KTextEditor::Document
#include <KTextEditor/Document>
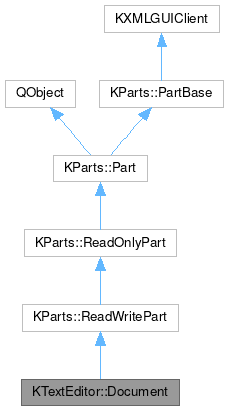
Classes | |
class | EditingTransaction |
Signals | |
void | configChanged (KTextEditor::Document *document) |
![]() | |
void | sigQueryClose (bool *handled, bool *abortClosing) |
![]() | |
void | canceled (const QString &errMsg) |
void | completed () |
void | completedWithPendingAction () |
void | started (KIO::Job *job) |
void | urlChanged (const QUrl &url) |
![]() | |
void | setStatusBarText (const QString &text) |
void | setWindowCaption (const QString &caption) |
Public Member Functions | |
~Document () override | |
Printing | |
virtual bool | print ()=0 |
virtual void | printPreview ()=0 |
Showing Interactive Notifications | |
virtual bool | postMessage (Message *message)=0 |
Session Configuration | |
virtual void | readSessionConfig (const KConfigGroup &config, const QSet< QString > &flags=QSet< QString >())=0 |
virtual void | writeSessionConfig (KConfigGroup &config, const QSet< QString > &flags=QSet< QString >())=0 |
Crash Recovery | |
virtual bool | isDataRecoveryAvailable () const =0 |
virtual void | recoverData ()=0 |
virtual void | discardDataRecovery ()=0 |
Config | |
virtual QStringList | configKeys () const =0 |
virtual QVariant | configValue (const QString &key)=0 |
virtual void | setConfigValue (const QString &key, const QVariant &value)=0 |
Annotation Interface | |
virtual void | setAnnotationModel (AnnotationModel *model)=0 |
virtual AnnotationModel * | annotationModel () const =0 |
![]() | |
ReadWritePart (QObject *parent=nullptr, const KPluginMetaData &data={}) | |
bool | closeUrl () override |
virtual bool | closeUrl (bool promptToSave) |
bool | isModified () const |
bool | isReadWrite () const |
virtual bool | queryClose () |
virtual bool | saveAs (const QUrl &url) |
virtual void | setModified (bool modified) |
virtual void | setReadWrite (bool readwrite=true) |
![]() | |
ReadOnlyPart (QObject *parent=nullptr, const KPluginMetaData &data={}) | |
OpenUrlArguments | arguments () const |
bool | closeStream () |
bool | isProgressInfoEnabled () const |
NavigationExtension * | navigationExtension () const |
bool | openStream (const QString &mimeType, const QUrl &url) |
void | setArguments (const OpenUrlArguments &arguments) |
void | setProgressInfoEnabled (bool show) |
QUrl | url () const |
bool | writeStream (const QByteArray &data) |
![]() | |
Part (QObject *parent=nullptr, const KPluginMetaData &data={}) | |
virtual Part * | hitTest (QWidget *widget, const QPoint &globalPos) |
PartManager * | manager () const |
KPluginMetaData | metaData () const |
void | setAutoDeletePart (bool autoDeletePart) |
void | setAutoDeleteWidget (bool autoDeleteWidget) |
virtual void | setManager (PartManager *manager) |
virtual QWidget * | widget () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
void | setPartObject (QObject *object) |
Protected Member Functions | |
Document (DocumentPrivate *impl, const KPluginMetaData &data, QObject *parent) | |
![]() | |
virtual bool | saveFile ()=0 |
virtual bool | saveToUrl () |
![]() | |
void | abortLoad () |
virtual bool | doCloseStream () |
virtual bool | doOpenStream (const QString &mimeType) |
virtual bool | doWriteStream (const QByteArray &data) |
void | guiActivateEvent (GUIActivateEvent *event) override |
QString | localFilePath () const |
virtual bool | openFile () |
void | setLocalFilePath (const QString &localFilePath) |
void | setUrl (const QUrl &url) |
![]() | |
void | customEvent (QEvent *event) override |
QWidget * | hostContainer (const QString &containerName) |
virtual void | partActivateEvent (PartActivateEvent *event) |
virtual void | setWidget (QWidget *widget) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Modification Interface | |
enum | ModifiedOnDiskReason { OnDiskUnmodified = 0 , OnDiskModified = 1 , OnDiskCreated = 2 , OnDiskDeleted = 3 } |
virtual void | setModifiedOnDisk (ModifiedOnDiskReason reason)=0 |
virtual void | setModifiedOnDiskWarning (bool on)=0 |
void | modifiedOnDisk (KTextEditor::Document *document, bool isModified, KTextEditor::Document::ModifiedOnDiskReason reason) |
Mark Interface | |
enum | MarkTypes { markType01 = 0x1 , markType02 = 0x2 , markType03 = 0x4 , markType04 = 0x8 , markType05 = 0x10 , markType06 = 0x20 , markType07 = 0x40 , markType08 = 0x80 , markType09 = 0x100 , markType10 = 0x200 , markType11 = 0x400 , markType12 = 0x800 , markType13 = 0x1000 , markType14 = 0x2000 , markType15 = 0x4000 , markType16 = 0x8000 , markType17 = 0x10000 , markType18 = 0x20000 , markType19 = 0x40000 , markType20 = 0x80000 , markType21 = 0x100000 , markType22 = 0x200000 , markType23 = 0x400000 , markType24 = 0x800000 , markType25 = 0x1000000 , markType26 = 0x2000000 , markType27 = 0x4000000 , markType28 = 0x8000000 , markType29 = 0x10000000 , markType30 = 0x20000000 , markType31 = 0x40000000 , markType32 = 0x80000000 , Bookmark = markType01 , BreakpointActive = markType02 , BreakpointReached = markType03 , BreakpointDisabled = markType04 , Execution = markType05 , Warning = markType06 , Error = markType07 , SearchMatch = markType32 } |
enum | MarkChangeAction { MarkAdded = 0 , MarkRemoved = 1 } |
virtual uint | mark (int line)=0 |
virtual void | setMark (int line, uint markType)=0 |
virtual void | clearMark (int line)=0 |
virtual void | addMark (int line, uint markType)=0 |
virtual void | removeMark (int line, uint markType)=0 |
virtual const QHash< int, KTextEditor::Mark * > & | marks ()=0 |
virtual void | clearMarks ()=0 |
virtual void | setMarkDescription (MarkTypes mark, const QString &text)=0 |
virtual QString | markDescription (MarkTypes mark) const =0 |
virtual void | setEditableMarks (uint markMask)=0 |
virtual uint | editableMarks () const =0 |
