QCA::SecureLayer
#include <QtCrypto>
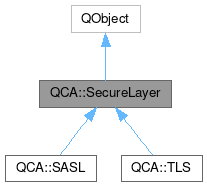
Signals | |
void | closed () |
void | error () |
void | readyRead () |
void | readyReadOutgoing () |
Public Member Functions | |
SecureLayer (QObject *parent=nullptr) | |
virtual int | bytesAvailable () const =0 |
virtual int | bytesOutgoingAvailable () const =0 |
virtual void | close () |
virtual int | convertBytesWritten (qint64 encryptedBytes)=0 |
virtual bool | isClosable () const |
virtual QByteArray | read ()=0 |
virtual QByteArray | readOutgoing (int *plainBytes=nullptr)=0 |
virtual QByteArray | readUnprocessed () |
virtual void | write (const QByteArray &a)=0 |
virtual void | writeIncoming (const QByteArray &a)=0 |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Abstract interface to a security layer.
SecureLayer is normally used between an application and a potentially insecure network. It provides secure communications over that network.
The concept is that (after some initial setup), the application can write() some data to the SecureLayer implementation, and that data is encrypted (or otherwise protected, depending on the setup). The SecureLayer implementation then emits the readyReadOutgoing() signal, and the application uses readOutgoing() to retrieve the encrypted data from the SecureLayer implementation. The encrypted data is then sent out on the network.
When some encrypted data comes back from the network, the application does a writeIncoming() to the SecureLayer implementation. Some time later, the SecureLayer implementation may emit readyRead() to the application, which then read()s the decrypted data from the SecureLayer implementation.
Note that sometimes data is sent or received between the SecureLayer implementation and the network without any data being sent between the application and the SecureLayer implementation. This is a result of the initial negotiation activities (which require network traffic to agree a configuration to use) and other overheads associated with the secure link.
Definition at line 104 of file qca_securelayer.h.
Constructor & Destructor Documentation
◆ SecureLayer()
QCA::SecureLayer::SecureLayer | ( | QObject * | parent = nullptr | ) |
Constructor for an abstract secure communications layer.
- Parameters
-
parent the parent object for this object
Member Function Documentation
◆ bytesAvailable()
|
pure virtual |
◆ bytesOutgoingAvailable()
|
pure virtual |
Returns the number of bytes available to be readOutgoing() on the network side.
◆ close()
|
virtual |
Close the link.
Note that this may not be meaningful / possible for all implementations.
- See also
- isClosable() for a test that verifies if the link can be closed.
Reimplemented in QCA::TLS.
◆ closed
|
signal |
This signal is emitted when the SecureLayer connection is closed.
- Examples
- sslservtest.cpp, ssltest.cpp, and tlssocket.cpp.
◆ convertBytesWritten()
|
pure virtual |
◆ error
|
signal |
This signal is emitted when an error is detected.
You can determine the error type using errorCode().
- Examples
- saslclient.cpp, saslserver.cpp, sslservtest.cpp, ssltest.cpp, and tlssocket.cpp.
◆ isClosable()
|
virtual |
Returns true if the layer has a meaningful "close".
Reimplemented in QCA::TLS.
◆ read()
|
pure virtual |
This method reads decrypted (plain) data from the SecureLayer implementation.
You normally call this function on the application side after receiving the readyRead() signal.
◆ readOutgoing()
|
pure virtual |
This method provides encoded (typically encrypted) data.
You normally call this function to get data to write out to the network socket (e.g. using QTcpSocket::write()) after receiving the readyReadOutgoing() signal.
- Parameters
-
plainBytes the number of bytes that were read.
◆ readUnprocessed()
|
virtual |
This allows you to read data without having it decrypted first.
This is intended to be used for protocols that close off the connection and return to plain text transfer. You do not normally need to use this function.
Reimplemented in QCA::TLS.
◆ readyRead
|
signal |
This signal is emitted when SecureLayer has decrypted (application side) data ready to be read.
Typically you will connect this signal to a slot that reads the data (using read()).
- Examples
- saslclient.cpp, saslserver.cpp, sslservtest.cpp, ssltest.cpp, and tlssocket.cpp.
◆ readyReadOutgoing
|
signal |
This signal is emitted when SecureLayer has encrypted (network side) data ready to be read.
Typically you will connect this signal to a slot that reads the data (using readOutgoing()) and writes it to a network socket.
- Examples
- saslclient.cpp, saslserver.cpp, sslservtest.cpp, ssltest.cpp, and tlssocket.cpp.
◆ write()
|
pure virtual |
This method writes unencrypted (plain) data to the SecureLayer implementation.
You normally call this function on the application side.
- Parameters
-
a the source of the application-side data
◆ writeIncoming()
|
pure virtual |
This method accepts encoded (typically encrypted) data for processing.
You normally call this function using data read from the network socket (e.g. using QTcpSocket::readAll()) after receiving a signal that indicates that the socket has data to read.
- Parameters
-
a the ByteArray to take network-side data from
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:50:48 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.