, ThumbnailList, etc..).
- See also
- PrintInterface, ConfigInterface, GuiInterface
Definition at line 188 of file generator.h.
Member Enumeration Documentation
◆ DocumentMetaDataKey
|
protected |
Internal document setting.
Enumerator | |
---|---|
PaperColorMetaData | Returns (QColor) the paper color if set in Settings or the default color (white) if option is true (otherwise returns a non initialized QColor) |
TextAntialiasMetaData | Returns (bool) text antialias from Settings (option is not used) |
GraphicsAntialiasMetaData | Returns (bool)graphic antialias from Settings (option is not used) |
TextHintingMetaData | Returns (bool)text hinting from Settings (option is not used) |
Definition at line 576 of file generator.h.
◆ GeneratorFeature
Describe the possible optional features that a Generator can provide.
Enumerator | |
---|---|
Threaded | Whether the Generator supports asynchronous generation of pictures or text pages. |
TextExtraction | Whether the Generator can extract text from the document in the form of TextPage's. |
ReadRawData | Whether the Generator can read a document directly from its raw data. |
FontInfo | Whether the Generator can provide information about the fonts used in the document. |
PageSizes | Whether the Generator can change the size of the document pages. |
PrintNative | Whether the Generator supports native cross-platform printing (QPainter-based). |
PrintPostscript | Whether the Generator supports postscript-based file printing. |
PrintToFile | Whether the Generator supports export to PDF & PS through the Print Dialog. |
TiledRendering | Whether the Generator can render tiles.
|
SwapBackingFile | Whether the Generator can hot-swap the file it's reading from.
|
SupportsCancelling | Whether the Generator can cancel requests.
|
Definition at line 202 of file generator.h.
◆ PageLayout
This enum identifies default page layouts.
- Since
- 24.12
Definition at line 370 of file generator.h.
◆ PageSizeMetric
This enum identifies the metric of the page size.
Enumerator | |
---|---|
None | The page size is not defined in a physical metric. |
Points | The page size is given in 1/72 inches. |
Pixels | The page size is given in screen pixels.
|
Definition at line 394 of file generator.h.
◆ SwapBackingFileResult
Describes the result of an swap file operation.
- Since
- 1.3
Definition at line 279 of file generator.h.
Constructor & Destructor Documentation
◆ Generator()
|
explicit |
Creates a new generator.
Definition at line 174 of file generator.cpp.
◆ ~Generator()
|
override |
Destroys the generator.
Definition at line 188 of file generator.cpp.
Member Function Documentation
◆ additionalDocumentAction()
|
virtual |
Retrieves the additional document action for the specified type
.
- Since
- 24.08
Definition at line 486 of file generator.cpp.
◆ canGeneratePixmap()
|
virtual |
This method returns whether the generator is ready to handle a new pixmap request.
Reimplemented in Okular::TextDocumentGenerator.
Definition at line 248 of file generator.cpp.
◆ canGenerateTextPage()
|
virtual |
This method returns whether the generator is ready to handle a new text page request.
Definition at line 319 of file generator.cpp.
◆ canSign()
|
virtual |
Definition at line 254 of file generator.cpp.
◆ certificateStore()
|
virtual |
Definition at line 264 of file generator.cpp.
◆ closeDocument()
bool Generator::closeDocument | ( | ) |
This method is called when the document is closed and not used any longer.
- Returns
- true on success, false otherwise.
Definition at line 221 of file generator.cpp.
◆ defaultPageContinuous()
|
virtual |
This method returns if the default page layout is continuous.
- Since
- 24.12
Definition at line 371 of file generator.cpp.
◆ defaultPageLayout()
|
virtual |
This method returns the default page layout.
- Since
- 24.12
Definition at line 366 of file generator.cpp.
◆ doCloseDocument()
|
protectedpure virtual |
This method is called when the document is closed and not used any longer.
- Returns
- true on success, false otherwise.
Implemented in Okular::TextDocumentGenerator.
◆ document()
|
protected |
Returns a pointer to the document.
Definition at line 477 of file generator.cpp.
◆ documentMetaData()
|
protected |
Request a meta data of the Document, if available, like an internal setting.
- Since
- 1.1
Definition at line 501 of file generator.cpp.
