KIO::FileJob
#include <KIO/FileJob>
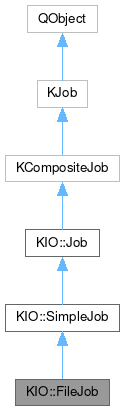
Signals | |
void | data (KIO::Job *job, const QByteArray &data) |
void | fileClosed (KIO::Job *job) |
void | mimeTypeFound (KIO::Job *job, const QString &mimeType) |
void | open (KIO::Job *job) |
void | position (KIO::Job *job, KIO::filesize_t offset) |
void | redirection (KIO::Job *job, const QUrl &url) |
void | truncated (KIO::Job *job, KIO::filesize_t length) |
void | written (KIO::Job *job, KIO::filesize_t written) |
![]() | |
void | connected (KIO::Job *job) |
![]() | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=QPair< QString, QString >(), const QPair< QString, QString > &field2=QPair< QString, QString >()) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &message) |
void | percentChanged (KJob *job, unsigned long percent) |
void | processedAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &message) |
Public Member Functions | |
void | close () |
void | read (KIO::filesize_t size) |
void | seek (KIO::filesize_t offset) |
KIO::filesize_t | size () |
void | truncate (KIO::filesize_t length) |
void | write (const QByteArray &data) |
![]() | |
bool | isRedirectionHandlingEnabled () const |
virtual void | putOnHold () |
void | setRedirectionHandlingEnabled (bool handle) |
const QUrl & | url () const |
![]() | |
void | addMetaData (const QMap< QString, QString > &values) |
void | addMetaData (const QString &key, const QString &value) |
QStringList | detailedErrorStrings (const QUrl *reqUrl=nullptr, int method=-1) const |
QString | errorString () const override |
void | mergeMetaData (const QMap< QString, QString > &values) |
MetaData | metaData () const |
MetaData | outgoingMetaData () const |
Job * | parentJob () const |
QString | queryMetaData (const QString &key) |
void | setMetaData (const KIO::MetaData &metaData) |
void | setParentJob (Job *parentJob) |
void | setUiDelegateExtension (JobUiDelegateExtension *extension) |
void | start () override |
JobUiDelegateExtension * | uiDelegateExtension () const |
![]() | |
KCompositeJob (QObject *parent=nullptr) | |
![]() | |
KJob (QObject *parent=nullptr) | |
Capabilities | capabilities () const |
qint64 | elapsedTime () const |
int | error () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isFinishedNotificationHidden () const |
bool | isStartedWithExec () const |
bool | isSuspended () const |
unsigned long | percent () const |
Q_SCRIPTABLE qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setFinishedNotificationHidden (bool hide=true) |
void | setUiDelegate (KJobUiDelegate *delegate) |
Q_SCRIPTABLE qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
KIOCORE_NO_EXPORT | FileJob (FileJobPrivate &dd) |
![]() | |
KIOCORE_NO_EXPORT | SimpleJob (SimpleJobPrivate &dd) |
bool | doKill () override |
bool | doResume () override |
bool | doSuspend () override |
![]() | |
Job (JobPrivate &dd) | |
bool | addSubjob (KJob *job) override |
bool | removeSubjob (KJob *job) override |
![]() | |
void | clearSubjobs () |
bool | hasSubjobs () const |
const QList< KJob * > & | subjobs () const |
![]() | |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | isFinished () const |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setProgressUnit (Unit unit) |
void | setTotalAmount (Unit unit, qulonglong amount) |
void | startElapsedTimer () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
typedef QFlags< Capability > | Capabilities |
enum | Capability |
enum | Unit |
![]() | |
objectName | |
![]() | |
void | slotError (int, const QString &) |
![]() | |
bool | kill (KJob::KillVerbosity verbosity=KJob::Quietly) |
bool | resume () |
bool | suspend () |
![]() | |
static void | removeOnHold () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
Bytes | |
Directories | |
Files | |
Items | |
Killable | |
NoCapabilities | |
Suspendable | |
UnitsCount | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | slotFinished () |
virtual void | slotMetaData (const KIO::MetaData &_metaData) |
virtual void | slotWarning (const QString &) |
![]() | |
virtual void | slotInfoMessage (KJob *job, const QString &message) |
virtual void | slotResult (KJob *job) |
![]() | |
JobPrivate *const | d_ptr |
Detailed Description
The file-job is an asynchronous version of normal file handling.
