KGameIO
#include <KGame/KGameIO>
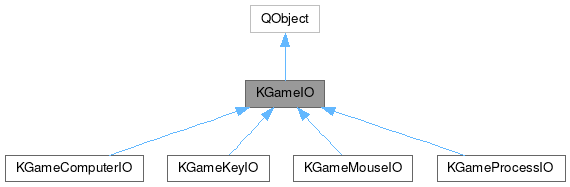
Public Types | |
enum | IOMode { GenericIO = 1 , KeyIO = 2 , MouseIO = 4 , ProcessIO = 8 , ComputerIO = 16 } |
Signals | |
void | signalPrepareTurn (QDataStream &stream, bool turn, KGameIO *io, bool *send) |
Public Member Functions | |
KGameIO () | |
KGameIO (KPlayer *) | |
void | Debug () |
KGame * | game () const |
virtual void | initIO (KPlayer *p) |
virtual void | notifyTurn (bool b) |
KPlayer * | player () const |
virtual int | rtti () const =0 |
bool | sendInput (QDataStream &stream, bool transmit=true, quint32 sender=0) |
void | setPlayer (KPlayer *p) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
KGameIO (KGameIOPrivate &dd, KPlayer *player=nullptr) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
Base class for IO devices for games.
This is the master class for creating IO game devices. You cannot use it directly. Either take one of the classes derived from it or you have to create your own IO class derived from it (more probably).
The idea behind this class is to provide a common interface for input devices into your game. By programming a KGameIO device you need not distinguish the actual IO in the game anymore. All work is done by the IO's. This allows very easy reuse in other games as well. A further advantage of using the IO's is that you can exchange the control of a player at runtime. E.g. you switch a player to be controlled by the computer or vice versa.
To achieve this you have to make all of your player inputs through a KGameIO. You will usually call KGameIO::sendInput to do so.
Member Enumeration Documentation
◆ IOMode
enum KGameIO::IOMode |
Constructor & Destructor Documentation
◆ KGameIO() [1/3]
KGameIO::KGameIO | ( | ) |
Constructs a KGameIO object.
Definition at line 39 of file kgameio.cpp.
◆ KGameIO() [2/3]
|
explicit |
Definition at line 44 of file kgameio.cpp.
◆ ~KGameIO()
|
override |
Definition at line 58 of file kgameio.cpp.
◆ KGameIO() [3/3]
|
explicitprotected |
Definition at line 49 of file kgameio.cpp.
Member Function Documentation
◆ Debug()
void KGameIO::Debug | ( | ) |
Gives debug output of the game status.
Definition at line 116 of file kgameio.cpp.
◆ game()
KGame * KGameIO::game | ( | ) | const |
Equivalent to player()->game()
- Returns
- the KGame object of this player
Definition at line 100 of file kgameio.cpp.
◆ initIO()
|
virtual |
Init this device by setting the player and e.g.
sending an init message to the device. This initialisation message is very useful for computer players as you can transmit the game status to them and only update this status in the setTurn commands.
Called by KPlayer::addGameIO only!
Reimplemented in KGameProcessIO.
Definition at line 77 of file kgameio.cpp.
◆ notifyTurn()
|
virtual |
Notifies the IO device that the player's setTurn had been called Called by KPlayer.
This emits signalPrepareTurn and sends the turn if the send parameter is set to true.
- Parameters
-
b turn is true/false
Reimplemented in KGameProcessIO.
Definition at line 82 of file kgameio.cpp.
◆ player()
KPlayer * KGameIO::player | ( | ) | const |
This function returns the player who owns this IO.
- Returns
- the player this IO device is plugged into
Definition at line 67 of file kgameio.cpp.
◆ rtti()
|
pure virtual |
Run time identification.
Predefined values are from IOMode You MUST overwrite this in derived classes!
- Returns
- rtti value
Implemented in KGameComputerIO, KGameKeyIO, KGameMouseIO, and KGameProcessIO.
◆ sendInput()
bool KGameIO::sendInput | ( | QDataStream & | stream, |
bool | transmit = true, | ||
quint32 | sender = 0 ) |
Send an input message using KPlayer::forwardInput.
Definition at line 108 of file kgameio.cpp.
◆ setPlayer()
void KGameIO::setPlayer | ( | KPlayer * | p | ) |
Sets the player to which this IO belongs to.
This is done automatically when adding a device to a player
- Parameters
-
p the player
Definition at line 72 of file kgameio.cpp.
◆ signalPrepareTurn
|
signal |
Signal generated when KPlayer::myTurn changes.
This can either be when you get the turn status or when you lose it.
The datastream has to be filled with a move. If you set (or leave) the send parameter to FALSE then nothing happens: the datastream will be ignored. If you set it to TRUE sendInput is used to send the move.
Often you want to ignore this signal (leave send=FALSE) and send the message later. This is usually the case for a human player as he probably doesn't react immediately. But you can still use this e.g. to notify the player about the turn change.
Example:
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:53:12 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.