◆ dpi()
|
protected |
Returns DPI, previously set via setDPI()
- Since
- 0.19 (KDE 4.13)
Definition at line 536 of file generator.cpp.
◆ embeddedFiles()
|
virtual |
Returns the 'list of embedded files' object of the document or 0 if no list of embedded files is available.
Definition at line 361 of file generator.cpp.
◆ error
|
signal |
This signal should be emitted whenever an error occurred in the generator.
- Parameters
-
message The message which should be shown to the user. duration The time that the message should be shown to the user.
◆ exportFormats()
|
virtual |
Returns the list of additional supported export formats.
Reimplemented in Okular::TextDocumentGenerator.
Definition at line 419 of file generator.cpp.
◆ exportTo()
|
virtual |
This method is called to export the document in the given format
and save it under the given fileName
.
The format must be one of the supported export formats.
Reimplemented in Okular::TextDocumentGenerator.
Definition at line 424 of file generator.cpp.
◆ fontsForPage()
|
virtual |
Returns the 'list of embedded fonts' object of the specified page
of the document.
- Parameters
-
page a page of the document, starting from 0 - -1 indicates all the other fonts
Definition at line 356 of file generator.cpp.
◆ freeOpaqueActionContents()
|
virtual |
Frees the contents of the opaque action (if any);.
- Since
- 22.04
Definition at line 409 of file generator.cpp.
◆ generateDocumentInfo()
|
virtual |
Returns the general information object of the document.
Changed signature in okular version 0.21
Reimplemented in Okular::TextDocumentGenerator.
Definition at line 344 of file generator.cpp.
◆ generateDocumentSynopsis()
|
virtual |
Returns the 'table of content' object of the document or 0 if no table of content is available.
Reimplemented in Okular::TextDocumentGenerator.
Definition at line 351 of file generator.cpp.
◆ generatePixmap()
|
virtual |
This method can be called to trigger the generation of a new pixmap as described by request
.
We create the text page for every page that is visible to the user, so he can use the text extraction tools without a delay.
Reimplemented in Okular::TextDocumentGenerator.
Definition at line 269 of file generator.cpp.
◆ generateTextPage()
void Generator::generateTextPage | ( | Page * | page | ) |
This method can be called to trigger the generation of a text page for the given page
.
The generation is done in the calling thread.
- See also
- TextPage
Definition at line 325 of file generator.cpp.
◆ hasFeature()
bool Generator::hasFeature | ( | GeneratorFeature | feature | ) | const |
Query for the specified feature
.
Definition at line 438 of file generator.cpp.
◆ image()
|
protectedvirtual |
Returns the image of the page as specified in the passed pixmap request
.
Must return a null image if the request was cancelled and the generator supports cancelling
- Warning
- this method may be executed in its own separated thread if the Threaded is enabled!
Definition at line 333 of file generator.cpp.
◆ isAllowed()
|
virtual |
Returns whether the given action
is allowed in the document.
- See also
- Okular::Permission
Definition at line 381 of file generator.cpp.
◆ layersModel()
|
virtual |
Returns the 'layers model' object of the document or NULL if layers model is not available.
- Since
- 0.24
Definition at line 542 of file generator.cpp.
◆ loadDocument()
Loads the document with the given fileName
and fills the pagesVector
with the parsed pages.
- Note
- If you implement the WithPassword variants you don't need to implement this one
- Returns
- true on success, false otherwise.
Definition at line 193 of file generator.cpp.
◆ loadDocumentFromData()
|
virtual |
Loads the document from the raw data fileData
and fills the pagesVector
with the parsed pages.
- Note
- If you implement the WithPassword variants you don't need to implement this one
- the Generator has to have the feature ReadRawData enabled
- Returns
- true on success, false otherwise.
Definition at line 201 of file generator.cpp.
◆ loadDocumentFromDataWithPassword()
|
virtual |
Loads the document from the raw data fileData
and password
and fills the pagesVector
with the parsed pages.
- Note
- Do not implement this if your format doesn't support passwords, it'll cleanly call loadDocumentFromData()
- the Generator has to have the feature ReadRawData enabled
- Since
- 0.20 (KDE 4.14)
- Returns
- a LoadResult defining the result of the operation
Definition at line 211 of file generator.cpp.