It allows block-wise reading and writing, and allows seeking and truncation. Results are returned through signals.
Should always be created using KIO::open(const QUrl&, QIODevice::OpenMode).
Constructor & Destructor Documentation
◆ ~FileJob()
|
override |
Definition at line 62 of file filejob.cpp.
◆ FileJob()
|
explicitprotected |
Definition at line 57 of file filejob.cpp.
Member Function Documentation
◆ close()
void FileJob::close | ( | ) |
Closes the file KIO worker.
The worker emits close() and result().
Definition at line 109 of file filejob.cpp.
◆ data
|
signal |
◆ fileClosed
|
signal |
Signals that the file is closed and will accept no more commands.
- Parameters
-
job the job that emitted this signal
- Since
- 5.79
◆ mimeTypeFound
MIME type determined.
- Parameters
-
job the job that emitted this signal mimeType the MIME type
- Since
- 5.78
◆ open
|
signal |
File is open, metadata has been determined and the file KIO worker is ready to receive commands.
- Parameters
-
job the job that emitted this signal
◆ position
|
signal |
The file has reached this position.
Emitted after seek().
- Parameters
-
job the job that emitted this signal offset the new position
◆ read()
void FileJob::read | ( | KIO::filesize_t | size | ) |
This function attempts to read up to size
bytes from the URL passed to KIO::open() and returns the bytes received via the data() signal.
The read operation commences at the current file offset, and the file offset is incremented by the number of bytes read, but this change in the offset does not result in the position() signal being emitted.
If the current file offset is at or past the end of file (i.e. EOD), no bytes are read, and the data() signal returns an empty QByteArray.
On error the data() signal is not emitted. To catch errors please connect to the result() signal.
- Parameters
-
size the requested amount of data to read
Definition at line 66 of file filejob.cpp.
◆ redirection
Signals the file is a redirection.
Follow this url manually to reach data
- Parameters
-
job the job that emitted this signal url the new URL
◆ seek()
void FileJob::seek | ( | KIO::filesize_t | offset | ) |
Seek.
The worker emits position() on successful seek to the specified offset
.
On error the position() signal is not emitted. To catch errors please connect to the result() signal.
- Parameters
-
offset the position from start to go to
Definition at line 87 of file filejob.cpp.
◆ size()
KIO::filesize_t FileJob::size | ( | ) |
◆ truncate()
void FileJob::truncate | ( | KIO::filesize_t | length | ) |
Truncate.
The worker emits truncated() on successful truncation to the specified length
.
On error the truncated() signal is not emitted. To catch errors please connect to the result() signal.
- Parameters
-
length the desired length to truncate to
- Since
- 5.66
Definition at line 98 of file filejob.cpp.
◆ truncated
|
signal |
The file has been truncated to this point.
Emitted after truncate().
- Parameters
-
job the job that emitted this signal length the new length of the file
- Since
- 5.66
◆ write()
void FileJob::write | ( | const QByteArray & | data | ) |
This function attempts to write all the bytes in data
to the URL passed to KIO::open() and returns the bytes written received via the written() signal.
The write operation commences at the current file offset, and the file offset is incremented by the number of bytes read, but this change in the offset does not result in the position() being emitted.
On error the written() signal is not emitted. To catch errors please connect to the result() signal.
- Parameters
-
data the data to write
Definition at line 77 of file filejob.cpp.
◆ written
|
signal |
written
bytes were written to the file.
Emitted after write().
- Parameters
-
job the job that emitted this signal written bytes written.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 4 2025 12:07:32 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.