virtual void | setMarkIcon (MarkTypes markType, const QIcon &icon)=0 |
virtual QIcon | markIcon (MarkTypes markType) const =0 |
void | marksChanged (KTextEditor::Document *document) |
void | markChanged (KTextEditor::Document *document, KTextEditor::Mark mark, KTextEditor::Document::MarkChangeAction action) |
void | markToolTipRequested (KTextEditor::Document *document, KTextEditor::Mark mark, QPoint position, bool &handled) |
void | markContextMenuRequested (KTextEditor::Document *document, KTextEditor::Mark mark, QPoint pos, bool &handled) |
void | markClicked (KTextEditor::Document *document, KTextEditor::Mark mark, bool &handled) |
static int | reservedMarkersCount () |
Manage Views of this Document | |
virtual View * | createView (QWidget *parent, KTextEditor::MainWindow *mainWindow=nullptr)=0 |
virtual QList< View * > | views () const =0 |
void | viewCreated (KTextEditor::Document *document, KTextEditor::View *view) |
General Information about this Document | |
virtual QString | documentName () const =0 |
virtual QString | mimeType ()=0 |
virtual QByteArray | checksum () const =0 |
virtual bool | setEncoding (const QString &encoding)=0 |
virtual QString | encoding () const =0 |
void | documentNameChanged (KTextEditor::Document *document) |
void | documentUrlChanged (KTextEditor::Document *document) |
void | modifiedChanged (KTextEditor::Document *document) |
void | readWriteChanged (KTextEditor::Document *document) |
File Loading and Saving | |
All this actions cause user interaction in some cases. | |
virtual bool | documentReload ()=0 |
virtual bool | documentSave ()=0 |
virtual bool | documentSaveAs ()=0 |
bool | openingError () const |
void | documentSavedOrUploaded (KTextEditor::Document *document, bool saveAs) |
void | aboutToClose (KTextEditor::Document *document) |
void | aboutToReload (KTextEditor::Document *document) |
void | reloaded (KTextEditor::Document *document) |
void | aboutToSave (KTextEditor::Document *document) |
Text Manipulation | |
virtual bool | isEditingTransactionRunning () const =0 |
virtual QString | text () const =0 |
virtual QString | text (Range range, bool block=false) const =0 |
virtual QChar | characterAt (KTextEditor::Cursor position) const =0 |
virtual QString | wordAt (KTextEditor::Cursor cursor) const =0 |
virtual KTextEditor::Range | wordRangeAt (KTextEditor::Cursor cursor) const =0 |
virtual bool | isValidTextPosition (KTextEditor::Cursor cursor) const =0 |
virtual QStringList | textLines (Range range, bool block=false) const =0 |
virtual QString | line (int line) const =0 |
virtual int | lines () const =0 |
virtual bool | isLineModified (int line) const =0 |
virtual bool | isLineSaved (int line) const =0 |
virtual bool | isLineTouched (int line) const =0 |
virtual Cursor | documentEnd () const =0 |
Range | documentRange () const |
virtual qsizetype | totalCharacters () const =0 |
virtual bool | isEmpty () const |
virtual int | lineLength (int line) const =0 |
Cursor | endOfLine (int line) const |
virtual bool | setText (const QString &text)=0 |
virtual bool | setText (const QStringList &text)=0 |
virtual bool | clear ()=0 |
virtual bool | insertText (KTextEditor::Cursor position, const QString &text, bool block=false)=0 |
virtual bool | insertText (KTextEditor::Cursor position, const QStringList &text, bool block=false)=0 |
virtual bool | replaceText (Range range, const QString &text, bool block=false) |
virtual bool | replaceText (Range range, const QStringList &text, bool block=false) |
virtual bool | removeText (Range range, bool block=false)=0 |
virtual bool | insertLine (int line, const QString &text)=0 |
virtual bool | insertLines (int line, const QStringList &text)=0 |
virtual bool | removeLine (int line)=0 |
QList< KTextEditor::Range > | searchText (KTextEditor::Range range, const QString &pattern, const SearchOptions options=Default) const |
virtual qsizetype | cursorToOffset (KTextEditor::Cursor c) const =0 |
virtual KTextEditor::Cursor | offsetToCursor (qsizetype offset) const =0 |
void | editingStarted (KTextEditor::Document *document) |
void | editingFinished (KTextEditor::Document *document) |
void | lineWrapped (KTextEditor::Document *document, KTextEditor::Cursor position) |
void | lineUnwrapped (KTextEditor::Document *document, int line) |
void | textInserted (KTextEditor::Document *document, KTextEditor::Cursor position, const QString &text) |
void | textRemoved (KTextEditor::Document *document, KTextEditor::Range range, const QString &text) |
void | textChanged (KTextEditor::Document *document) |
Highlighting and Related Information | |
virtual KSyntaxHighlighting::Theme::TextStyle | defaultStyleAt (KTextEditor::Cursor position) const =0 |
virtual QString | mode () const =0 |
virtual QString | highlightingMode () const =0 |
virtual QStringList | embeddedHighlightingModes () const =0 |
virtual QString | highlightingModeAt (KTextEditor::Cursor position)=0 |
virtual QStringList | modes () const =0 |
virtual QStringList | highlightingModes () const =0 |
virtual bool | setMode (const QString &name)=0 |
virtual bool | setHighlightingMode (const QString &name)=0 |
virtual QString | highlightingModeSection (int index) const =0 |
virtual QString | modeSection (int index) const =0 |
void | modeChanged (KTextEditor::Document *document) |
void | highlightingModeChanged (KTextEditor::Document *document) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
virtual bool | save () |
void | setModified () |
bool | waitSaveComplete () |
![]() | |
virtual bool | openUrl (const QUrl &url) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
void | slotWidgetDestroyed () |
Detailed Description
A KParts derived class representing a text document.