◆ loadDocumentWithPassword()
|
virtual |
Loads the document with the given fileName
and password
and fills the pagesVector
with the parsed pages.
- Note
- Do not implement this if your format doesn't support passwords, it'll cleanly call loadDocument()
- Since
- 0.20 (KDE 4.14)
- Returns
- a LoadResult defining the result of the operation
Reimplemented in Okular::TextDocumentGenerator.
Definition at line 206 of file generator.cpp.
◆ metaData()
This method returns the meta data of the given key
with the given option
of the document.
Definition at line 413 of file generator.cpp.
◆ notice
|
signal |
This signal should be emitted whenever the user should be noticed.
- Parameters
-
message The message which should be shown to the user. duration The time that the message should be shown to the user.
◆ opaqueAction()
|
virtual |
Calls the backend to execute a BackendOpaqueAction action
and returns BackendOpaqueAction result.
Definition at line 404 of file generator.cpp.
◆ pageSizeChanged()
This method is called when the page size has been changed by the user.
Definition at line 395 of file generator.cpp.
◆ pageSizes()
|
virtual |
Returns the list of supported page sizes.
Definition at line 390 of file generator.cpp.
◆ pagesSizeMetric()
|
virtual |
This method returns the metric of the page size.
Default is None.
Definition at line 376 of file generator.cpp.
◆ print()
|
virtual |
This method is called to print the document to the given printer
.
Reimplemented in Okular::TextDocumentGenerator.
Definition at line 399 of file generator.cpp.
◆ requestFontData()
|
protectedvirtual |
Gets the font data for the given font.
- Since
- 0.8 (old signature)
- 22.04 (new signature)
Definition at line 525 of file generator.cpp.
◆ rotationChanged()
This method is called when the orientation has been changed by the user.
Definition at line 386 of file generator.cpp.
◆ setDPI()
void Generator::setDPI | ( | const QSizeF | dpi | ) |
Update DPI of the generator.
- Since
- 0.19 (old signature)
- 22.04 (new signature)
Definition at line 530 of file generator.cpp.
◆ setFeature()
|
protected |
Toggle the feature
.
Definition at line 491 of file generator.cpp.
◆ sign()
|
virtual |
Definition at line 259 of file generator.cpp.
◆ signalPartialPixmapRequest
|
protectedslot |
This method can be called to trigger a partial pixmap update for the given request Make sure you call it in a way it's executed in the main thread.
- Since
- 1.3
Definition at line 464 of file generator.cpp.
◆ signalPixmapRequestDone()
|
protected |
This method must be called when the pixmap request triggered by generatePixmap() has been finished.
Definition at line 444 of file generator.cpp.
◆ signalTextGenerationDone()
This method must be called when a text generation has been finished.
Definition at line 454 of file generator.cpp.
◆ swapBackingFile()
|
virtual |
Changes the path of the file we are reading from.
The new path must point to a copy of the same document.
- Note
- the Generator has to have the feature SwapBackingFile enabled
- Since
- 1.3
Definition at line 216 of file generator.cpp.
◆ textPage()
|
protectedvirtual |
Returns the text page for the given request
.
Must return a null pointer if the request was cancelled and the generator supports cancelling
- Warning
- this method may be executed in its own separated thread if the Threaded is enabled!
- Since
- 1.4
Reimplemented in Okular::TextDocumentGenerator.
Definition at line 339 of file generator.cpp.
◆ updatePageBoundingBox()
|
protected |
Set the bounding box of a page after the page has already been handed to the Document.
Call this instead of Page::setBoundingBox() to ensure that all observers are notified.
- Since
- 0.7 (KDE 4.1)
Definition at line 517 of file generator.cpp.
◆ userMutex()
|
protected |
Return the pointer to a mutex the generator can use freely.
Definition at line 511 of file generator.cpp.
◆ walletDataForFile()
|
virtual |
This method is called to know which wallet data should be used for the given file name.
Unless you have very special requirements to where wallet data should be stored you don't need to reimplement this method.
Definition at line 429 of file generator.cpp.
◆ warning
|
signal |
This signal should be emitted whenever the user should be warned.
- Parameters
-
message The message which should be shown to the user. duration The time that the message should be shown to the user.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:55:12 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.