Kate::TextFolding
#include <katetextfolding.h>
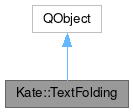
Public Types | |
enum | FoldingRangeFlag { Persistent = 0x1 , Folded = 0x2 } |
typedef QFlags< FoldingRangeFlag > | FoldingRangeFlags |
![]() | |
typedef | QObjectList |
Signals | |
void | foldingRangesChanged () |
Public Slots | |
void | clear () |
Public Member Functions | |
TextFolding (TextBuffer &buffer) | |
~TextFolding () override | |
QString | debugDump () const |
void | debugPrint (const QString &title) const |
void | editEnd (int startLine, int endLine, std::function< bool(int)> isLineFoldingStart) |
void | ensureLineIsVisible (int line) |
QJsonDocument | exportFoldingRanges () const |
KTextEditor::Range | foldingRange (qint64 id) const |
QList< QPair< qint64, FoldingRangeFlags > > | foldingRangesForParentRange (qint64 parentRangeId=-1) const |
QList< QPair< qint64, FoldingRangeFlags > > | foldingRangesStartingOnLine (int line) const |
bool | foldRange (qint64 id) |
void | importFoldingRanges (const QJsonDocument &folds) |
bool | isLineVisible (int line, qint64 *foldedRangeId=nullptr) const |
int | lineToVisibleLine (int line) const |
qint64 | newFoldingRange (KTextEditor::Range range, FoldingRangeFlags flags=FoldingRangeFlags()) |
bool | unfoldRange (qint64 id, bool remove=false) |
int | visibleLines () const |
int | visibleLineToLine (int visibleLine) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Class representing the folding information for a TextBuffer.
The interface allows to arbitrary fold given regions of a buffer as long as they are well nested. Multiple instances of this class can exist for the same buffer.
Definition at line 32 of file katetextfolding.h.
Member Typedef Documentation
◆ FoldingRangeFlags
Definition at line 62 of file katetextfolding.h.
Member Enumeration Documentation
◆ FoldingRangeFlag
Folding state of a range.
Enumerator | |
---|---|
Persistent | Range is persistent, e.g. it should not auto-delete after unfolding! |
Folded | Range is folded away. |
Definition at line 51 of file katetextfolding.h.
Constructor & Destructor Documentation
◆ TextFolding()
|
explicit |
Create folding object for given buffer.
- Parameters
-
buffer text buffer we want to provide folding info for
Definition at line 34 of file katetextfolding.cpp.
◆ ~TextFolding()
|
override |
Cleanup.
Definition at line 43 of file katetextfolding.cpp.
Member Function Documentation
◆ clear
|
slot |
Clear the complete folding.
This is automatically triggered if the buffer is cleared.
Definition at line 49 of file katetextfolding.cpp.
◆ debugDump()
QString Kate::TextFolding::debugDump | ( | ) | const |
Dump folding state as string, for unit testing and debugging.
- Returns
- current state as text
Definition at line 428 of file katetextfolding.cpp.
◆ debugPrint()
void Kate::TextFolding::debugPrint | ( | const QString & | title | ) | const |
Print state to stdout for testing.
Definition at line 434 of file katetextfolding.cpp.
◆ editEnd()
void Kate::TextFolding::editEnd | ( | int | startLine, |
int | endLine, | ||
std::function< bool(int)> | isLineFoldingStart ) |
Definition at line 440 of file katetextfolding.cpp.
◆ ensureLineIsVisible()
void Kate::TextFolding::ensureLineIsVisible | ( | int | line | ) |
Ensure that a given line will be visible.
Potentially unfold recursively all folds hiding this line, else just returns.
- Parameters
-
line line to make visible
Definition at line 241 of file katetextfolding.cpp.
◆ exportFoldingRanges()
QJsonDocument Kate::TextFolding::exportFoldingRanges | ( | ) | const |
Return the current known folding ranges a QJsonDocument to store in configs.
- Returns
- current folds as variant list
Definition at line 722 of file katetextfolding.cpp.
◆ foldingRange()
KTextEditor::Range Kate::TextFolding::foldingRange | ( | qint64 | id | ) | const |
Returns the folding range associated with id
.
If id
is not a valid id, the returned range matches KTextEditor::Range::invalid().
- Note
- This works for either persistent ranges or folded ranges. Note, that the highlighting does not add folds unless text is folded.