Topics:
- Introduction
- Text Manipulation
- Document Views
- Read-Only Mode
- Notifications in Documents and Views
- Crash Recovery for Documents
- Document Moving Interface
- Document Config
- External modification extension interface for the Document.
- doc_marktext
- Annotation Interface
Introduction
The Document class represents a pure text document providing methods to modify the content and create views. A document can have any number of views, each view representing the same content, i.e. all views are synchronized. Support for text selection is handled by a View and text format attributes by the Attribute class.
To load a document call KParts::ReadOnlyPart::openUrl(). To reload a document from a file call documentReload(), to save the document call documentSave() or documentSaveAs(). Whenever the modified state of the document changes the signal modifiedChanged() is emitted. Check the modified state with KParts::ReadWritePart::isModified(). Further signals are documentUrlChanged(). The encoding can be specified with setEncoding(), however this will only take effect on file reload and file save.
Text Manipulation
Get the whole content with text() and set new content with setText(). Call insertText() or insertLine() to insert new text or removeText() and removeLine() to remove content. Whenever the document's content changed the signal textChanged() is emitted. Additional signals are textInserted() and textRemoved(). Note, that the first line in the document is line 0.
A Document provides full undo/redo history. Text manipulation actions can be grouped together to one undo/redo action by using an the class EditingTransaction. You can stack multiple EditingTransactions. Internally, the Document has a reference counter. If this reference counter is increased the first time (by creating an instance of EditingTransaction), the signal editingStarted() is emitted. Only when the internal reference counter reaches zero again, the signal editingFinished() and optionally the signal textChanged() are emitted. Whether an editing transaction is currently active can be checked by calling isEditingTransactionRunning().
- Note
- The signal editingFinished() is always emitted when the last instance of EditingTransaction is destroyed. Contrary, the signal textChanged() is emitted only if text changed. Hence, textChanged() is more accurate with respect to changes in the Document.
Every text editing transaction is also available through the signals lineWrapped(), lineUnwrapped(), textInserted() and textRemoved(). However, these signals should be used with care. Please be aware of the following warning:
- Warning
- Never change the Document's contents when edit actions are active, i.e. in between of (foreign) editing transactions. In case you violate this, the currently active edit action may perform edits that lead to undefined behavior.
Document Views
A View displays the document's content. As already mentioned, a document can have any number of views, all synchronized. Get a list of all views with views(). Create a new view with createView(). Every time a new view is created the signal viewCreated() is emitted.
Read-Only Mode
A Document may be in read-only mode, for instance due to missing file permissions. The read-only mode can be checked with isReadWrite(). Further, the signal readWriteChanged() is emitted whenever the state changes either to read-only mode or to read/write mode. The read-only mode can be controlled with setReadWrite().
Notifications in Documents and Views
A Document has the ability to show a Message to the user in a View. The Message then is shown either the specified View if Message::setView() was called, or in all Views of the Document.
To post a message just create a new Message and send it with postMessage(). Further information is available in the API documentation of Message.
- See also
- Message
Crash Recovery for Documents
When the system or the application using the editor component crashed with unsaved changes in the Document, the View notifies the user about the lost data and asks, whether the data should be recovered.
This Document gives you control over the data recovery process. Use isDataRecoveryAvailable() to check for lost data. If you do not want the editor component to handle the data recovery process automatically, you can either trigger the data recovery by calling recoverData() or discard it through discardDataRecovery().
Document Moving Interface
Document Moving Interface allows you to create MovingRange and MovingCursor. A Moving Range or Cursor is a special type of range/cursor because it automatically moves on text insertion or removal. Additionally, one can use the moving ranges to change the color of a particular word or give it a different attribute such as bold. Use newMovingCursor() to create a new moving cursor and newMovingRange() to create a new moving range.
Upon destruction or reload, a document will remove all its moving ranges. You can connect to aboutToDeleteMovingInterfaceContent() and aboutToInvalidateMovingInterfaceContent() signals to know when that is going to happen and update the cached ranges accordingly.
Document Config
Config provides methods to access and modify the low level config information for a given Document. KTextEditor::Document has support for the following config keys:
- backup-on-save-local [bool], enable/disable backup when saving local files
- backup-on-save-remote [bool], enable/disable backup when saving remote files
- backup-on-save-suffix [string], set the suffix for file backups, e.g. "~"
- backup-on-save-prefix [string], set the prefix for file backups, e.g. "."
- replace-tabs [bool], whether to replace tabs
- indent-pasted-text [bool], whether to indent pasted text
- tab-width [int], read/set the width for tabs
- indent-width [int], read/set the indentation width
- on-the-fly-spellcheck [bool], enable/disable on the fly spellcheck
External modification extension interface for the Document.
The class ModificationInterface provides methods to handle modifications of all opened files caused by external programs. Whenever the modified-on-disk state changes the signal modifiedOnDisk() is emitted along with a ModifiedOnDiskReason. Set the state by calling setModifiedOnDisk(). Whether the Editor should show warning dialogs to inform the user about external modified files can be controlled with setModifiedOnDiskWarning(). The slot modifiedOnDisk() is called to ask the user what to do whenever a file was modified.
doc_marktext
The Mark Interface provides methods to enable and disable marks in a Document, a marked line can be visualized for example with a shaded background color and/or a pixmap in the iconborder of the Document's View. There are a number of predefined mark types, specified in reservedMarkersCount(). Additionally it is possible to add custom marks and set custom pixmaps.
