KIO::Job
#include <KIO/Job>
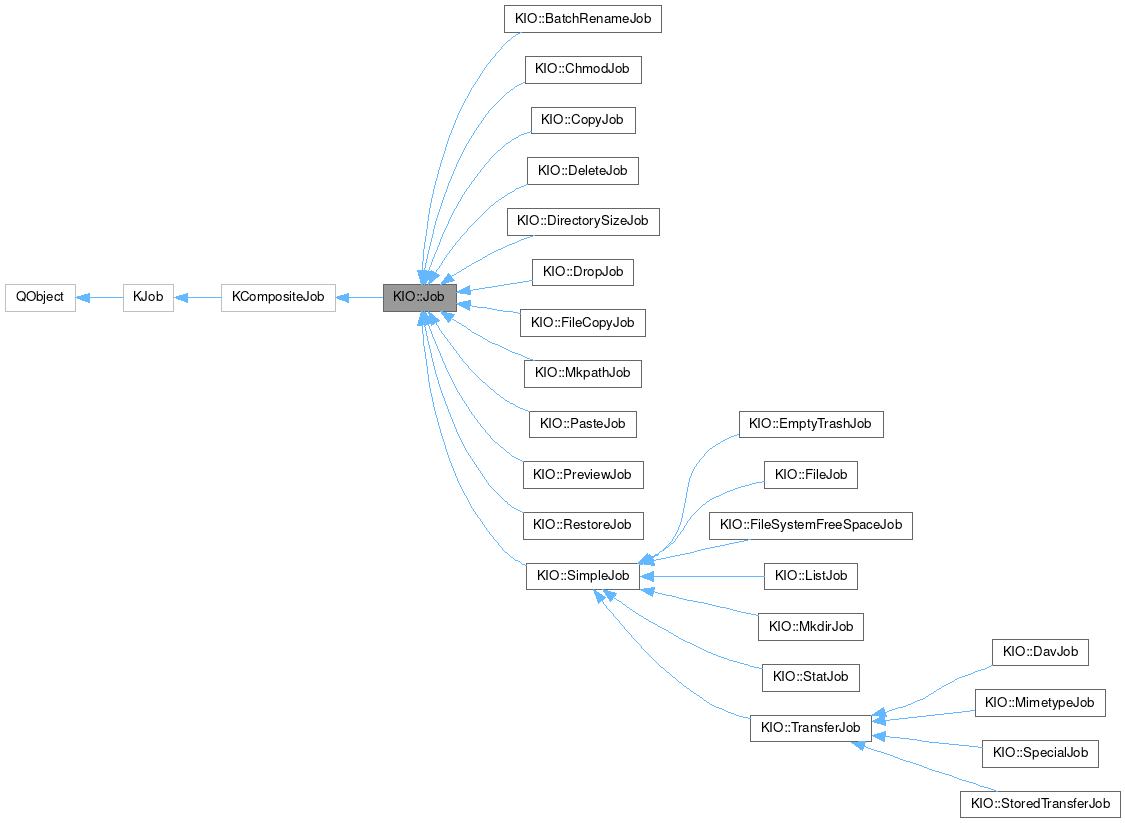
Signals | |
void | connected (KIO::Job *job) |
![]() | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=QPair< QString, QString >(), const QPair< QString, QString > &field2=QPair< QString, QString >()) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &message) |
void | percentChanged (KJob *job, unsigned long percent) |
void | processedAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &message) |
Public Member Functions | |
void | addMetaData (const QMap< QString, QString > &values) |
void | addMetaData (const QString &key, const QString &value) |
QStringList | detailedErrorStrings (const QUrl *reqUrl=nullptr, int method=-1) const |
QString | errorString () const override |
void | mergeMetaData (const QMap< QString, QString > &values) |
MetaData | metaData () const |
MetaData | outgoingMetaData () const |
Job * | parentJob () const |
QString | queryMetaData (const QString &key) |
void | setMetaData (const KIO::MetaData &metaData) |
void | setParentJob (Job *parentJob) |
void | setUiDelegateExtension (JobUiDelegateExtension *extension) |
void | start () override |
JobUiDelegateExtension * | uiDelegateExtension () const |
![]() | |
KCompositeJob (QObject *parent=nullptr) | |
![]() | |
KJob (QObject *parent=nullptr) | |
Capabilities | capabilities () const |
int | error () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isFinishedNotificationHidden () const |
bool | isStartedWithExec () const |
bool | isSuspended () const |
unsigned long | percent () const |
Q_SCRIPTABLE qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setFinishedNotificationHidden (bool hide=true) |
void | setUiDelegate (KJobUiDelegate *delegate) |
Q_SCRIPTABLE qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
Job (JobPrivate &dd) | |
bool | addSubjob (KJob *job) override |
bool | doKill () override |
bool | doResume () override |
bool | doSuspend () override |
bool | removeSubjob (KJob *job) override |
![]() | |
void | clearSubjobs () |
bool | hasSubjobs () const |
const QList< KJob * > & | subjobs () const |
![]() | |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | isFinished () const |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setProgressUnit (Unit unit) |
void | setTotalAmount (Unit unit, qulonglong amount) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
JobPrivate *const | d_ptr |
Additional Inherited Members | |
![]() | |
typedef QFlags< Capability > | Capabilities |
enum | Capability |
enum | Unit |
![]() | |
objectName | |
![]() | |
bool | kill (KJob::KillVerbosity verbosity=KJob::Quietly) |
bool | resume () |
bool | suspend () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
Bytes | |
Directories | |
Files | |
Items | |
Killable | |
NoCapabilities | |
Suspendable | |
UnitsCount | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | slotInfoMessage (KJob *job, const QString &message) |
virtual void | slotResult (KJob *job) |
Detailed Description
The base class for all jobs.
