KTextEditor::Application
#include <KTextEditor/Application>
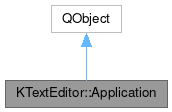
Signals | |
void | documentCreated (KTextEditor::Document *document) |
void | documentDeleted (KTextEditor::Document *document) |
void | documentWillBeDeleted (KTextEditor::Document *document) |
void | pluginCreated (const QString &name, KTextEditor::Plugin *plugin) |
void | pluginDeleted (const QString &name, KTextEditor::Plugin *plugin) |
Public Member Functions | |
Application (QObject *parent) | |
~Application () override | |
KTextEditor::MainWindow * | activeMainWindow () |
bool | closeDocument (KTextEditor::Document *document) |
bool | closeDocuments (const QList< KTextEditor::Document * > &documents) |
QList< KTextEditor::Document * > | documents () |
KTextEditor::Document * | findUrl (const QUrl &url) |
QList< KTextEditor::MainWindow * > | mainWindows () |
KTextEditor::Document * | openUrl (const QUrl &url, const QString &encoding=QString()) |
KTextEditor::Plugin * | plugin (const QString &name) |
bool | quit () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class allows the application that embeds the KTextEditor component to allow it access to application wide information and interactions.
For example the component can get the current active main window of the application.
The application must pass a pointer to the Application object to the setApplication method of the global editor instance and ensure that this object stays valid for the complete lifetime of the editor.
It must not reimplement this class but construct an instance and pass a pointer to a QObject that has the required slots to receive the requests.
KTextEditor::Editor::instance()->application() will always return a non-nullptr object to avoid the need for nullptr checks before calling the API.
The same holds for activeMainWindow(), even if no main window is around, you will get a non-nullptr interface object that allows to call the functions of the MainWindow without needs for a nullptr check around it in the client code.
Definition at line 43 of file application.h.
Constructor & Destructor Documentation
◆ Application()
KTextEditor::Application::Application | ( | QObject * | parent | ) |
Construct an Application wrapper object.
The passed parent is both the parent of this QObject and the receiver of all interface calls via invokeMethod.
- Parameters
-
parent object the calls are relayed to
Definition at line 15 of file application.cpp.
◆ ~Application()
|
overridedefault |
Virtual Destructor.
Member Function Documentation
◆ activeMainWindow()
KTextEditor::MainWindow * KTextEditor::Application::activeMainWindow | ( | ) |
Accessor to the active main window.
- Returns
- a pointer to the active mainwindow, even if no main window is active you will get a non-nullptr dummy interface that allows you to call interface functions without the need for null checks
Definition at line 40 of file application.cpp.
◆ closeDocument()
bool KTextEditor::Application::closeDocument | ( | KTextEditor::Document * | document | ) |
Close the given document
.
If the document is modified, user will be asked if he wants that.
- Parameters
-
document the document to be closed
- Returns
- true on success, otherwise false
Definition at line 79 of file application.cpp.
◆ closeDocuments()
bool KTextEditor::Application::closeDocuments | ( | const QList< KTextEditor::Document * > & | documents | ) |
Close a list of documents.
If any of them are modified, user will be asked if he wants that. Use this, if you want to close multiple documents at once, as the application might be able to group the "do you really want that" dialogs into one.
- Parameters
-
documents list of documents to be closed
- Returns
- true on success, otherwise false
Definition at line 87 of file application.cpp.
◆ documentCreated
|
signal |
This signal is emitted when the document
was created.
- Parameters
-
document document that was created
◆ documentDeleted
|
signal |
This signal is emitted when the document
has been deleted.
- Warning
- Do not access the data referenced by the pointer, it is already invalid. Use the pointer only to remove mappings in hash or maps
- Parameters
-
document document that is deleted
◆ documents()
QList< KTextEditor::Document * > KTextEditor::Application::documents | ( | ) |
Get a list of all documents that are managed by the application.
This might contain less documents than the editor has in his documents () list.
- Returns
- all documents the application manages, might be empty!
Definition at line 50 of file application.cpp.
◆ documentWillBeDeleted
|
signal |
This signal is emitted before a document
which should be closed is deleted The document is still accessible and usable, but it will be deleted after this signal was send.
- Parameters
-
document document that will be deleted
◆ findUrl()
KTextEditor::Document * KTextEditor::Application::findUrl | ( | const QUrl & | url | ) |
Get the document with the URL url
.
if multiple documents match the searched url, return the first found one...
- Parameters
-
url the document's URL
- Returns
- the document with the given
url
or nullptr, if none found
Definition at line 58 of file application.cpp.
◆ mainWindows()
QList< KTextEditor::MainWindow * > KTextEditor::Application::mainWindows | ( | ) |
Get a list of all main windows.
- Returns
- all main windows, might be empty!
Definition at line 32 of file application.cpp.
◆ openUrl()
KTextEditor::Document * KTextEditor::Application::openUrl | ( | const QUrl & | url, |
const QString & | encoding = QString() ) |
Open the document url
with the given encoding
.
if the url is empty, a new empty document will be created
- Parameters
-
url the document's url encoding the preferred encoding. If encoding is QString() the encoding will be guessed or the default encoding will be used.
- Returns
- a pointer to the created document
Definition at line 66 of file application.cpp.
◆ plugin()
KTextEditor::Plugin * KTextEditor::Application::plugin | ( | const QString & | name | ) |
Get a plugin for the plugin with with identifier name
.
- Parameters
-
name the plugin's name
- Returns
- pointer to the plugin if a plugin with
name
is loaded, otherwise nullptr
Definition at line 95 of file application.cpp.
◆ pluginCreated
|
signal |
This signal is emitted when an Plugin was loaded.
- Parameters
-
name name of plugin plugin the new plugin
◆ pluginDeleted
|
signal |
This signal is emitted when an Plugin got deleted.
- Parameters
-
name name of plugin plugin the deleted plugin
- Warning
- Do not access the data referenced by the pointer, it is already invalid. Use the pointer only to remove mappings in hash or maps
◆ quit()
bool KTextEditor::Application::quit | ( | ) |
Ask app to quit.
The app might interact with the user and decide that quitting is not possible and return false.
- Returns
- true if the app could quit
Definition at line 23 of file application.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 17 2024 11:56:22 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.