Get all marks in the document by calling marks(). Use clearMarks() to remove all marks in the entire document. A single mark can be retrieved with mark(). To remove all marks from a line call clearMark(). To add and remove marks from a given line use addMark() and removeMark(). It is also possible to replace all marks with setMark(), i.e. setMark() is the same as a call of clearMark() followed by addMark(). The signals marksChanged() and markChanged() are emitted whenever a line's marks changed.
- Attention
- A mark type is represented as an uint. An uint can have several mark types combined (see above: logical OR). That means for all functions/signals with an uint parameter, e.g. setMark(), removeMark(), etc, the uint may contain multiple marks, i.e. you can add and remove multiple marks simultaneously.
All marks that should be editable by the user can be specified with a mark mask via setEditableMarks(). To set a description and pixmap of a mark type call setMarkDescription() and setMarkPixmap().
Annotation Interface
The Annotation Interface is designed to provide line annotation information for a document. This interface provides means to associate a document with a annotation model, which provides some annotation information for each line in the document.
Setting a model for a Document makes the model data available for all views. If you only want to provide annotations in exactly one view, you can use the AnnotationViewInterface directly. See the AnnotationViewInterface for further details. To summarize, the two use cases are
- (1) show annotations in all views. This means you set an AnnotationModel with this interface, and then call setAnnotationBorderVisible() for each view.
- (2) show annotations only in one view. This means to not use this interface. Instead, use the AnnotationViewInterface, which inherits this interface. This means you set a model for the specific View.
If you set a model to the Document and the View, the View's model has higher priority.
More information about interfaces for the document can be found in Document Extension Interfaces.
- See also
- KParts::ReadWritePart, KTextEditor::Editor, KTextEditor::View, KTextEditor::MarkInterface
Definition at line 283 of file document.h.
Member Enumeration Documentation
◆ MarkChangeAction
Possible actions on a mark.
- See also
- markChanged()
Enumerator | |
---|---|
MarkAdded | action: a mark was added.
|
MarkRemoved | action: a mark was removed. |
Definition at line 1658 of file document.h.
◆ MarkTypes
Predefined mark types.
To add a new standard mark type, edit this interface and document the type.
Enumerator | |
---|---|
markType01 | Bookmark. |
markType02 | Breakpoint active. |
markType03 | Breakpoint reached. |
markType04 | Breakpoint disabled. |
markType05 | Execution mark. |
markType06 | Warning. |
markType07 | Error. |
Definition at line 1551 of file document.h.
◆ ModifiedOnDiskReason
Reasons why a document is modified on disk.
Enumerator | |
---|---|
OnDiskUnmodified | Not modified. |
OnDiskModified | The file was modified on disk. |
OnDiskCreated | The file was created on disk. |
OnDiskDeleted | The file was deleted on disk. |
Definition at line 1423 of file document.h.
Constructor & Destructor Documentation
◆ Document()
|
protected |
Constructor.
Create a new document with parent
.
Pass it the internal implementation to store a d-pointer.
- Parameters
-
impl d-pointer to use parent parent object
- See also
- Editor::createDocument()
Definition at line 12 of file document.cpp.
◆ ~Document()
|
overridedefault |
Virtual destructor.
Member Function Documentation
◆ aboutToClose
|
signal |
Warn anyone listening that the current document is about to close.
At this point all of the information is still accessible, such as the text, cursors and ranges.
Any modifications made to the document at this point will be lost.
- Parameters
-
document the document being closed
◆ aboutToDeleteMovingInterfaceContent
|
signal |
This signal is emitted before the cursors/ranges/revisions of a document are destroyed as the document is deleted.
- Parameters
-
document the document which the interface belongs to which is in the process of being deleted
◆ aboutToInvalidateMovingInterfaceContent
|
signal |
This signal is emitted before the ranges of a document are invalidated and the revisions are deleted as the document is cleared (for example on load/reload).
While this signal is emitted, the old document content is still valid and accessible before the clear.
- Parameters
-
document the document which the interface belongs to which will invalidate its data
◆ aboutToReload
|
signal |
Warn anyone listening that the current document is about to reload.
At this point all of the information is still accessible, such as the text, cursors and ranges.
Any modifications made to the document at this point will be lost.
- Parameters
-
document the document being reloaded
◆ aboutToSave
|
signal |
Emitted just before the document will be saved Any modifications made to the document at this point will get stored on disk.
- Parameters
-
document the document that was reloaded.
- Since
- 5.91
◆ addMark()
|
pure virtual |
Add marks of type markType
to line
.
Existing marks on this line are preserved. If the mark markType
already is set, nothing happens.
- Parameters
-
line line to set the mark markType mark type
- See also
- removeMark(), setMark()
◆ annotationModel()
|
pure virtual |
returns the currently set AnnotationModel or 0 if there's none set
- Returns
- the current AnnotationModel
◆ characterAt()
|
pure virtual |
◆ checksum()
|
pure virtual |
Get the git hash of the Document's contents on disk.
The returned hash equals the git hash of the file written to disk. If the document is a remote document, the checksum may not be available. In this case, QByteArray::isNull() returns true.
git hash is defined as:
sha1("blob " + filesize + "\0" + filecontent)
- Returns
- the git hash of the document
◆ clear()
|
pure virtual |
Remove the whole content of the document.
- Returns
- true on success, otherwise false
- See also
- removeText(), removeLine()
◆ clearMark()
|
pure virtual |
Clear all marks set in the line
.
- Parameters
-
line line to clear marks
- See also
- clearMarks(), removeMark(), addMark()
◆ clearMarks()
|
pure virtual |
Clear all marks in the entire document.
- See also
- clearMark(), removeMark() TODO: dominik: add argument unit mask = 0
◆ configChanged
|
signal |
This signal is emitted whenever the current document configuration is changed.
- Parameters
-
document the document which's config has changed
- Since
- 5.79
◆ configKeys()
|
pure virtual |
Get a list of all available keys.
◆ configValue()
Get a value for the key
.
◆ createView()
|
pure virtual |
Create a new view attached to parent
.