- Returns
- the folding range for
id
Definition at line 119 of file katetextfolding.cpp.
◆ foldingRangesChanged
|
signal |
If the folding state of existing ranges changes or ranges are added/removed, this signal is emitted.
◆ foldingRangesForParentRange()
QList< QPair< qint64, TextFolding::FoldingRangeFlags > > Kate::TextFolding::foldingRangesForParentRange | ( | qint64 | parentRangeId = -1 | ) | const |
Query child folding ranges for given range id.
To query the toplevel ranges pass id -1
- Parameters
-
parentRangeId id of parent range, pass -1 to query top level ranges
- Returns
- vector of id's + flags for child ranges
Definition at line 405 of file katetextfolding.cpp.
◆ foldingRangesStartingOnLine()
QList< QPair< qint64, TextFolding::FoldingRangeFlags > > Kate::TextFolding::foldingRangesStartingOnLine | ( | int | line | ) | const |
Queries which folding ranges start at the given line and returns the id + flags for all of them.
Very fast if nothing is folded, else binary search.
- Parameters
-
line line to query starting folding ranges
- Returns
- vector of id's + flags
Definition at line 363 of file katetextfolding.cpp.
◆ foldRange()
bool Kate::TextFolding::foldRange | ( | qint64 | id | ) |
Fold the given range.
- Parameters
-
id id of the range to fold
- Returns
- success
Definition at line 129 of file katetextfolding.cpp.
◆ importFoldingRanges()
void Kate::TextFolding::importFoldingRanges | ( | const QJsonDocument & | folds | ) |
Import the folding ranges given as a QJsonDocument like read from configs.
- Parameters
-
folds list of folds to import
Definition at line 752 of file katetextfolding.cpp.
◆ isLineVisible()
bool Kate::TextFolding::isLineVisible | ( | int | line, |
qint64 * | foldedRangeId = nullptr ) const |
Query if a given line is visible.
Very fast, if nothing is folded, else does binary search log(n) for n == number of folded ranges
- Parameters
-
line real line to query foldedRangeId if the line is not visible and that pointer is not 0, will be filled with id of range hiding the line or -1
- Returns
- is that line visible?
Definition at line 215 of file katetextfolding.cpp.
◆ lineToVisibleLine()
int Kate::TextFolding::lineToVisibleLine | ( | int | line | ) | const |
Convert a text buffer line to a visible line number.
Very fast, if nothing is folded, else walks over all folded regions O(n) for n == number of folded ranges
- Parameters
-
line line index in the text buffer
- Returns
- index in visible lines
Definition at line 281 of file katetextfolding.cpp.
◆ newFoldingRange()
qint64 Kate::TextFolding::newFoldingRange | ( | KTextEditor::Range | range, |
FoldingRangeFlags | flags = FoldingRangeFlags() ) |
Create a new folding range.
- Parameters
-
range folding range flags initial flags for the new folding range
- Returns
- on success, id of new range >= 0, else -1, we return no pointer as folding ranges might be auto-deleted internally! the ids are stable for one Kate::TextFolding, e.g. you can rely in unit tests that you get 0,1,.... for successfully created ranges!
Definition at line 76 of file katetextfolding.cpp.
◆ unfoldRange()
bool Kate::TextFolding::unfoldRange | ( | qint64 | id, |
bool | remove = false ) |
Unfold the given range.
In addition it can be forced to remove the region, even if it is persistent.
- Parameters
-
id id of the range to unfold remove should the range be removed from the folding after unfolding? ranges that are not persistent auto-remove themself on unfolding
- Returns
- success
Definition at line 148 of file katetextfolding.cpp.
◆ visibleLines()
int Kate::TextFolding::visibleLines | ( | ) | const |
Query number of visible lines.
Very fast, if nothing is folded, else walks over all folded regions O(n) for n == number of folded ranges
Definition at line 261 of file katetextfolding.cpp.
◆ visibleLineToLine()
int Kate::TextFolding::visibleLineToLine | ( | int | visibleLine | ) | const |
Convert a visible line number to a line number in the text buffer.
Very fast, if nothing is folded, else walks over all folded regions O(n) for n == number of folded ranges
- Parameters
-
visibleLine visible line index
- Returns
- index in text buffer lines
Definition at line 322 of file katetextfolding.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:11:28 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.