For all jobs created in an application, the code looks like
(other connects, specific to the job)
And slotResult is usually at least:
- See also
- KIO::Scheduler
Definition at line 44 of file job_base.h.
Constructor & Destructor Documentation
◆ Job() [1/2]
◆ Job() [2/2]
◆ ~Job()
Member Function Documentation
◆ addMetaData() [1/2]
Add key/value pairs to the meta data that is sent to the worker.
If a certain key already existed, it will be overridden.
- Parameters
-
values the meta data to add
- See also
- setMetaData()
- mergeMetaData()
◆ addMetaData() [2/2]
Add key/value pair to the meta data that is sent to the worker.
- Parameters
-
key the key of the meta data value the value of the meta data
- See also
- setMetaData()
- mergeMetaData()
◆ addSubjob()
|
overrideprotectedvirtual |
Add a job that has to be finished before a result is emitted.
This has obviously to be called before the finish signal is emitted by the worker.
- Parameters
-
job the subjob to add
Reimplemented from KCompositeJob.
◆ connected
|
signal |
Emitted when the worker successfully connected to the host.
There is no guarantee the worker will send this, and this is currently unused (in the applications).
- Parameters
-
job the job that emitted this signal
◆ detailedErrorStrings()
QStringList KIO::Job::detailedErrorStrings | ( | const QUrl * | reqUrl = nullptr, |
int | method = -1 ) const |
Converts an error code and a non-i18n error message into i18n strings suitable for presentation in a detailed error message box.
- Parameters
-
reqUrl the request URL that generated this error message method the method that generated this error message (unimplemented)
- Returns
- the following strings: title, error + description, causes+solutions
Definition at line 271 of file job_error.cpp.
◆ doKill()
|
overrideprotectedvirtual |
Abort this job.
This kills all subjobs and deletes the job.
Reimplemented from KJob.
Reimplemented in KIO::SimpleJob.
◆ doResume()
|
overrideprotectedvirtual |
Resume this job.
- See also
- suspend
Reimplemented from KJob.
Reimplemented in KIO::SimpleJob, and KIO::TransferJob.
◆ doSuspend()
|
overrideprotectedvirtual |
◆ errorString()
|
overridevirtual |
Converts an error code and a non-i18n error message into an error message in the current language.
The low level (non-i18n) error message (usually a url) is put into the translated error message using %1.
Example for errid == ERR_CANNOT_OPEN_FOR_READING:
Use this to display the error yourself, but for a dialog box use uiDelegate()->showErrorMessage(). Do not call it if error() is not 0.
- Returns
- the error message and if there is no error, a message telling the user that the app is broken, so check with error() whether there is an error
Reimplemented from KJob.
Definition at line 26 of file job_error.cpp.
◆ mergeMetaData()
Add key/value pairs to the meta data that is sent to the worker.
If a certain key already existed, it will remain unchanged.
- Parameters
-
values the meta data to merge
- See also
- setMetaData()
- addMetaData()
◆ metaData()
MetaData Job::metaData | ( | ) | const |
◆ outgoingMetaData()
MetaData Job::outgoingMetaData | ( | ) | const |
◆ parentJob()
Job * Job::parentJob | ( | ) | const |
Returns the parent job, if there is one.
- Returns
- the parent job, or
nullptr
if there is none
- See also
- setParentJob
◆ queryMetaData()
◆ removeSubjob()
|
overrideprotectedvirtual |
Mark a sub job as being done.
Note that this does not terminate the parent job, even if job
is the last subjob. emitResult must be called to indicate that the job is complete.
- Parameters
-
job the subjob to remove
Reimplemented from KCompositeJob.
◆ setMetaData()
void Job::setMetaData | ( | const KIO::MetaData & | metaData | ) |
Set meta data to be sent to the worker, replacing existing meta data.
- Parameters
-
metaData the meta data to set
- See also
- addMetaData()
- mergeMetaData()
◆ setParentJob()
void Job::setParentJob | ( | Job * | parentJob | ) |
Set the parent Job.
One example use of this is when FileCopyJob calls RenameDialog::open, it must pass the correct progress ID of the parent CopyJob (to hide the progress dialog). You can set the parent job only once. By default a job does not have a parent job.
- Parameters
-
parentJob the new parent job
◆ setUiDelegateExtension()
void Job::setUiDelegateExtension | ( | JobUiDelegateExtension * | extension | ) |
Sets the UI delegate extension to be used by this job.
The default UI delegate extension is KIO::defaultJobUiDelegateExtension()
◆ start()
|
inlineoverridevirtual |
Implements KJob.
Definition at line 55 of file job_base.h.
◆ uiDelegateExtension()
JobUiDelegateExtension * Job::uiDelegateExtension | ( | ) | const |
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 230 of file job_base.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 17 2024 11:53:30 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.