- Parameters
-
parent parent widget mainWindow the main window responsible for this view, if any
- Returns
- the new view
◆ cursorToOffset()
|
pure virtual |
Retrives the offset for the given cursor position NOTE: It will return -1 if the cursor was invalid or out of bounds.
- Since
- 6.0
◆ defaultStyleAt()
|
pure virtual |
Get the default style of the character located at position
.
If position
is not a valid text position, the default style KSyntaxHighlighting::Theme::TextStyle::Normal is returned.
- Note
- Further information about the colors of default styles depend on the currently chosen schema. Since each View may have a different color schema, the color information can be obtained through View::defaultStyleAttribute() and View::lineAttributes().
- Parameters
-
position text position
- Returns
- default style, see enum KSyntaxHighlighting::Theme::TextStyle
◆ discardDataRecovery()
|
pure virtual |
If recover data is available, calling discardDataRecovery() will discard the recover data and the recover data is lost.
If isDataRecoveryAvailable() returns false, calling this function does nothing.
- See also
- isDataRecoveryAvailable(), recoverData()
◆ documentEnd()
|
pure virtual |
End position of the document.
- Returns
- The last column on the last line of the document
- See also
- all()
◆ documentName()
|
pure virtual |
Get this document's name.
The editor part should provide some meaningful name, like some unique "Untitled XYZ" for the document - without URL or basename for documents with url.
- Returns
- readable document name
◆ documentNameChanged
|
signal |
This signal is emitted whenever the document
name changes.
- Parameters
-
document document which changed its name
- See also
- documentName()
◆ documentRange()
|
inline |
A Range which encompasses the whole document.
- Returns
- A range from the start to the end of the document
Definition at line 785 of file document.h.
◆ documentReload()
|
pure virtual |
Reload the current file.
The user will be prompted by the part on changes and more and can cancel this action if it can harm.
- Returns
- true if the reload has been done, otherwise false. If the document has no url set, it will just return false.
◆ documentSave()
|
pure virtual |
Save the current file.
The user will be asked for a filename if needed and more.
- Returns
- true on success, i.e. the save has been done, otherwise false
◆ documentSaveAs()
|
pure virtual |
Save the current file to another location.
The user will be asked for a filename and more.
- Returns
- true on success, i.e. the save has been done, otherwise false
◆ documentSavedOrUploaded
|
signal |
This signal should be emitted after a document has been saved to disk or for remote files uploaded.
saveAs should be set to true, if the operation is a save as operation
◆ documentUrlChanged
|
signal |
This signal is emitted whenever the document
URL changes.
- Parameters
-
document document which changed its URL
- See also
- KParts::ReadOnlyPart::url()
◆ editableMarks()
|
pure virtual |
Get, which marks can be toggled by the user.
The returned value is a mark mask containing all editable marks combined with a logical OR.
- Returns
- mark mask containing all editable marks
- See also
- setEditableMarks()
◆ editingFinished
|
signal |
Editing transaction has finished.
- Note
- This signal is emitted also for editing actions that maybe do not modify the
document
contents (think of having an empty EditingTransaction). If you want to get notified only after text really changed, connect to the signal textChanged().
- Parameters
-
document document which emitted this signal
- See also
- textChanged()
◆ editingStarted
|
signal |
Editing transaction has started.
- Parameters
-
document document which emitted this signal
◆ embeddedHighlightingModes()
|
pure virtual |
Get all available highlighting modes for the current document.
Each document can be highlighted using an arbitrary number of highlighting contexts. This method will return the names for each of the used modes.
Example: The "PHP (HTML)" mode includes the highlighting for PHP, HTML, CSS and JavaScript.
- Returns
- Returns a list of embedded highlighting modes for the current Document.
◆ encoding()
|
pure virtual |
Get the current chosen encoding.
The return value is an empty string, if the document uses the default encoding of the editor and no own special encoding.
- Returns
- current encoding of the document
- See also
- setEncoding()
◆ endOfLine()
|
inline |
Get the end cursor position of line line
.
- Parameters
-
line line
- See also
- lineLength(), line()
Definition at line 817 of file document.h.
◆ highlightingMode()
|
pure virtual |
Return the name of the currently used mode.
- Returns
- name of the used mode
- See also
- highlightingModes(), setHighlightingMode()
◆ highlightingModeAt()
|
pure virtual |
Get the highlight mode used at a given position in the document.
Retrieve the name of the applied highlight mode at a given position
in the current document.
Calling this might trigger re-highlighting up to the given line. Therefore this is not const.
- See also
- highlightingModes()
◆ highlightingModeChanged
|
signal |
Warn anyone listening that the current document's highlighting mode has changed.
- Parameters
-
document the document which's mode has changed
- See also
- setHighlightingMode()
◆ highlightingModes()
|
pure virtual |
Return a list of the names of all possible modes.
- Returns
- list of mode names
- See also
- highlightingMode(), setHighlightingMode()
◆ highlightingModeSection()
|
pure virtual |
Returns the name of the section for a highlight given its index in the highlight list (as returned by highlightModes()).
You can use this function to build a tree of the highlight names, organized in sections.
- Parameters
-
index the index of the highlight in the list returned by modes()
◆ insertLine()
|
pure virtual |
Insert line(s) at the given line number.
The newline character '\n' is treated as line delimiter, so it is possible to insert multiple lines. To append lines at the end of the document, use
- Parameters
-
line line where to insert the text text text which should be inserted
- Returns
- true on success, otherwise false
- See also
- insertText()
◆ insertLines()
|
pure virtual |
Insert line(s) at the given line number.
The newline character '\n' is treated as line delimiter, so it is possible to insert multiple lines. To append lines at the end of the document, use
- Parameters
-
line line where to insert the text text text which should be inserted
- Returns
- true on success, otherwise false
- See also
- insertText()
◆ insertText() [1/2]
|
pure virtual |
Insert text
at position
.
- Parameters
-
position position to insert the text text text to insert block insert this text as a visual block of text rather than a linear sequence
- Returns
- true on success, otherwise false
- See also
- setText(), removeText()
◆ insertText() [2/2]
|
pure virtual |
Insert text
at position
.
- Parameters
-
position position to insert the text text text to insert block insert this text as a visual block of text rather than a linear sequence
- Returns
- true on success, otherwise false
- See also
- setText(), removeText()
◆ isDataRecoveryAvailable()
|
pure virtual |
Returns whether a recovery is available for the current document.
- See also
- recoverData(), discardDataRecovery()
◆ isEditingTransactionRunning()
|
pure virtual |
Check whether an editing transaction is currently running.
- See also
- EditingTransaction
◆ isEmpty()
|
virtual |
Returns if the document is empty.
Definition at line 103 of file document.cpp.
◆ isLineModified()
|
pure virtual |
Check whether line
currently contains unsaved data.
If line
contains unsaved data, true is returned, otherwise false. When the user saves the file, a modified line turns into a saved line. In this case isLineModified() returns false and in its stead isLineSaved() returns true.
- Parameters
-
line line to query
- See also
- isLineSaved(), isLineTouched()
- Since
- 5.0
◆ isLineSaved()
|
pure virtual |
Check whether line
currently contains only saved text.
Saved text in this case implies that a line was touched at some point by the user and then then changes were either undone or the user saved the file.
In case line
was touched and currently contains only saved data, true is returned, otherwise false.
- Parameters
-
line line to query
- See also
- isLineModified(), isLineTouched()
- Since
- 5.0
◆ isLineTouched()
|
pure virtual |
Check whether line
was touched since the file was opened.
This equals the statement isLineModified() || isLineSaved().
- Parameters
-
line line to query
- See also
- isLineModified(), isLineSaved()
- Since
- 5.0
◆ isValidTextPosition()
|
pure virtual |
Get whether cursor
is a valid text position.
A cursor position at (line, column) is valid, if
- line >= 0 and line < lines() holds, and
- column >= 0 and column <= lineLength(column).
The text position cursor
is also invalid if it is inside a Unicode surrogate. Therefore, use this function when iterating over the characters of a line.
- Parameters
-
cursor cursor position to check for validity
- Returns
- true, if
cursor
is a valid text position, otherwisefalse
- Since
- 5.0
◆ lastSavedRevision()
|
pure virtual |
Last revision the buffer got successful saved.
- Returns
- last revision buffer got saved, -1 if none
◆ line()
|
pure virtual |
Get a single text line.
- Parameters
-
line the wanted line
- Returns
- the requested line, or "" for invalid line numbers
- See also
- text(), lineLength()
◆ lineLength()
|
pure virtual |
Get the length of a given line in characters.
- Parameters
-
line line to get length from
- Returns
- the number of characters in the line or -1 if the line was invalid
- See also
- line()
◆ lines()
|
pure virtual |
Get the count of lines of the document.
- Returns
- the current number of lines in the document
- See also
- length()
◆ lineUnwrapped
|
signal |
A line got unwrapped.
- Parameters
-
document document which emitted this signal line line where the unwrap occurred
◆ lineWrapped
|
signal |
A line got wrapped.
- Parameters
-
document document which emitted this signal position position where the wrap occurred
◆ lockRevision()
|
pure virtual |
Lock a revision, this will keep it around until released again.
But all revisions will always be cleared on buffer clear() (and therefor load())
- Parameters
-
revision revision to lock
◆ mark()
|
pure virtual |
Get all marks set on the line
.
- Parameters
-
line requested line
- Returns
- a uint representing of the marks set in
line
concatenated by logical OR
- See also
- addMark(), removeMark()
◆ markChanged
|
signal |
The document
emits this signal whenever the mark
changes.
- Parameters
-
document the document which emitted the signal mark changed mark action action, either removed or added
- See also
- marksChanged()
◆ markClicked
|
signal |
The document
emits this signal whenever the mark
is left-clicked.
- Parameters
-
document the document which emitted the signal mark mark that was right-clicked handled set this to 'true' if this event was handled externally, and kate should not do own handling of the left click.
◆ markContextMenuRequested
|
signal |
The document
emits this signal whenever the mark
is right-clicked to show a context menu.
The receiver may show an own context menu instead of the kate internal one.
- Parameters
-
document the document which emitted the signal mark mark that was right-clicked pos position where the menu should be started handled set this to 'true' if this event was handled externally, and kate should not create an own context menu.
◆ markDescription()
Get the mark's
description to text.
- Parameters
-
mark mark to set the description
- Returns
- text of the given
mark
or QString(), if the entry does not exist
- See also
- setMarkDescription(), setMarkPixmap()
◆ markIcon()
Get the mark's
icon.
- Parameters
-
markType mark type. If the icon does not exist the resulting is null (check with QIcon::isNull()).
- See also
- setMarkDescription()
◆ marks()
|
pure virtual |
Get a hash holding all marks in the document.
The hash key for a mark is its line.
- Returns
- a hash holding all marks in the document
KF6 TODO: Change Mark* to Mark. No need for pointer here.
◆ marksChanged
|
signal |
The document
emits this signal whenever a mark mask changed.
- Parameters
-
document document which emitted this signal
- See also
- markChanged()
◆ markToolTipRequested
|
signal |
The document
emits this signal whenever the mark
is hovered using the mouse, and the receiver may show a tooltip.
- Parameters
-
document the document which emitted the signal mark mark that was hovered position mouse position during the hovering handled set this to 'true' if this event was handled externally
◆ mimeType()
|
pure virtual |
Get this document's mimetype.
- Returns
- mimetype
◆ mode()
|
pure virtual |
◆ modeChanged
|
signal |
Warn anyone listening that the current document's mode has changed.
- Parameters
-
document the document whose mode has changed
- See also
- setMode()
◆ modes()
|
pure virtual |
◆ modeSection()
|
pure virtual |
◆ modifiedChanged
|
signal |
This signal is emitted whenever the document's
buffer changed from either state unmodified to modified or vice versa.
- Parameters
-
document document which changed its modified state
◆ modifiedOnDisk
|
signal |
This signal is emitted whenever the document
changed its modified-on-disk state.
- Parameters
-
document the Document object that represents the file on disk isModified if true, the file was modified rather than created or deleted reason the reason why the signal was emitted
- See also
- setModifiedOnDisk()
◆ newMovingCursor()
|
pure virtual |
Create a new moving cursor for this document.
- Parameters
-
position position of the moving cursor to create insertBehavior insertion behavior
- Returns
- new moving cursor for the document
◆ newMovingRange()
|
pure virtual |
Create a new moving range for this document.
- Parameters
-
range range of the moving range to create insertBehaviors insertion behaviors emptyBehavior behavior on becoming empty
- Returns
- new moving range for the document
◆ offsetToCursor()
|
pure virtual |
Retrives the cursor position for given offset NOTE: It will return an invalid cursor(-1, -1) if offset is invalid.
- Since
- 6.0
◆ openingError()
bool Document::openingError | ( | ) | const |
True, eg if the file for opening could not be read This doesn't have to handle the KPart job canceled cases.
- Returns
- was there some problem loading the file?
Definition at line 80 of file document.cpp.
◆ postMessage()
|
pure virtual |
Post message
to the Document and its Views.
If multiple Messages are posted, the one with the highest priority is shown first.
Usually, you can simply forget the pointer, as the Message is deleted automatically, once it is processed or the document gets closed.
If the Document does not have a View yet, the Message is queued and shown, once a View for the Document is created.
- Parameters
-
message the message to show
- Returns
- true, if
message
was posted. false, if message == 0.
◆ print()
|
pure virtual |
Print the document.
This should result in showing the print dialog.
- Returns
- true if document was printed
◆ printPreview()
|
pure virtual |
Shows the print preview dialog/.
◆ readSessionConfig()
|
pure virtual |
Read session settings from the given config
.
Known flags:
SkipUrl
=> do not save/restore the fileSkipMode
=> do not save/restore the modeSkipHighlighting
=> do not save/restore the highlightingSkipEncoding
=> do not save/restore the encoding
- Parameters
-
config read the session settings from this KConfigGroup flags additional flags
- See also
- writeSessionConfig()
◆ readWriteChanged
|
signal |
This signal is emitted whenever the readWrite state of a document changes.
- Parameters
-
document the document whose read/write property changed
◆ recoverData()
|
pure virtual |
If recover data is available, calling recoverData() will trigger the recovery of the data.
If isDataRecoveryAvailable() returns false, calling this function does nothing.
◆ reloaded
|
signal |
Emitted after the current document was reloaded.
At this point, some information might have been invalidated, like for example the editing history.
- Parameters
-
document the document that was reloaded.
- Since
- 4.6
◆ removeLine()
|
pure virtual |
Remove line
from the document.
- Parameters
-
line line to remove
- Returns
- true on success, otherwise false
- See also
- removeText(), clear()
◆ removeMark()
|
pure virtual |
Remove the mark mask of type markType
from line
.
- Parameters
-
line line to remove the mark markType mark type to be removed
- See also
- clearMark()
◆ removeText()
|
pure virtual |
Remove the text specified in range
.
- Parameters
-
range range of text to remove block set this to true to remove a text block on the basis of columns, rather than everything inside range
- Returns
- true on success, otherwise false
- See also
- setText(), insertText()
◆ replaceText() [1/2]
|
virtual |
Replace text from range
with specified text
.
- Parameters
-
range range of text to replace text text to replace with block replace text as a visual block of text rather than a linear sequence
- Returns
- true on success, otherwise false
- See also
- setText(), removeText(), insertText()
Definition at line 85 of file document.cpp.
◆ replaceText() [2/2]
|
virtual |
Replace text from range
with specified text
.
- Parameters
-
range range of text to replace text text to replace with block replace text as a visual block of text rather than a linear sequence
- Returns
- true on success, otherwise false
- See also
- setText(), removeText(), insertText()
Definition at line 94 of file document.cpp.
◆ reservedMarkersCount()
|
inlinestatic |
Get the number of predefined mark types we have so far.
- Note
- FIXME: If you change this you have to make sure katepart supports the new size!
- Returns
- number of reserved marker types
Definition at line 1540 of file document.h.
◆ revision()
|
pure virtual |
Current revision.
- Returns
- current revision
◆ searchText()
QList< KTextEditor::Range > Document::searchText | ( | KTextEditor::Range | range, |
const QString & | pattern, | ||
const SearchOptions | options = Default ) const |
Searches the given input range for a text pattern.
Searches for a text pattern within the given input range. The kind of search performed depends on the options
used. Use this function for plaintext searches as well as regular expression searches. If no match is found the first (and only) element in the vector return is the invalid range. When searching for regular expressions, the first element holds the range of the full match, the subsequent elements hold the ranges of the capturing parentheses.
- Parameters
-
range Input range to search in pattern Text pattern to search for options Combination of search flags
- Returns
- List of ranges (length >=1)
- Since
- 5.11
Definition at line 108 of file document.cpp.
◆ setAnnotationModel()
|
pure virtual |
Sets a new AnnotationModel for this document to provide annotation information for each line.
- Parameters
-
model the new AnnotationModel
◆ setConfigValue()
|
pure virtual |
Set a the key's
value to value
.
◆ setEditableMarks()
|
pure virtual |
Set the mark mask the user is allowed to toggle to markMask
.
I.e. concatenate all editable marks with a logical OR. If the user should be able to add a bookmark and set a breakpoint with the context menu in the icon pane, you have to call
- Parameters
-
markMask bitmap pattern
- See also
- editableMarks(), setMarkPixmap(), setMarkDescription()
◆ setEncoding()
|
pure virtual |
Set the encoding for this document.
This encoding will be used while loading and saving files, it will not affect the already existing content of the document, e.g. if the file has already been opened without the correct encoding, this will not fix it, you would for example need to trigger a reload for this.
- Parameters
-
encoding new encoding for the document, the name must be accepted by QStringDecoder/QStringEncoder, if an empty encoding name is given, the part should fallback to its own default encoding, e.g. the system encoding or the global user settings
- Returns
- true on success, or false, if the encoding could not be set.
- See also
- encoding()
◆ setHighlightingMode()
|
pure virtual |
Set the current mode of the document by giving its name.
- Parameters
-
name name of the mode to use for this document
- Returns
- true on success, otherwise false
◆ setMark()
|
pure virtual |
Set the line's
mark types to markType
.
If line
already contains a mark of the given type it has no effect. All other marks are deleted before the mark is set. You can achieve the same by calling
- Parameters
-
line line to set the mark markType mark type
- See also
- clearMark(), addMark(), mark()
◆ setMarkDescription()
|
pure virtual |
Set the mark's
description to text
.
- Parameters
-
mark mark to set the description text new descriptive text
- See also
- markDescription(), setMarkPixmap()
◆ setMarkIcon()
|
pure virtual |
Set the mark's
icon to icon
.
- Parameters
-
markType mark type to which the icon will be attached icon new icon
- See also
- setMarkDescription()
◆ setMode()
|
pure virtual |
Set the current mode of the document by giving its name.
- Parameters
-
name name of the mode to use for this document
- Returns
- true on success, otherwise false
- See also
- mode(), modes(), modeChanged()
◆ setModifiedOnDisk()
|
pure virtual |
Set the document's modified-on-disk state to reason
.
KTextEditor implementations should emit the signal modifiedOnDisk() along with the reason. When the document is in a clean state again the reason should be ModifiedOnDiskReason::OnDiskUnmodified.
- Parameters
-
reason the modified-on-disk reason.
- See also
- ModifiedOnDiskReason, modifiedOnDisk()
◆ setModifiedOnDiskWarning()
|
pure virtual |
Control, whether the editor should show a warning dialog whenever a file was modified on disk.
If on
is true the editor will show warning dialogs.
- Parameters
-
on controls, whether the editor should show a warning dialog for files modified on disk
◆ setText() [1/2]
|
pure virtual |
Set the given text as new document content.
- Parameters
-
text new content for the document
- Returns
- true on success, otherwise false
- See also
- text()
◆ setText() [2/2]
|
pure virtual |
Set the given text as new document content.
- Parameters
-
text new content for the document
- Returns
- true on success, otherwise false
- See also
- text()
◆ text() [1/2]
|
pure virtual |
◆ text() [2/2]
◆ textChanged
|
signal |
The document
emits this signal whenever its text changes.
- Parameters
-
document document which emitted this signal
- See also
- text(), textLine()
◆ textInserted
|
signal |
Text got inserted.
- Parameters
-
document document which emitted this signal position position where the insertion occurred text inserted text
◆ textLines()
|
pure virtual |
Get the document content within the given range
.
- Parameters
-
range the range of text to retrieve block Set this to true to receive text in a visual block, rather than everything inside range
.
- Returns
- the requested text lines, or QStringList() for invalid ranges. no end of line termination is included.
- See also
- setText()
◆ textRemoved
|
signal |
Text got removed.
- Parameters
-
document document which emitted this signal range range where the removal occurred text removed text
◆ totalCharacters()
|
pure virtual |
Get the count of characters in the document.
A TAB character counts as only one character.
- Returns
- the number of characters in the document
- See also
- lines()
◆ transformCursor() [1/2]
|
pure virtual |
Transform a cursor from one revision to an other.
- Parameters
-
line line number of the cursor to transform column column number of the cursor to transform insertBehavior behavior of this cursor on insert of text at its position fromRevision from this revision we want to transform toRevision to this revision we want to transform, default of -1 is current revision
◆ transformCursor() [2/2]
|
pure virtual |
Transform a cursor from one revision to an other.
- Parameters
-
cursor cursor to transform insertBehavior behavior of this cursor on insert of text at its position fromRevision from this revision we want to transform toRevision to this revision we want to transform, default of -1 is current revision
◆ transformRange()
|
pure virtual |
Transform a range from one revision to an other.
- Parameters
-
range range to transform insertBehaviors behavior of this range on insert of text at its position emptyBehavior behavior on becoming empty fromRevision from this revision we want to transform toRevision to this revision we want to transform, default of -1 is current revision
◆ unlockRevision()
|
pure virtual |
Release a revision.
- Parameters
-
revision revision to release
◆ viewCreated
|
signal |
This signal is emitted whenever the document
creates a new view
.
It should be called for every view to help applications / plugins to attach to the view
.
- Attention
- This signal should be emitted after the view constructor is completed, e.g. in the createView() method.
- Parameters
-
document the document for which a new view is created view the new view
- See also
- createView()
◆ views()
Returns the views pre-casted to KTextEditor::Views.
◆ wordAt()
|
pure virtual |
Get the word at the text position cursor
.
The returned word is defined by the word boundaries to the left and right starting at cursor
. The algorithm takes highlighting information into account, e.g. a dash ('-') in C++ is interpreted as word boundary, whereas e.g. CSS allows identifiers with dash ('-').
If cursor
is not a valid text position or if there is no word under the requested position cursor
, an empty string is returned.
- Parameters
-
cursor requested cursor position for the word
- Returns
- the word under the cursor or an empty string if there is no word.
- See also
- wordRangeAt(), characterAt()
◆ wordRangeAt()
|
pure virtual |
Get the text range for the word located under the text position cursor
.
The returned word is defined by the word boundaries to the left and right starting at cursor
. The algorithm takes highlighting information into account, e.g. a dash ('-') in C++ is interpreted as word boundary, whereas e.g. CSS allows identifiers with dash ('-').
If cursor
is not a valid text position or if there is no word under the requested position cursor
, an invalid text range is returned. If the text range is valid, it is always on a single line.
- Parameters
-
cursor requested cursor position for the word
- Returns
- the Range spanning the word under the cursor or an invalid range if there is no word.
◆ writeSessionConfig()
|
pure virtual |
Write session settings to the config
.
See readSessionConfig() for more details about available flags
.
- Parameters
-
config write the session settings to this KConfigGroup flags additional flags
- See also
- readSessionConfig()
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 3 2024 11:46:29 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.